This article walks you through a list of the best open-source HTTP request libraries for Node.js in the year 2023. Without any further ado (like talking about the history of Node or explaining what HTTP is), let’s dive right into the things that matter.
node-fetch
- GitHub stars: 8.7k+
- NPM weekly downloads: 50m – 60m
- License: MIT
- Written in: Javascript, TypeScript
- Links: GitHub repo | NPM page
Node-fetch is one of the most downloaded npm packages these days. It’s lightweight with an unpacked size of around 75 kB.
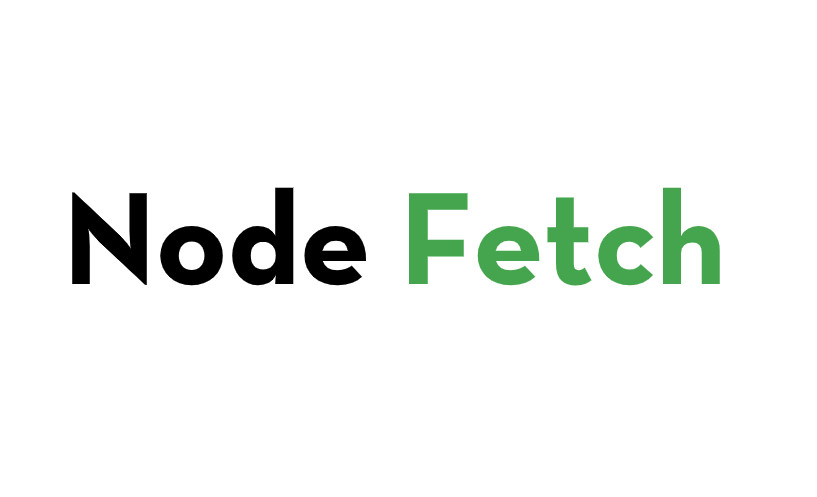
Key features:
- Stay consistent with the window.fetch API on browsers
- Use native Node streams for the body, on both request and response
- Use native promise and async functions
- Decode content encoding (gzip/deflate/brotli) properly, and convert string output to UTF-8 text or JSON automatically
- seful extensions such as redirect limit, response size limit, explicit errors for troubleshooting
Installing:
npm i node-fetch
Example:
const fetch = require('node-fetch');
const body = {name: "John Doe"};
const response = await fetch('https://api.kindacode.com/examples/post', {
method: 'post',
body: JSON.stringify(body),
headers: {'Content-Type': 'application/json'}
});
const data = await response.json();
console.log(data);
Axios
- GitHub stars: 105k+
- NPM weekly downloads: 48m – 60m
- License: MIT
- Written in: Javascript, TypeScript, HTML
- Links: GitHub repo | NPM page | Official website
Axios works for both Node.js and web browsers.
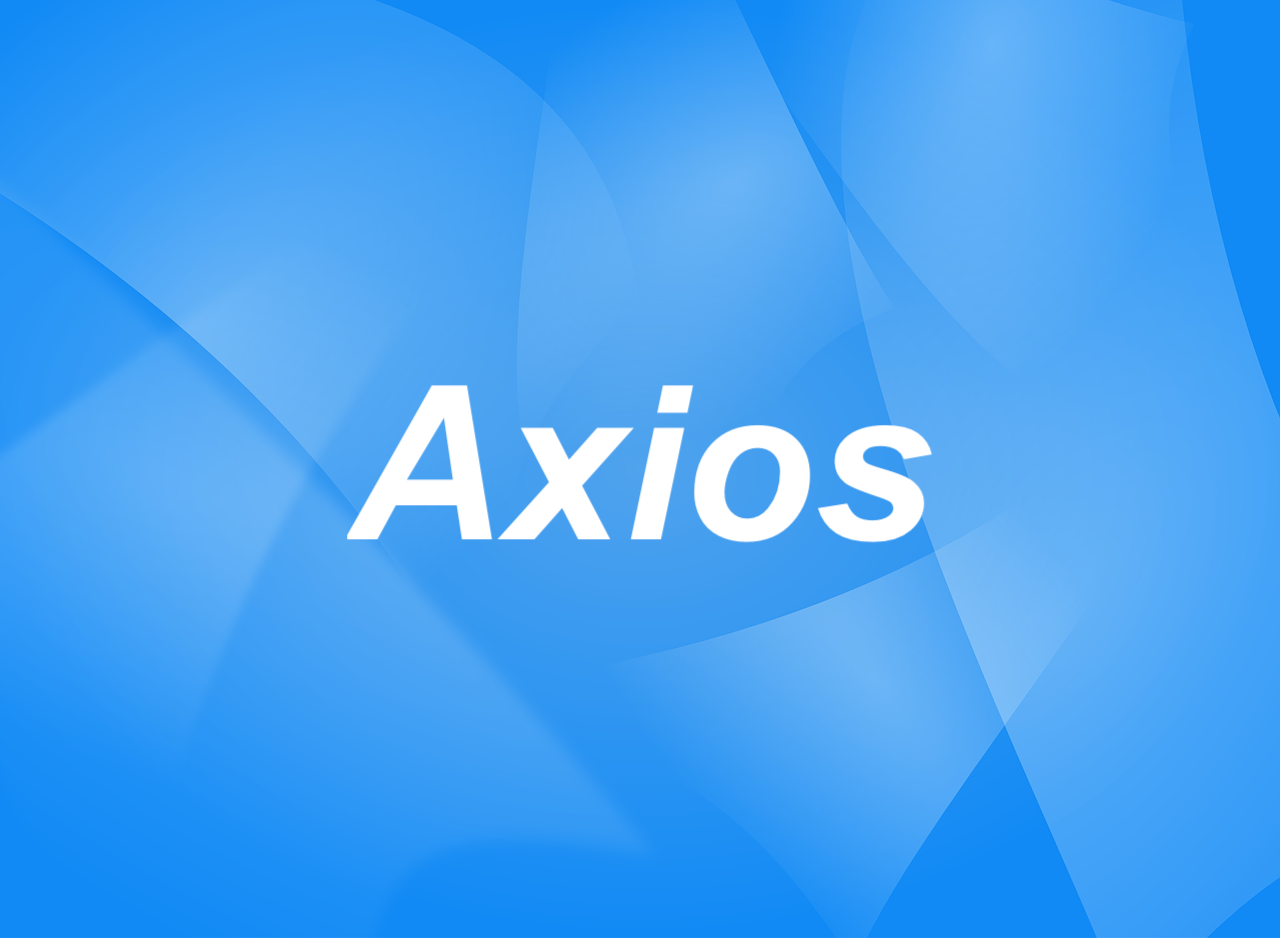
Key features:
- Make http requests from Node
- Supports the Promise API
- Transform request and response data
- Intercept request and response
- Cancelable requests
- Automatic transforms for JSON data
Installing:
npm i axios
Example:
const axios = require('axios');
axios.post('[your API endpoint]', {
name: 'A book',
price: 1.23
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
got
- GitHub stars: 13.7k+
- NPM weekly downloads: 19m – 25m
- License: MIT
- Written in: TypeScript (100%)
- Links: GitHub repo | NPM page
Got is a modern, friendly, and powerful HTTP request library for Node.js. It supports a wide range of features on the server side, such as promise API, HTTP/2, stream API, requests cancelation, RFC-compliant caching, cookie, following redirects, retrying on failure, progress events, JSON mode, self-included TypeScript support, and many more.
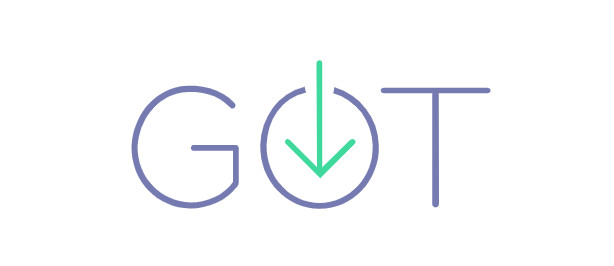
Installing:
npm i got
Example:
const got = require('got');
(async () => {
const {body} = await got.post('https://www.example.com/api/', {
json: {
title: 'Something meaningless'
},
responseType: 'json'
});
console.log(body.data);
})();
make-fetch-happen
- GitHub stars: 180+
- NPM weekly downloads: 17m – 20m
- License: ISC
- Written in: Javascript (100%)
- Links: GitHub repo | NPM page
make-fetch-happen is a lightweight Node.js library that implements window.fetch on the server-side with many additional features, including HTTP Cache support, request pooling, proxies (http, https, socks, socks4, socks5), retries, cache-fallback automatic “offline mode”, transparent gzip and deflate support, and more.
To install the package, run:
npm i make-fetch-happen
In order to use make-fetch-happen with TypeScript, you need to install @type/make-fetch-happen:
npm i @types/make-fetch-happen
Example:
const fetch = require('make-fetch-happen').defaults({
cachePath: './your-local-cache'
})
fetch('https://www.example.com/api') // will always use the cache
needle
- GitHub stars: 1.6k+
- NPM weekly downloads: 7m – 9m
- License: MIT
- Written in: Javascript
- Links: GitHub repo | NPM page
Needle is a lean and handsome HTTP request library for Node with only two real dependencies.
Key features:
- All of Node’s native TLS options, such as ‘rejectUnauthorized’
- Basic & Digest authentication with auto-detection
- HTTP Proxy forwarding, optionally with authentication
- Streaming gzip, deflate, and brotli decompression
- Automatic XML & JSON parsing
- 301/302/303 redirect following, with fine-grained tuning, and
- Streaming non-UTF-8 charset decoding, via iconv-lite
Installing:
npm i needle
If you want to write TypeScript:
npm i @types/needle
Example:
const needle = require('needle');
needle('get', 'https://www.kindacode.com')
.then(function(resp) { console.log(resp.body) })
.catch(function(err) { console.error(err) })
superagent
- GitHub stars: 16.5k+
- NPM weekly downloads: 8m – 12m
- License: MIT
- Written in: Javascript, HTML, Makefile
- Links: GitHub repo | NPM page
superagent is a small progressive HTTP request library for both client-side and Node.js. It’s extendable with many plugins and extensions, such as:
- superagent-no-cache: Prevents caching by including Cache-Control header
- superagent-prefix: prefixes absolute URLs (useful in test environment)
- superagent-mock: simulate HTTP calls by returning data fixtures based on the requested URL
- superagent-mocker: simulate REST API
- superagent-cache-plugin: A SuperAgent plugin with built-in, flexible caching
- superagent-node-http-timings: Measures http timings in node.js
- The list of extensions
Installing:
npm i superagent
Types definition:
npm i @types/superagent
cross-fetch
- GitHub stars: 1.5k+
- NPM weekly downloads: 15m – 20m
- License: MIT
- Written in: Javascript, Typescript, HTML, Shell
- Links: GitHub repo | NPM page
This one is a universal WHATWG Fetch API for Node, Browsers, and React Native. The scenario that cross-fetch really shines is when the same JavaScript codebase needs to run on different platforms. The library also supports TypeScript out-of-the-box.
Installing:
npm i cross-fetch
Example:
const fetch = require('cross-fetch');
(async () => {
try {
const res = await fetch('https://www.kindacode.com');
if (res.status >= 400) {
throw new Error("Bad response from server");
}
const data = await res.json();
console.log(data);
} catch (err) {
console.error(err);
}
})();
Wrap Up
We’ve covered the best open-source HTTP request libraries for Node.js nowadays. Which one will you choose for your next project? If you’d like to explore more new and awesome things about Node.js and back-end development, take a look at the following articles:
- 6 best Node.js frameworks to build backend APIs
- Top 4 best Node.js Open Source Headless CMS
- Using Axios to download images and videos in Node.js
- Node.js: How to Use “Import” and “Require” in the Same File
- 4 Ways to Convert Object into Query String in Node.js
You can also check out our Node.js category page for the latest tutorials and examples.