There might be cases where you want to display some subscripts and superscripts in a Flutter application, such as mathematical formulas, chemical equations, or physics curves.
To render subscripts, you can set the style of Text or TextSpan widgets like this:
TextStyle(
fontFeatures: [FontFeature.subscripts()]),
/* other styles go here */
),
To render superscripts, you can set the style of Text or TextSpan widgets like this:
TextStyle(
fontFeatures: [FontFeature.superscripts()]),
/* other styles go here */
),
For more clarity, see the examples below.
Example 1: Using multiple Text widgets
Screenshot:
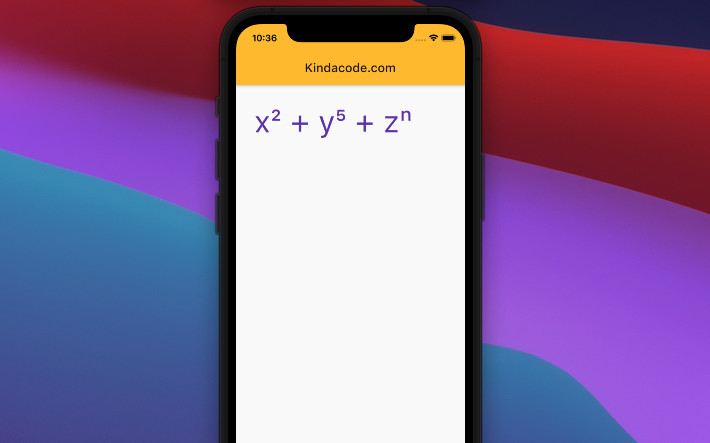
The code:
// KindaCode.com
// main.dart
import 'package:flutter/material.dart';
import 'dart:ui';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
// hide the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('KindaCode.com')),
body: const Padding(
padding: EdgeInsets.all(30),
child: DefaultTextStyle(
style: TextStyle(
fontSize: 50,
fontWeight: FontWeight.bold,
color: Colors.purple,
),
child: Wrap(
direction: Axis.horizontal,
children: [
Text('x'),
Text(
'2',
style: TextStyle(fontFeatures: [FontFeature.superscripts()]),
),
Text(' + '),
Text('y'),
Text(
'5',
style: TextStyle(fontFeatures: [FontFeature.superscripts()]),
),
Text(' + '),
Text('z'),
Text(
'n',
style: TextStyle(fontFeatures: [FontFeature.superscripts()]),
),
],
),
),
),
);
}
}
Example 2: Using RichText + TextSpan
Screenshot:
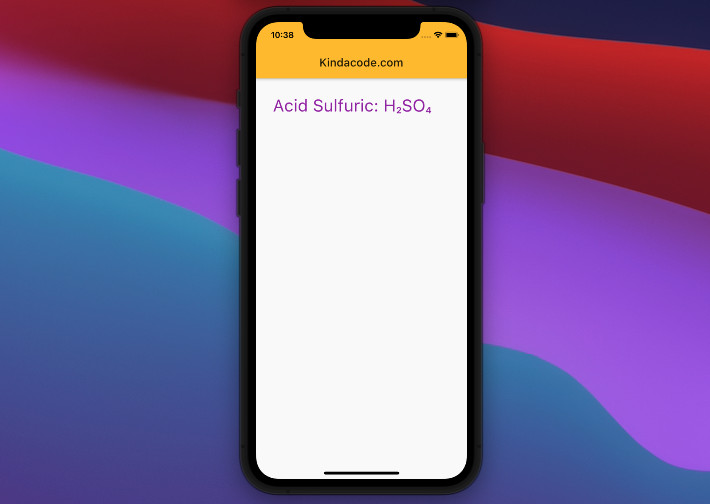
The full source code:
// main.dart
import 'package:flutter/material.dart';
import 'dart:ui';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(
primarySwatch: Colors.amber,
),
home: const HomePage());
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Padding(
padding: const EdgeInsets.all(30),
child: RichText(
text: const TextSpan(children: [
TextSpan(
text: 'Acid Sulfuric: ',
style: TextStyle(color: Colors.purple, fontSize: 30)),
TextSpan(
text: 'H',
style: TextStyle(color: Colors.purple, fontSize: 30)),
TextSpan(
text: '2',
style: TextStyle(
color: Colors.purple,
fontSize: 30,
fontFeatures: [FontFeature.subscripts()])),
TextSpan(
text: 'S',
style: TextStyle(color: Colors.purple, fontSize: 30),
),
TextSpan(
text: 'O',
style: TextStyle(color: Colors.purple, fontSize: 30),
),
TextSpan(
text: '4',
style: TextStyle(
color: Colors.purple,
fontSize: 30,
fontFeatures: [FontFeature.subscripts()]),
),
]),
),
));
}
}
What’s Next?
You’ve learned how to render subscripts and superscripts in Flutter and examined 2 examples of applying that knowledge in practice. If you want to render Math symbols or dynamic content that is loaded from APIs or databases:
Continue learning more new and interesting stuff about Flutter by taking a look at the following articles:
- Using RichText and TextSpan in Flutter
- A Complete Guide to Underlining Text in Flutter
- How to display Math Symbols in React
- Flutter: How to Read and Write Text Files
- How to encode/decode JSON in Flutter
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.