In Flutter, you can add a border (and customize its thickness and color as well) to an elevated button by using the ElevatedButton.styleFrom() static method like this:
ElevatedButton(
style: ElevatedButton.styleFrom(
side: const BorderSide(
width: 2, // the thickness
color: Colors.black // the color of the border
)
),
/* ... */
),
In case you need to create a rounded border, use this implementation:
ElevatedButton(
style: ElevatedButton.styleFrom(
shape: RoundedRectangleBorder(
side: const BorderSide(
width: 8, // thickness
color: Colors.deepPurple // color
),
// border radius
borderRadius: BorderRadius.circular(16)
)
),
/* ... */
),
Let’s examine the concrete example below for more clearness.
Screenshot:
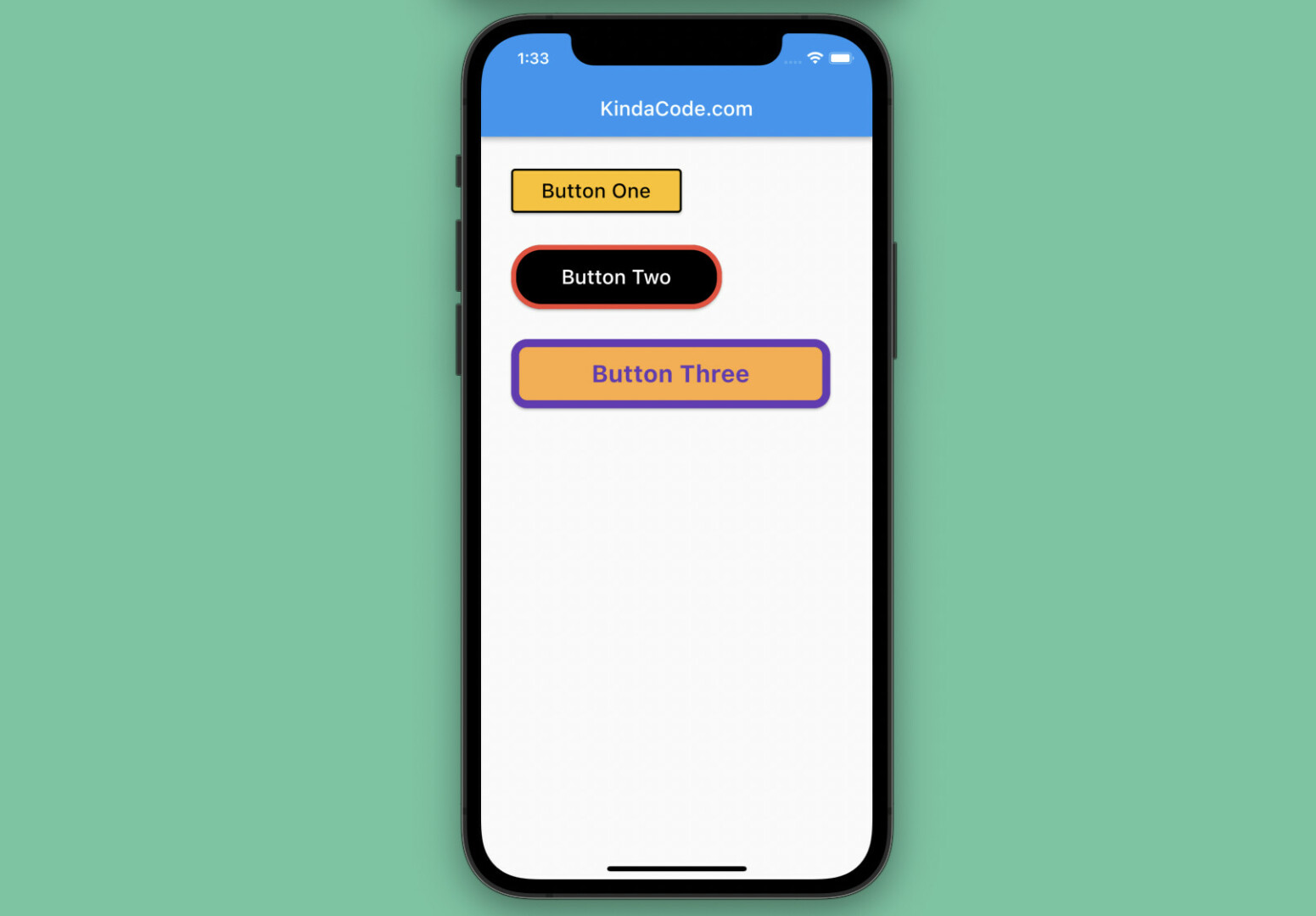
The code:
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
// Button One (a rectangular button)
ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(
side: const BorderSide(width: 2, color: Colors.black),
primary: Colors.amber,
padding:
const EdgeInsets.symmetric(vertical: 10, horizontal: 30)),
child: const Text(
'Button One',
style: TextStyle(color: Colors.black, fontSize: 20),
),
),
const SizedBox(
height: 30,
),
// Button Two (a pill button)
ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(
side: const BorderSide(width: 5, color: Colors.red),
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(30)),
primary: Colors.black,
padding:
const EdgeInsets.symmetric(vertical: 20, horizontal: 50)),
child: const Text(
'Button Two',
style: TextStyle(fontSize: 20, color: Colors.white),
),
),
const SizedBox(
height: 30,
),
// Button Three (a rounded button)
ElevatedButton(
onPressed: () {},
style: ElevatedButton.styleFrom(
shape: RoundedRectangleBorder(
side:
const BorderSide(width: 8, color: Colors.deepPurple),
borderRadius: BorderRadius.circular(16)),
primary: Colors.orangeAccent,
padding:
const EdgeInsets.symmetric(vertical: 20, horizontal: 80)),
child: const Text(
'Button Three',
style: TextStyle(
fontSize: 24,
fontWeight: FontWeight.bold,
color: Colors.deepPurple),
),
),
],
),
Further reading:
- Working with ElevatedButton in Flutter
- Working with OutlinedButton in Flutter
- Working with TextButton in Flutter
- Flutter and Firestore Database: CRUD example
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- How to Programmatically Take Screenshots in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.