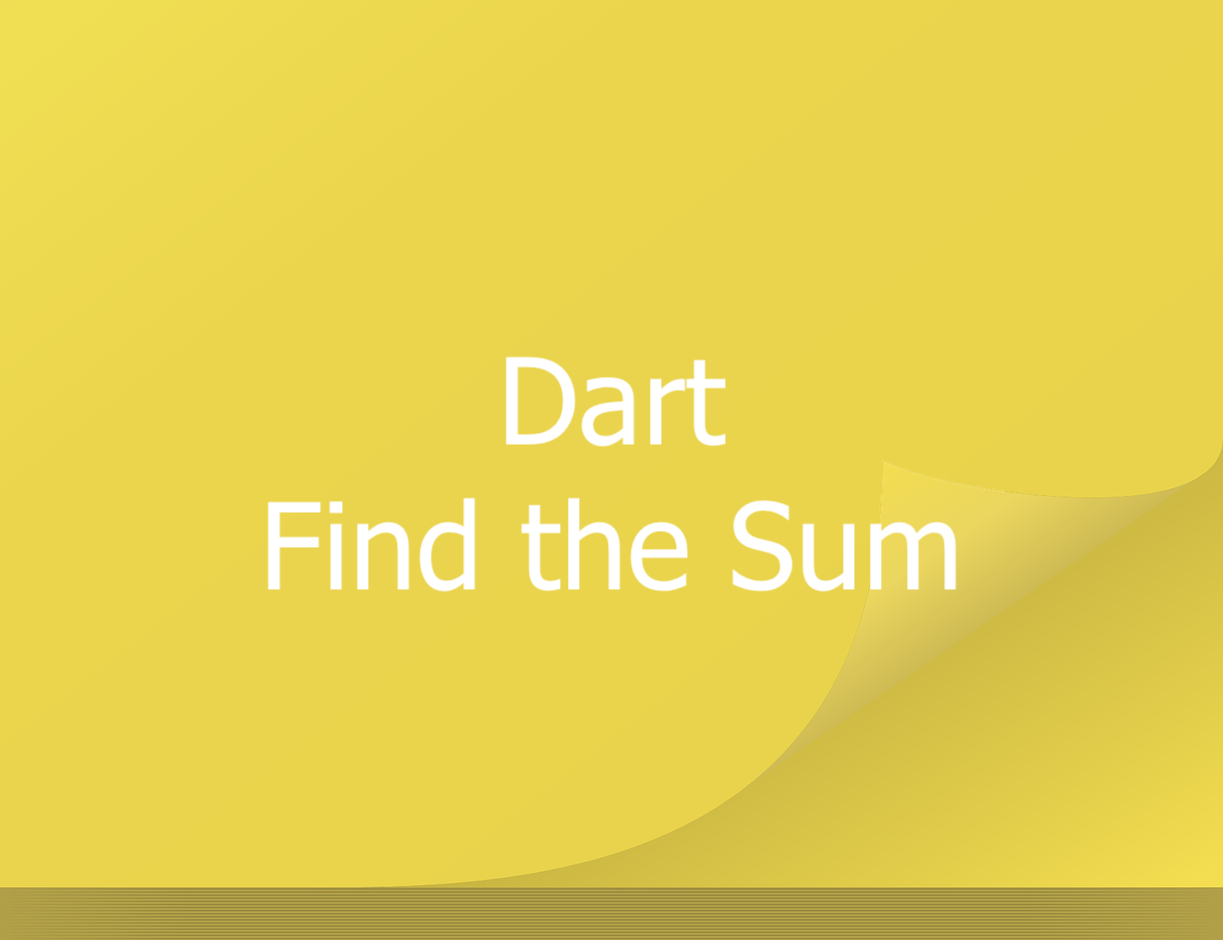
This short example-based article shows you a few ways to find the sum of all values in a given map in Dart (presume that all the values are numbers).
Using a For/In loop
Example:
// main.dart
void main() {
const Map<String, double> prices = {
'priceOne': 9.9,
'priceTwo': 20.5,
'priceThree': 45,
'priceFour': 30.88
};
double sum = 0;
// get the list of all values of the map
List<double> values = prices.values.toList();
// calculate the sum with a loop
for (double value in values) {
sum += value;
}
// print out the sum
print(sum);
}
Output:
106.28
Using Reduce() function
Example:
// main.dart
void main() {
const Map<String, double> map = {
'value1': 9,
'value2': 9.9,
'value3': 14,
'value4': 15.5,
'value5': 20
};
Iterable<double> values = map.values;
final result = values.reduce((sum, value) => sum + value);
print(result);
}
Output:
68.4
That’s it. Further reading:
- Dart: Converting a List to a Set and vice versa
- Flutter & Dart: How to Check if a String is Null/Empty
- How to Get Device ID in Flutter (2 approaches)
- Dart: Get Strings from ASCII Codes and Vice Versa
- Flutter: Making a Tic Tac Toe Game from Scratch
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.