The RotatedBox widget is used to rotate its child by an integral number of quarter turns.
Usage:
RotatedBox(
quarterTurns: // an integer,
child: // child widget,
)
Example
This sample app makes use of the RotatedBox widget to rotate the colored box blocks with text.
Screenshot:
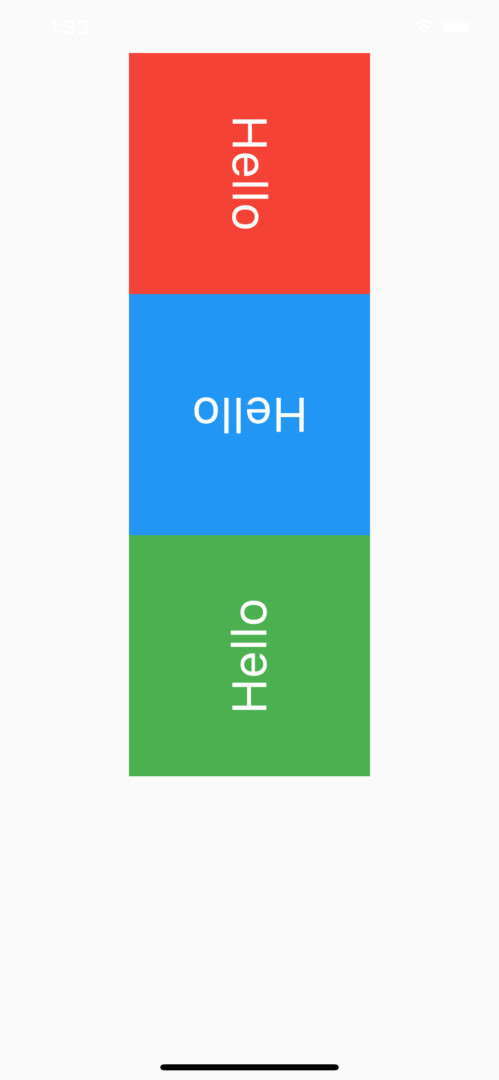
The code:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
// quarterTurns = 1, that means rotate 90 degrees
RotatedBox(
quarterTurns: 1,
child: Container(
width: 200,
height: 200,
color: Colors.red,
alignment: Alignment.center,
child: const Text(
'Hello',
style: TextStyle(color: Colors.white, fontSize: 40),
),
)),
// quarterTurns = 2, that means rotate 180 degrees
RotatedBox(
quarterTurns: 2,
child: Container(
width: 200,
height: 200,
color: Colors.blue,
alignment: Alignment.center,
child: const Text(
'Hello',
style: TextStyle(color: Colors.white, fontSize: 40),
),
)),
// quarterTurns = -1, that means rotate -90 degrees
RotatedBox(
quarterTurns: -1,
child: Container(
width: 200,
height: 200,
color: Colors.green,
alignment: Alignment.center,
child: const Text(
'Hello',
style: TextStyle(color: Colors.white, fontSize: 40),
),
)),
],
),
),
);
}
}
Further reading:
- Flutter: Making a Dropdown Multiselect with Checkboxes
- Working with dynamic Checkboxes in Flutter
- How to create Accordions in Flutter without plugins
- Flutter: Save Icon to Database, File, Shared Preferences
- Flutter PaginatedDataTable Example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.