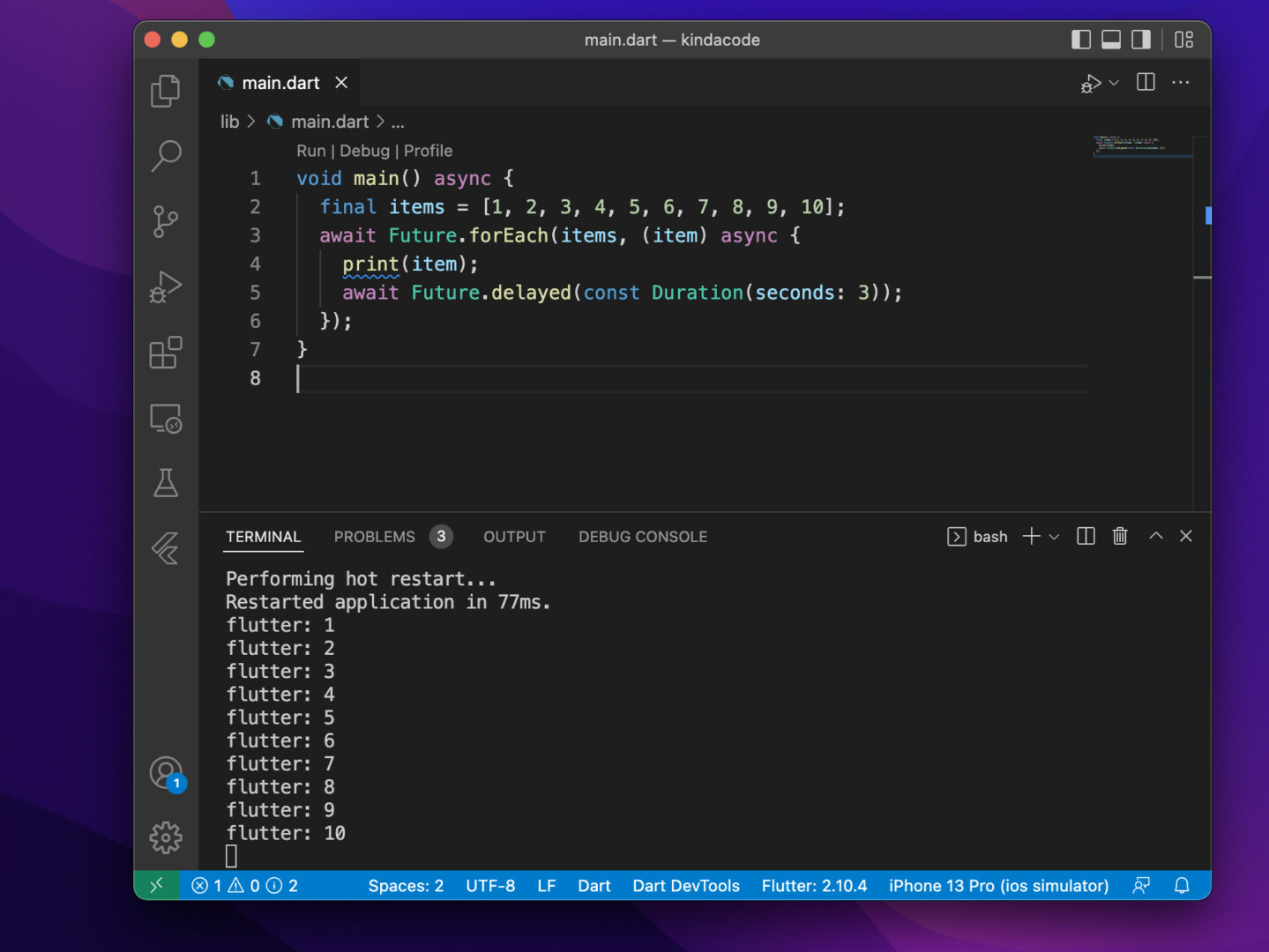
In Dart (and Flutter as well), you can perform synchronous operations sequentially in loops by using Future.forEach. The example program below will print the numbers from 1 to 10. Every time it finishes printing a number, it will wait for 3 seconds before printing the next number.
// kindacode.com
void main() async {
final items = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
await Future.forEach(items, (item) async {
print(item);
await Future.delayed(const Duration(seconds: 3));
});
}
If you want to get rid of the blue underline when calling the print() function, just add a kDebugMode check:
// kindacode.com
import 'package:flutter/foundation.dart';
void main() async {
final items = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
await Future.forEach(items, (item) async {
if (kDebugMode) {
print(item);
}
await Future.delayed(const Duration(seconds: 3));
});
}
Alternative
An alternative way is to use for … in syntax like this:
// kindacode.com
void main() async {
final items = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
for (int item in items) {
print(item);
await Future.delayed(const Duration(seconds: 3));
}
}
Further reading:
- 3 Ways to Cancel a Future in Flutter and Dart
- Dart: Converting a List to a Set and vice versa
- Sorting Lists in Dart and Flutter (5 Examples)
- Dart: Extract a substring from a given string (advanced)
- Working with MaterialBanner in Flutter
- Using Font Awesome Icons in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.