In Flutter, the Opacity widget is used to make its child partially or completely transparent. It can take a child widget and an opacity (a double) argument which determines the child’s alpha value.
Example 1: Different levels of transparency
Screenshot:
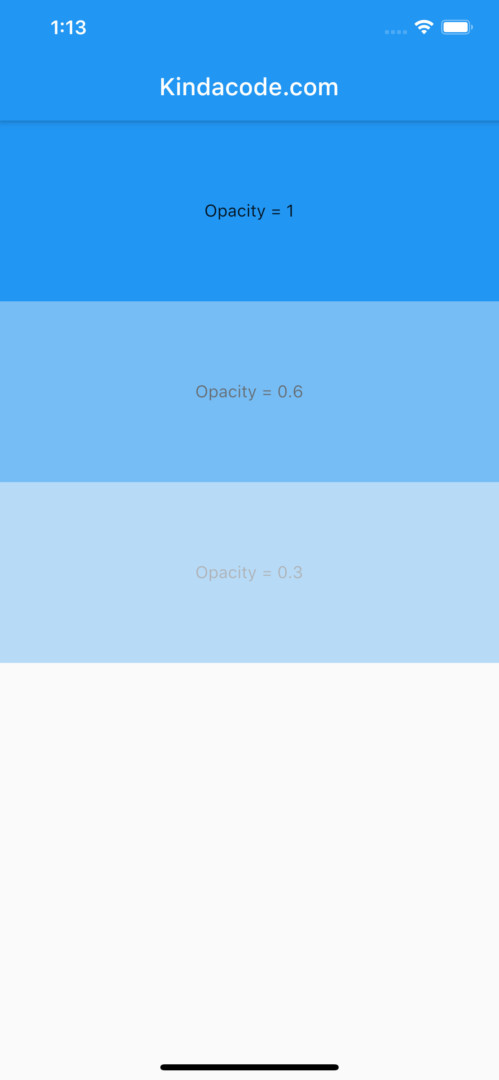
The code:
Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Column(
children: [
// Opacity = 1
Opacity(
opacity: 1,
child: Container(
width: double.infinity,
height: 150,
color: Colors.blue,
alignment: Alignment.center,
child: const Text('Opacity = 1'),
)),
// Opacity = 0.6
Opacity(
opacity: 0.6,
child: Container(
width: double.infinity,
height: 150,
color: Colors.blue,
alignment: Alignment.center,
child: const Text('Opacity = 0.6'),
)),
// Opacity = 0.3
Opacity(
opacity: 0.3,
child: Container(
width: double.infinity,
height: 150,
color: Colors.blue,
alignment: Alignment.center,
child: const Text('Opacity = 0.3'),
)),
// This one takes place but it's invisible
Opacity(
opacity: 0,
child: Container(
width: double.infinity,
height: 150,
color: Colors.blue,
alignment: Alignment.center,
child: const Text('Opacity = 0'),
)),
],
)
);
Example 2: Opacity & Image
Screenshot:
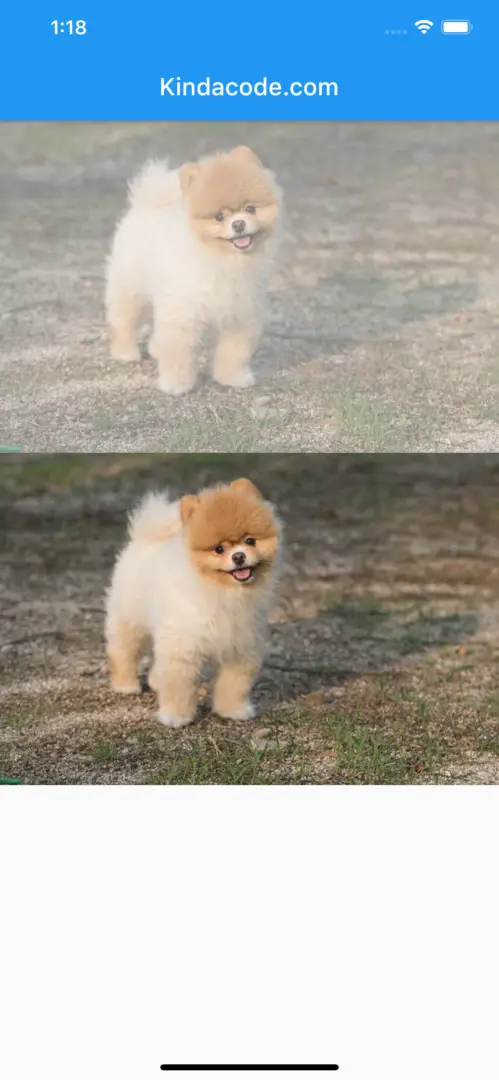
The code:
Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Column(
children: [
// Opacity = 0.5
Opacity(
opacity: 0.5,
child: Image.network(
'https://www.kindacode.com/wp-content/uploads/2020/11/my-dog.jpg',
fit: BoxFit.cover,
)),
// Opacity = 0.9
Opacity(
opacity: 0.9,
child: Image.network(
'https://www.kindacode.com/wp-content/uploads/2020/11/my-dog.jpg',
fit: BoxFit.cover,
)),
],
),
);
Example 3: Opacity & Gradient
Screenshot:
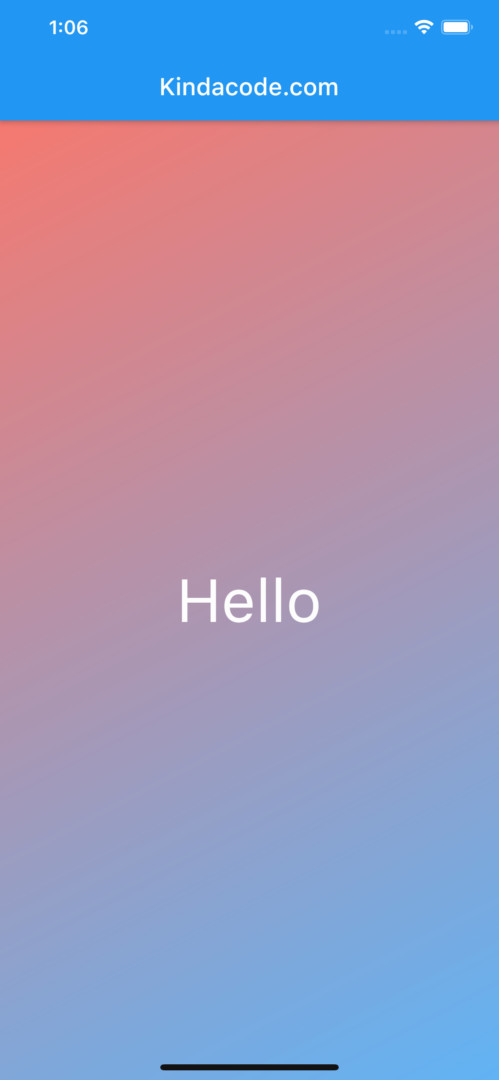
The code:
Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Opacity(
opacity: 0.7,
child: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
colors: [Colors.red, Colors.blue])),
alignment: Alignment.center,
child: const Text(
'Hello',
style: TextStyle(fontSize: 50, color: Colors.white),
),
),
)
);
References
- Opacity class (flutter.dev)
- Opacity (optics) on Wikipedia
- Opacity (developer.mozzilla.org)
Final Words
We’ve examined a few examples of using the Opacity widget to make more attractive user interfaces. If you’d like to explore more new and exciting stuff in Flutter, take a look at the following articles:
- Using Chip widget in Flutter: Tutorial & Examples
- How to create Blur Effects in Flutter
- How to make an image carousel in Flutter
- Flutter: GridPaper example
- Best Libraries for Making HTTP Requests in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.