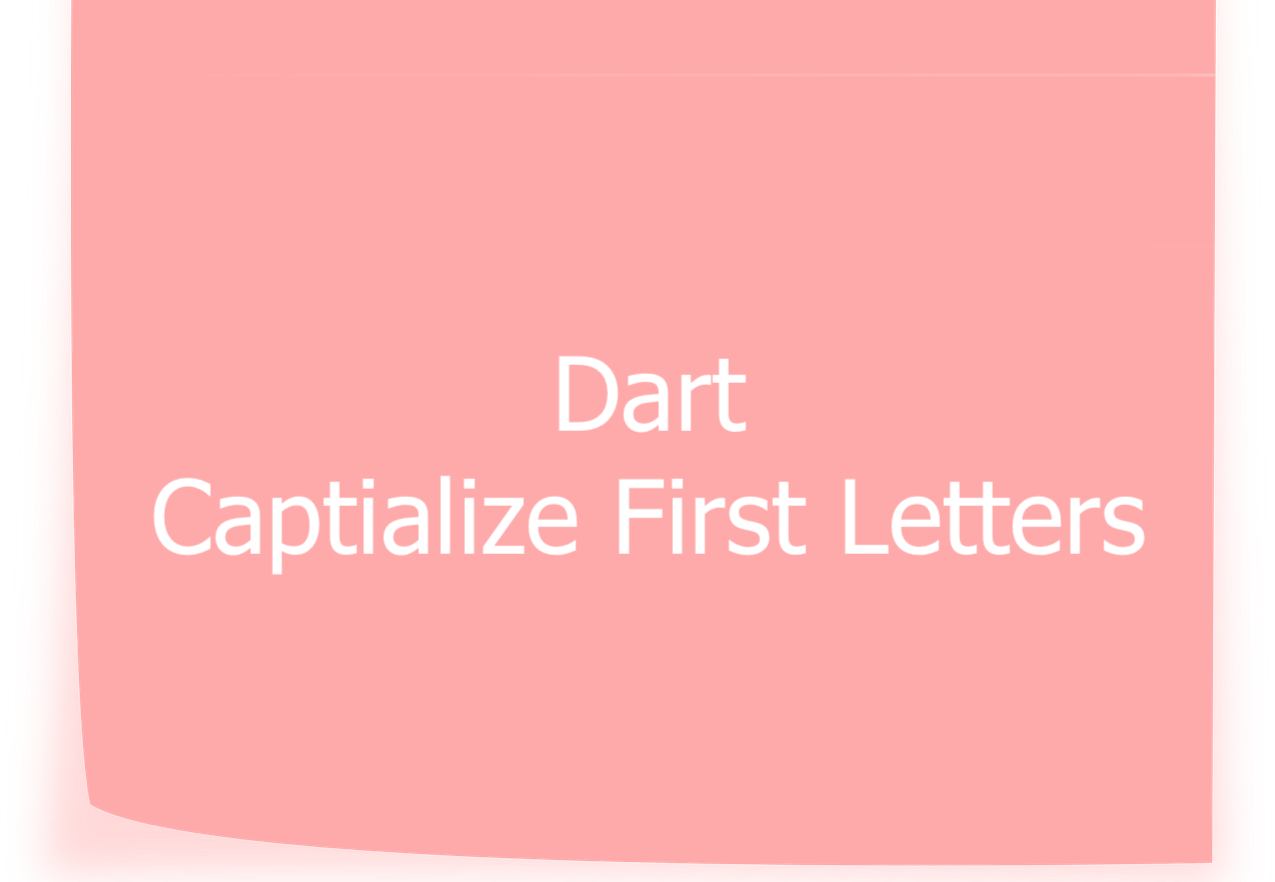
The example below shows you how to capitalize the first letter of each word in a string (use a title case for each word in a string) in Dart (and Flutter as well).
The code:
// KindaCode.com
void main() {
String totTitle(String input) {
final List<String> splitStr = input.split(' ');
for (int i = 0; i < splitStr.length; i++) {
splitStr[i] =
'${splitStr[i][0].toUpperCase()}${splitStr[i].substring(1)}';
}
final output = splitStr.join(' ');
return output;
}
const str1 = 'Kindacode.com is a website about programming.';
const str2 = 'Today is a raining day!';
print(totTitle(str1));
print(totTitle(str2));
}
Output:
Kindacode.com Is A Website About Programming.
Today Is A Raining Day!
That’s it. Further reading:
- Flutter Vertical Text Direction: An Example
- Dart: Sorting Entries of a Map by Its Values
- Dart: Convert Map to Query String and vice versa
- Conditional (Ternary) Operator in Dart and Flutter
- Sorting Lists in Dart and Flutter (5 Examples)
- Write a simple BMI Calculator with Flutter (Null Safety)
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.