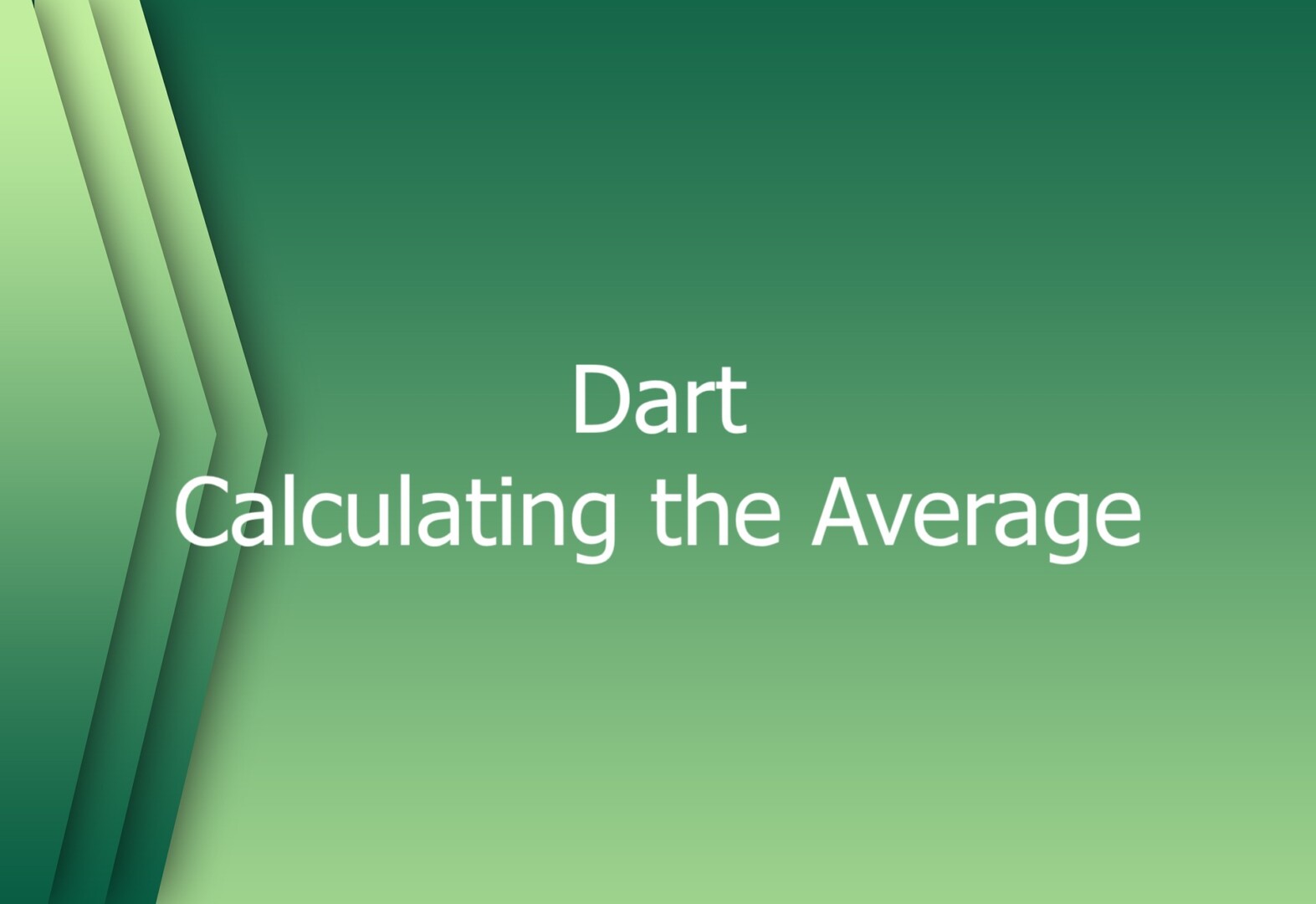
This practical, succinct article walks you through two examples of calculating the average of numerical data in a given list. The first example works with a simple list that contains only numbers, while the second one deals with a list of maps. No more ado; let’s get started.
List of Numbers
To find the result, just use the average getter, like so:
// kindacode.com
import 'package:collection/collection.dart';
void main() {
final listOne = [1, 2, 3, 4, 5, 9, 10];
final averageOne = listOne.average;
print(averageOne);
final listTwo = [-3, 1.4, 3.4, 9, 10, 6];
final averageTwo = listTwo.average;
print(averageTwo);
}
Output:
4.857142857142857
4.466666666666667
You don’t even need to calculate the sum of all elements and then divide it by the number of elements. If you want to display the results in a nicer format, see this article: Show a number to two decimal places in Dart (Flutter).
List of Maps
Let’s imagine you have a list of maps where each map contains information about a product, including product name, price, etc. The goal is to find the average price of all products. The program below demonstrates how to solve the problem:
// kindacode.com
import 'package:collection/collection.dart';
void main() {
final products = [
{"name": "Product One", "price": 3.33},
{"name": "Product Two", "price": 9.99},
{"name": "Product Three", "price": 5.00}
];
// Create of list of prices
final prices = products.map((product) => product["price"] as double).toList();
// Use the average function to get the average price
final averagePrice = prices.average;
print("Average Price: $averagePrice");
}
Output:
6.1066666666666665
That’s if. Further reading:
- Dart: Sorting Entries of a Map by Its Values
- How to Reverse/Shuffle a List in Dart
- Flutter & Dart: Convert a String into a List and vice versa
- Flutter: 6 Best Packages to Create Cool Bottom App Bars
- Adding a Border to an Image in Flutter (3 Examples)
- Flutter: How to Create a Custom Icon Picker from Scratch
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.