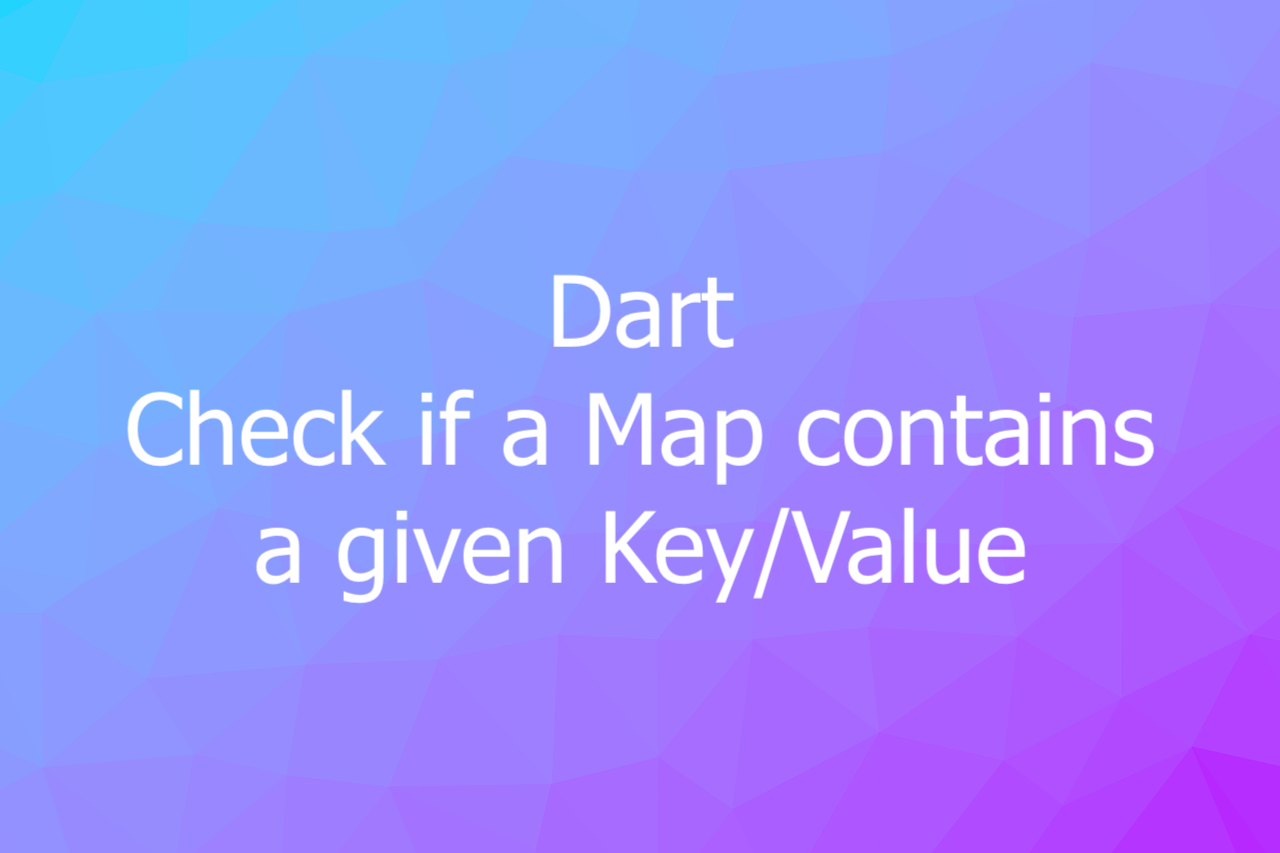
Dart provides us the tools to quickly and conveniently check whether a map contains a given key or value. These tools are the containsKey() method and containsValue() method.
Example:
// kindacode.com
// create a sample map
Map<String, dynamic> item = {
'id': 123,
'name': 'A Useless Pink Phone',
'OS': 'Android',
'manufacturer': 'KindaCode.com',
'price': 0.1,
'freeship': true
};
void main() {
if (item.containsKey('weight')) {
print('The weight of the product is ${item['weight']}');
} else {
print('No weight provided');
}
if (item.containsValue('Kindacode.com')) {
print('The product is bad. You should not buy it');
} else {
print('The product is not from Kindacode.com');
}
}
Output:
No weight provided
The product is not from Kindacode.com
Further reading:
- Dart: How to Add new Key/Value Pairs to a Map
- Dart: How to Update a Map
- Dart: How to remove specific Entries from a Map
- Dart: Convert Timestamp to DateTime and vice versa
- Dart: Convert Map to Query String and vice versa
- How to check numeric strings in Flutter and Dart
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.