This is a short and straight-to-the-point guide on how to make a search filed with a magnifying glass icon inside from scratch without using any third-party library. Here’s what you’ll see at the end:
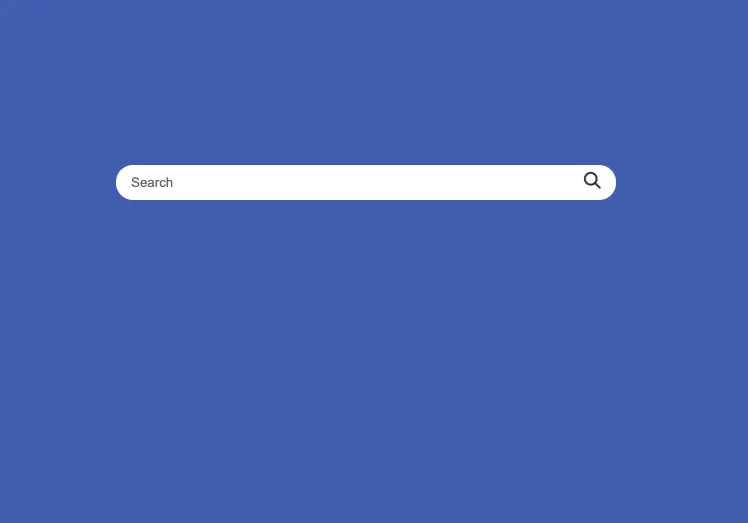
Getting Started
1. Create a new folder called example or whatever you like (the name doesn’t matter). In this folder, create 2 files: index.html and style.css.
2. Download the following PNG image to the example folder (you can use another png file if you want):
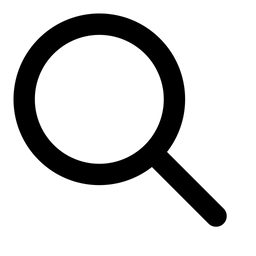
Our folder structure at this point:
.
├── index.html
├── search.png
└── style.css
3. HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Search Field</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div class="container">
<form action="/" method="GET" class="form">
<input type="search" placeholder="Search" class="search-field" />
<button type="submit" class="search-button">
<img src="search.png">
</button>
</form>
</div>
</body>
</html>
4. CSS code:
* {
/* Reset browser's padding and margin */
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
background: #4a69bd;
}
.container {
margin: 200px auto;
width: 500px;
height: 60px
}
/* The point of this tutorial is here */
.form {
display: flex;
flex-direction: row;
}
.search-field {
width: 100%;
padding: 10px 35px 10px 15px;
border: none;
border-radius: 100px;
outline: none;
}
.search-button {
background: transparent;
border: none;
outline: none;
margin-left: -33px;
}
.search-button img {
width: 20px;
height: 20px;
object-fit: cover;
}
That’s it. Further reading:
- CSS: Flipping Card on Hover
- Most Popular Open-Souce CSS Frameworks
- CSS Flexbox: Make an Element Fill the Remaining Space
- CSS Grid: repeat() and auto-fill example
- CSS: Styling Scrollbar Example
You can also check out our CSS category page for the latest tutorials and examples.