In React, you can render the current year like this:
<div>{new Date().getFullYear()}</div>
The complete example below presents a copyright symbol along with the current year (you can see this everywhere on the internet).
Screenshot:
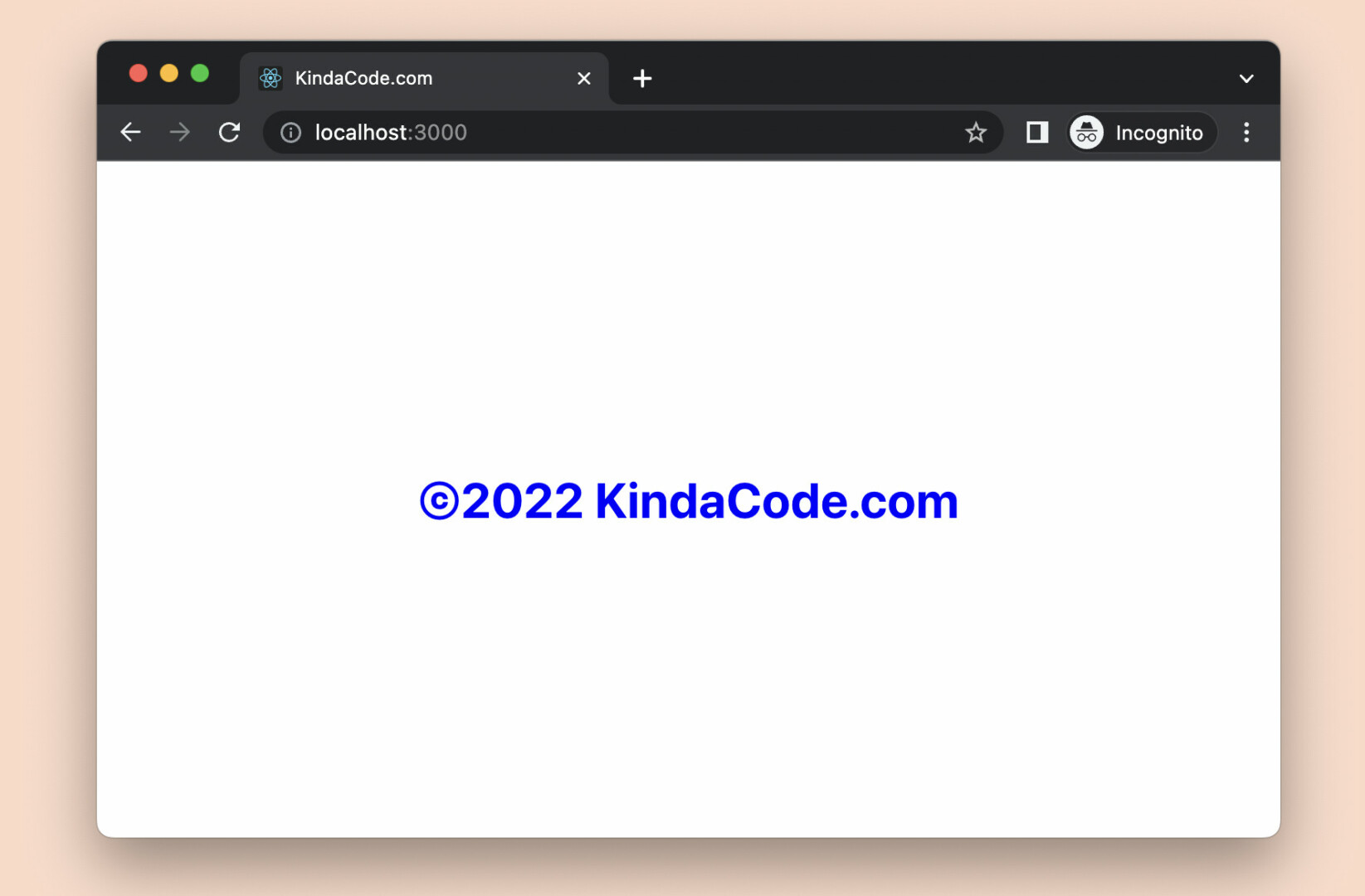
The code:
// KindaCode.com
// src/App.js
function App() {
return <div style={styles.container}>
<h1 style={styles.copyright}>©{new Date().getFullYear()} KindaCode.com</h1>
</div>;
}
const styles = {
container: {
width: '100vw',
height: '100vh',
display: 'flex',
alignItems: 'center',
justifyContent: 'center',
},
copyright: {
color: 'blue'
}
};
export default App;
Further reading:
- React + TypeScript: Using setTimeout() with Hooks
- React + TypeScript: setInterval() example (with hooks)
- React: How to Create an Image Carousel from Scratch
- React: How to Detect a Click Outside/Inside a Component
- React: 5+ Ways to Store Data Locally in Web Browsers
- React: Load and Display Data from Local JSON Files
You can also check our React category page and React Native category page for the latest tutorials and examples.