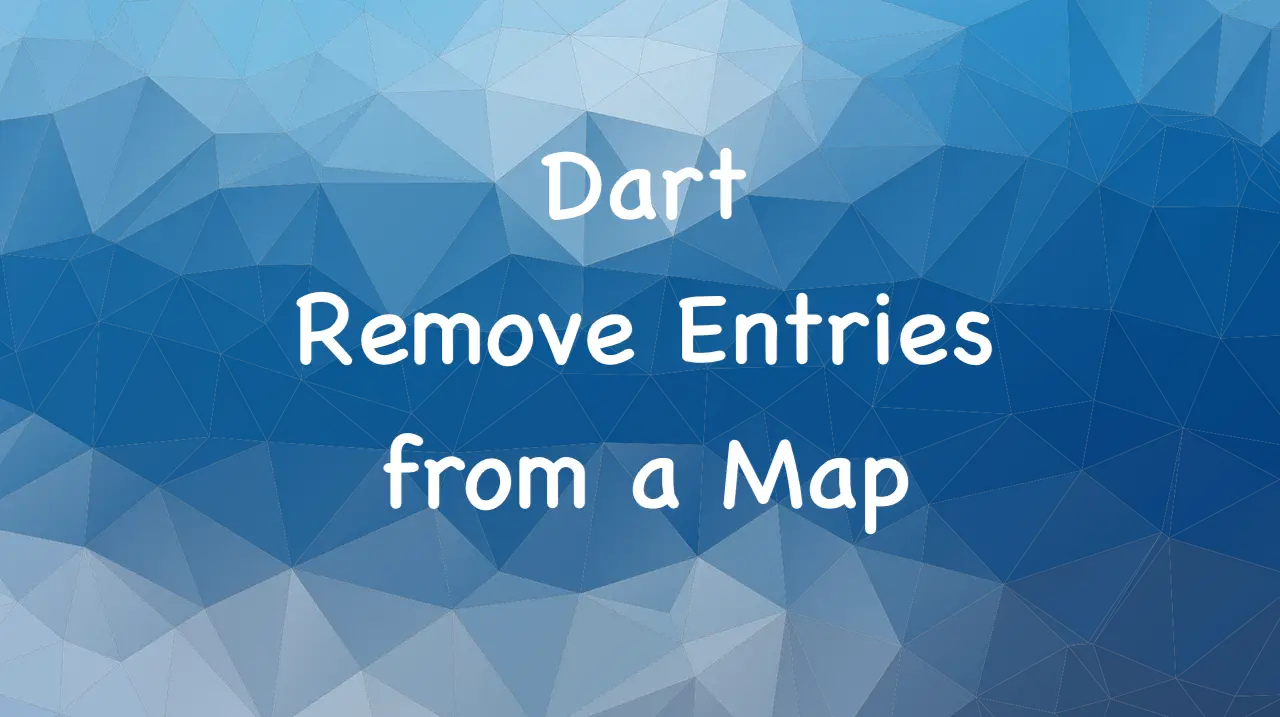
In Dart, you can remove key/value pairs from a given map by using one of the following methods:
- remove(): Removes the entry associated with the provided key. No error will occur even if the provided key doesn’t exist.
- removeWhere(): Removes all entries that satisfy the given conditions.
For more clarity, see the examples below.
Table of Contents
Example 1: Using remove()
This code snippet removes the entries that contain keys: age, occupation, and hometown.
// KindaCode.com
// main.dart
Map<String, dynamic> person = {
'name': 'John Doe',
'age': 39,
'occupation': 'Thief',
'hometown': 'Unknown',
'isMarried': false
};
void main() {
person.remove('age');
person.remove('occupation');
person.remove('hometown');
print(person);
}
Output:
name: John Doe, isMarried: false}
Example 2: Using removeWhere()
The following code removes entries with values of null or a whose key is secret:
// kindacode.com
Map<String, dynamic> project = {
'title': 'KindaCode.com: A Programming Website',
'age': 5,
'language': 'English',
'technologies': 'Unknown',
'traffic': null,
'contact': null,
'secret': 'Flying pan'
};
void main() {
project.removeWhere((key, value) => value == null || key == 'secret');
print(project);
}
Output:
{title: KindaCode.com: A Programming Website, age: 5, language: English, technologies: Unknown}
What’s Next?
Continue learning more about Dart and Flutter:
- Dart: Convert Timestamp to DateTime and vice versa
- Using Cascade Notation in Dart and Flutter
- Dart & Flutter: Get the Index of a Specific Element in a List
- Dart: Capitalize the First Letter of Each Word in a String
- Dart: Convert Map to Query String and vice versa
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.