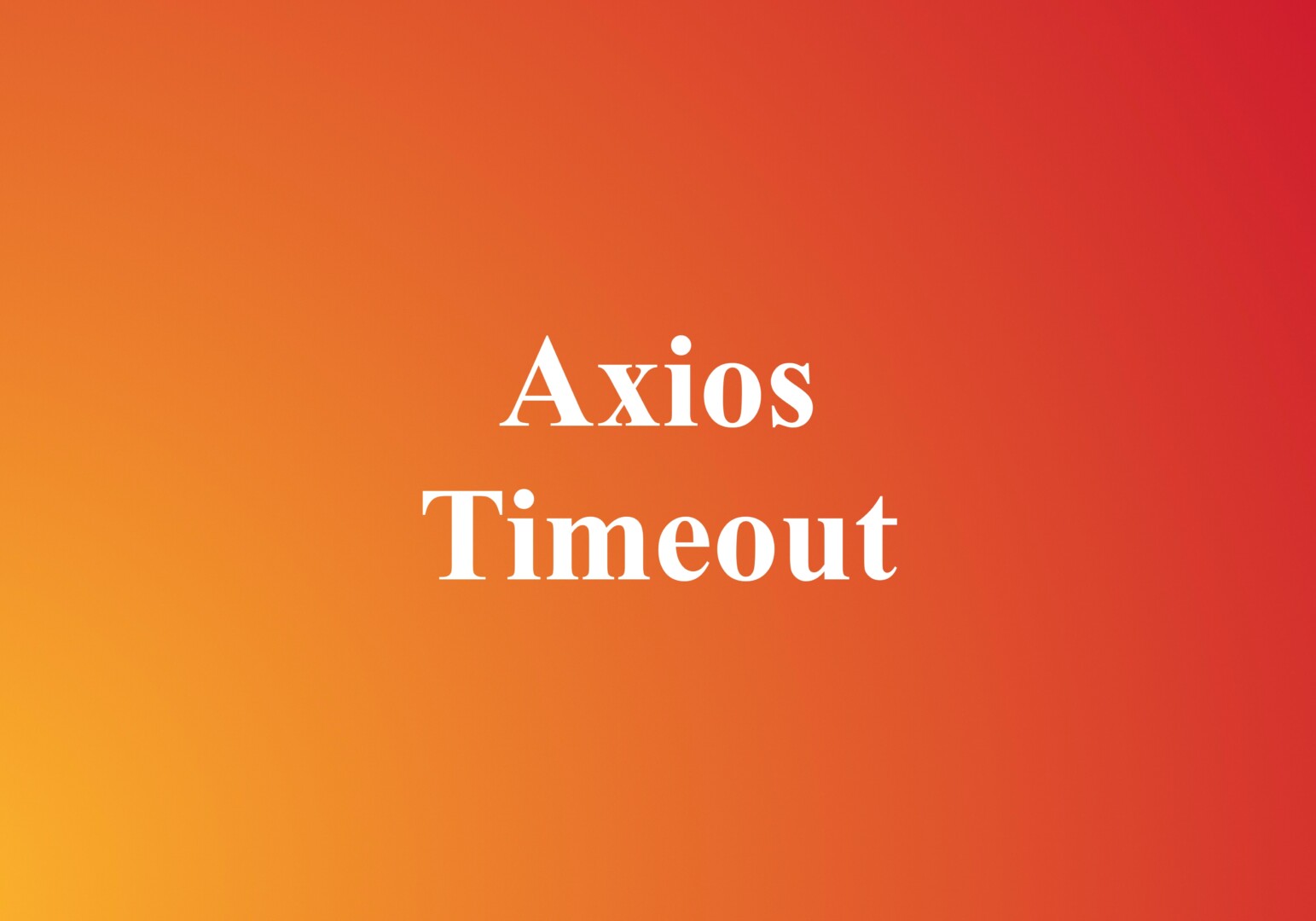
In Axios, the default timeout is 0. This quick and straightforward article shows you a few ways to set custom timeouts when making HTTP requests with Axios.
Set Timeout for a single Request
You can set a timeout for an individual request like so:
try {
const response = await axios('https://www.kindacode.com', {
timeout: 5000, // Override the default timeout to 5 seconds
method: 'GET',
});
console.log(response);
} catch (err) {
console.log(err);
}
Or like this:
try {
const response = await axios.post(
'https://jsonplaceholder.typicode.com/posts',
{
title: 'foo',
body: 'bar',
},
{
timeout: 5000, // Override the default timeout for this request
headers: {
'Content-Type': 'application/json',
},
}
);
console.log(response.data);
} catch (error) {
console.log(error);
}
Set a Timeout for multiple Requests
You can set a timeout for multiple requests by initializing an Axios instance. Here is an example:
// Create an axios instance
// and set the timeout for this instance
const client = axios.create({
baseURL: 'https://jsonplaceholder.typicode.com',
timeout: 3000, // 3 seconds
});
// make a GET request
try {
const response = await client.get('/posts');
console.log(response.data);
} catch (error) {
console.log(error);
}
// make a POST request
try {
const response = await client.post('/posts', {
title: 'foo',
body: 'bar',
userId: 1,
});
console.log(response.data);
} catch (error) {
console.log(error);
}
Further reading:
- React: How to Upload Multiple Files with Axios
- Axios: Set Headers in GET/POST Requests
- Axios: Passing Query Parameters in GET/POST Requests
- 7 Best Open-Source HTTP Request Libraries for Node.js
- 5 Best Open-Source HTTP Request Libraries for React
- How to fetch data from APIs with Axios and Hooks in React
You can also check out our Javascript category page, TypeScript category page, Node.js category page, and React category page for the latest tutorials and examples.