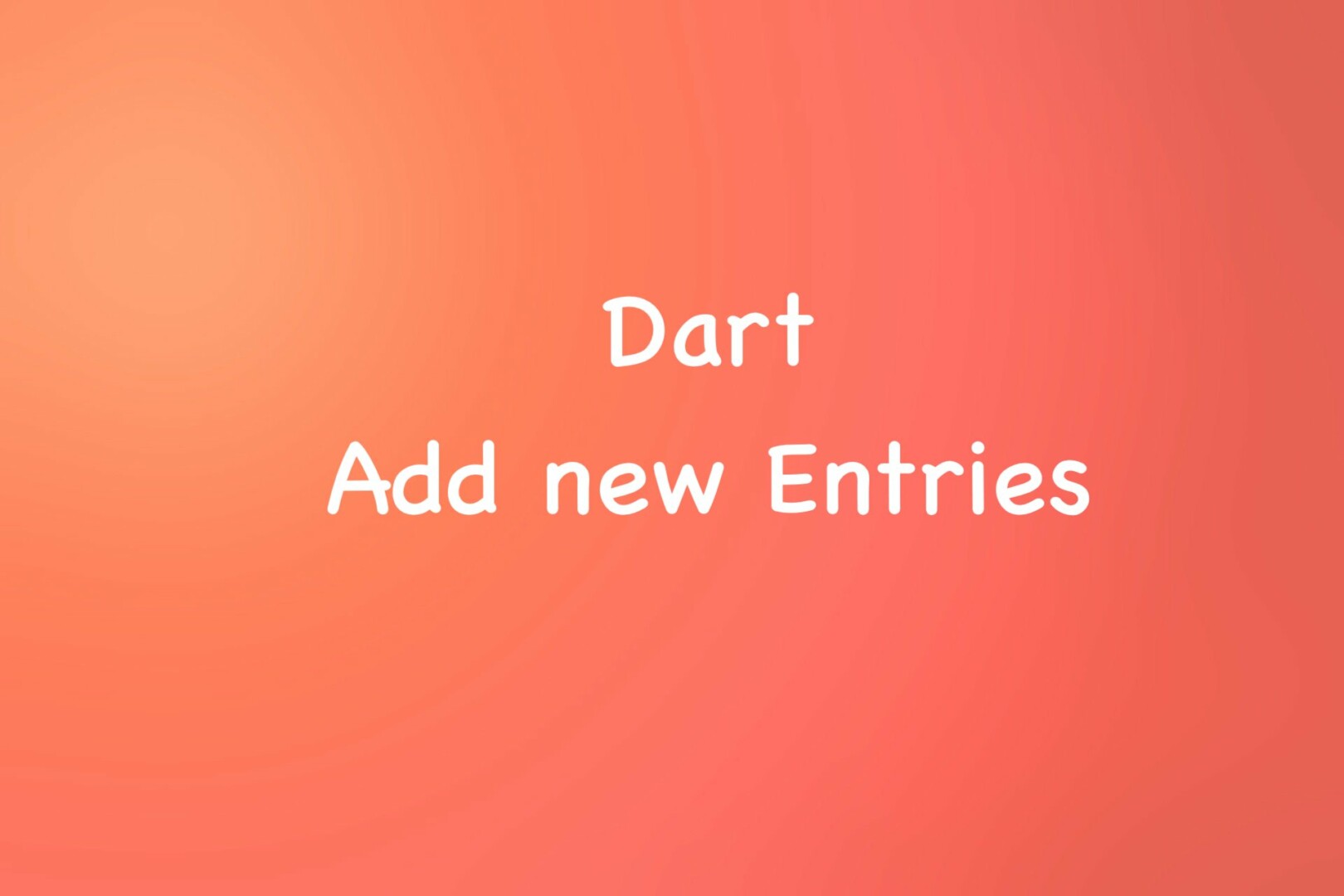
This concise, practical article shows you a few ways to add new key/value pairs to a given map in Dart (and Flutter as well).
Using map[newKey] = newValue syntax
Example:
Map<String, dynamic> map = {
'key1': 'Dog',
'key2': 'Chicken',
'key3': 'Kindacode.com',
'key4': 'Buffalo'
};
void main() {
map['key5'] = 'Blue';
map['key6'] = 'Green';
map['some_key'] = 'Hey Bro';
print(map);
}
Output:
{
key1: Dog,
key2: Chicken,
key3: Kindacode.com,
key4: Buffalo,
key5: Blue,
key6: Green,
some_key: Hey Bro
}
Using addAll() method
This method can be used to combine 2 different maps. It will add all key/value pairs of a map to another map. If a key already exists, its value will be overwritten.
Example:
// kindacode.com
Map<String, dynamic> map1 = {'key1': 'A', 'key2': 'B', 'key3': 'C'};
Map<String, dynamic> map2 = {'key3': '3', 'key4': '4', 'key5': '5'};
void main() {
map1.addAll(map2);
print(map1);
}
Output:
{
key1: A,
key2: B,
key3: 3, // This value is overwritten
key4: 4,
key5: 5
}
Using addEntries() method
This method is used to add a collection of key/value pairs to a given map. Like the addAll() method, if a key already exists in the map, its value will be overwritten.
Example:
// kindacode.com
Map<String, dynamic> map = {'key1': 'A', 'key2': 'B', 'key3': 'C'};
void main() {
map.addEntries([
const MapEntry('key3', 'A new value'),
const MapEntry('key4', 'D'),
const MapEntry('key5', 'E'),
const MapEntry('key6', 'Kindacode.com')
]);
print(map);
}
Output:
{
key1: A,
key2: B,
key3: A new value,
key4: D,
key5: E,
key6: Kindacode.com
}
Conclusion
We’ve gone through a couple of different ways to add new entries to a given map in Dart. These techniques might be very useful for you when working with data-intensive apps. If you’d like to learn more about modern Dart and Flutter, take a look at the following articles:
- Using Cascade Notation in Dart and Flutter
- Dart: Sorting Entries of a Map by Its Values
- Dart: How to Update a Map
- Dart: How to remove specific Entries from a Map
- Flutter & SQLite: CRUD Example
- Flutter and Firestore Database: CRUD example (null safety)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.