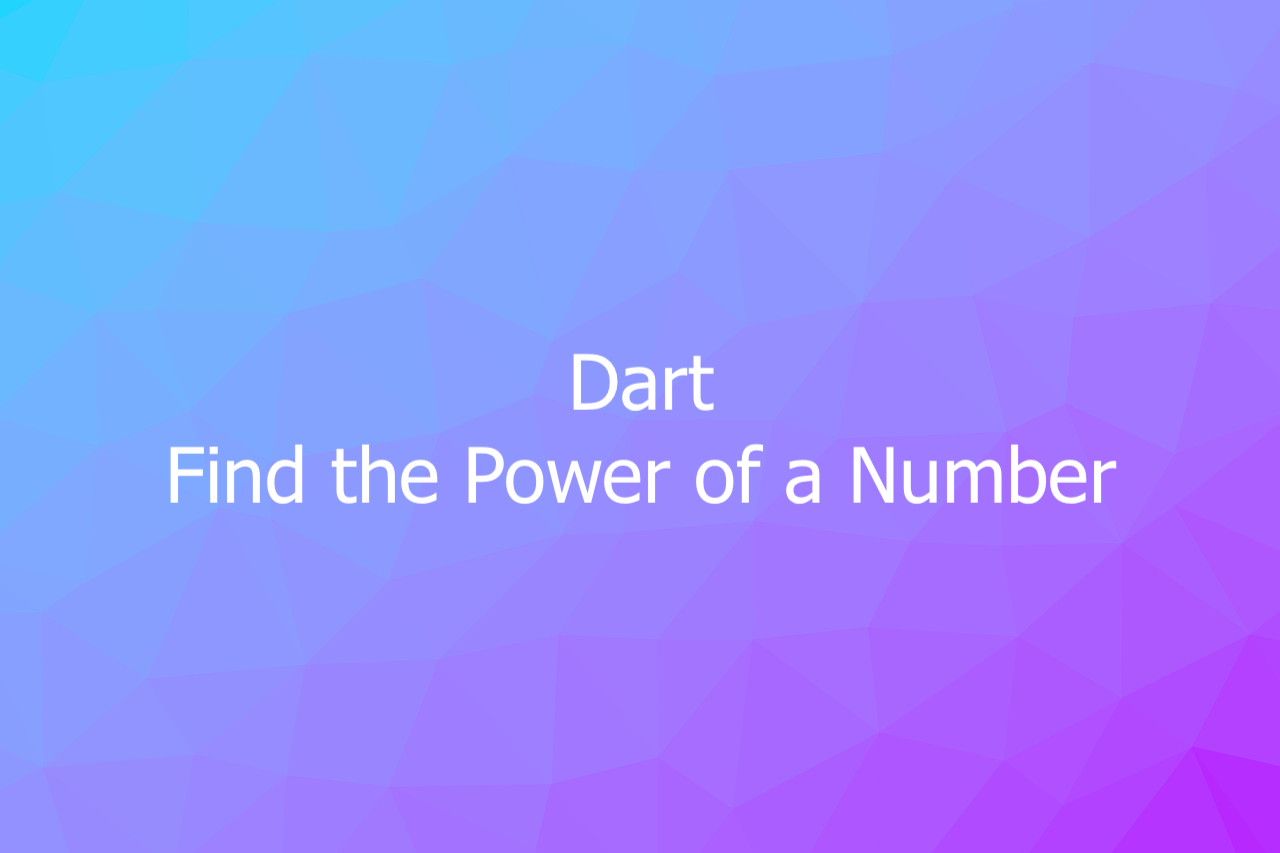
This concise and code-focused article shows you 2 different ways to find the power of a given number in Dart.
Using the pow() method
The pow() method from the math library (a built-in library of Dart) can be used for exponentiation. It takes two arguments: base and exponent. Here’s the syntax:
result = pow(base, exponent)
base and exponent can be integers or doubles, positive or negative, or zero.
Example:
import 'dart:math';
void main() {
var result1 = pow(2, 3);
var result2 = pow(-3, 3);
var result3 = pow(1.5, 1.5);
print('Result 1: $result1');
print('Result 2: $result2');
print('Result 3: $result3');
}
Output:
Result 1: 8
Result 2: -27
Result 3: 1.8371173070873836
Using a loop
Although the preceding approach is good and recommended, we still add this method for your reference.
Example
This example calculate the power of 5 to the 3rd power:
void main() {
int result = 1;
for (int i = 0; i < 3; i++) {
result *= 5;
}
print(result);
}
Output:
125
The limitation of this approach is that the exponent number must be a positive integer.
Further reading:
- How to add/remove a duration to/from a date in Dart
- 2 Ways to Get a Random Item from a List in Dart (and Flutter)
- 3 Ways to Cancel a Future in Flutter and Dart
- How to Create a Sortable ListView in Flutter
- Flutter: 2 Ways to Create an Onboarding Flow (Intro Slider)
- 2 Ways to Create Typewriter Effects in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.