You can check whether your Flutter app is running on a web browser by using the kIsWeb constant from the foundation library.
Example
Screenshot:
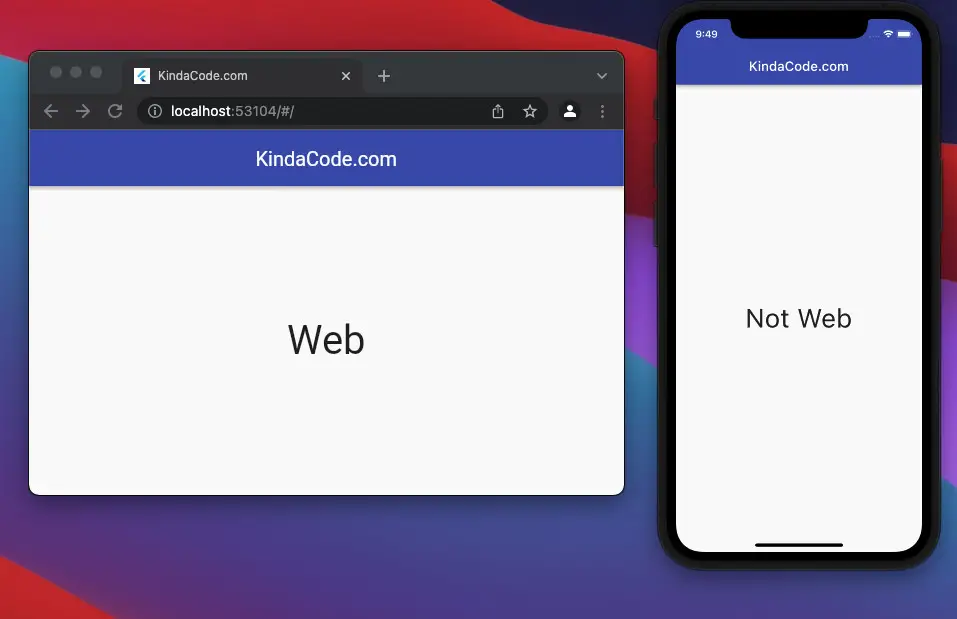
The code:
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/foundation.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const HomePage());
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('KindaCode.com')),
body: const Center(
child: Text(kIsWeb ? 'Web' : 'Not Web',
style: TextStyle(
fontSize: 40,
)),
),
);
}
}
Using this technique, you can implement separate logic and features for your Flutter application on the web and on other platforms.
Further reading:
- How to implement a Date Range Picker in Flutter
- Flutter: Making a Tic Tac Toe Game from Scratch
- Flutter: Detect the platform your app is running on
- Flutter: Get the device OS version
- Flutter Web: 2 Ways to Change Page Title Dynamically
- Create a Custom NumPad (Number Keyboard) in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.