Flutter provides several built-in widgets that can help you create a bottom bar for your app (BottomAppBar, BottomNavigationBar, CupertinoBottomTab, and maybe NavigationRail). However, there might be cases where you want to make a special bottom bar with stunning animation effects. In these scenarios, taking advantage of an open-source plugin is time-saving and headache-reducing. This article walks you through a list of best packages to implement cool bottom app bars in Flutter (the main criteria are the numbers of likes on pub.dev and stars on GitHub).
Convex Bottom Bar
Summary:
- Pub likes: 2.3k+
- GitHub stars: 700+
- License: Apache-2.0
- Written in: Dart, Shell
- Links: Pub page | GitHub repo | Documentation
At the time of writing, convex_bottom_bar is one of the most popular plugins on pub.dev. It helps you conveniently show a convex tab in the bottom bar.
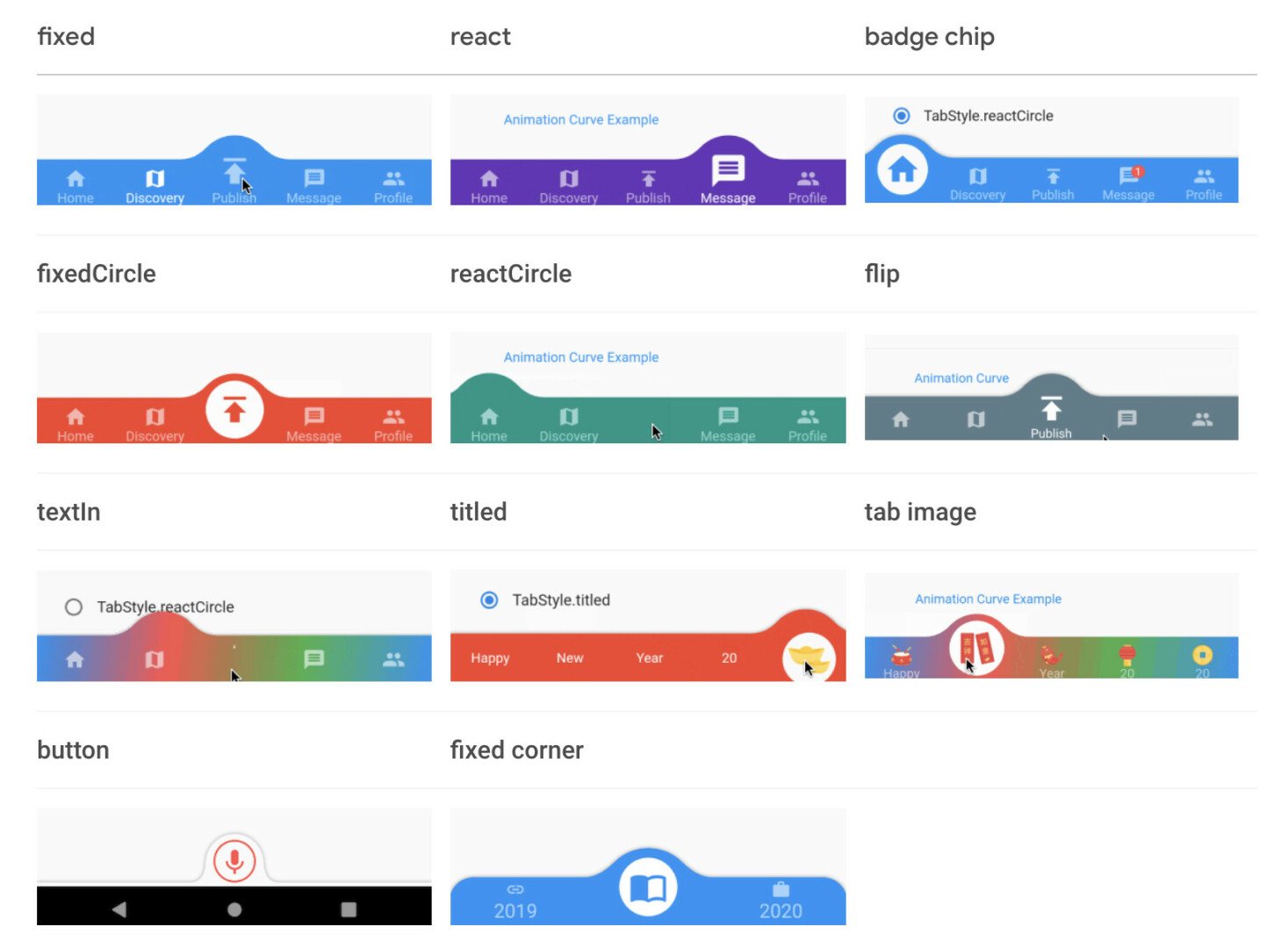
Key features:
- Theming supported
- Various internal styles and hook API to override some of these internal styles
- Builder API to customize new style on your own
- Elegant transition animation
- Badges
You can install the plugin by running the following command:
flutter pub add convex_bottom_bar
Then use it like so:
import 'package:convex_bottom_bar/convex_bottom_bar.dart';
Scaffold(
bottomNavigationBar: ConvexAppBar(
items: [
TabItem(icon: Icons.home, title: 'Home'),
TabItem(icon: Icons.map, title: 'Discovery'),
TabItem(icon: Icons.add, title: 'Add'),
],
onTap: (int i) => print('click index=$i'),
)
);
Bottom Navy Bar
Summary:
- Pub likes: 1.2+
- GitHub stars: 930+
- License: Apache-2.0
- Written in: Dart, Objective-C, Java
- Links: Pub page | GitHub repo | Documentation
This package helps you create a beautiful and animated bottom navigation bar.
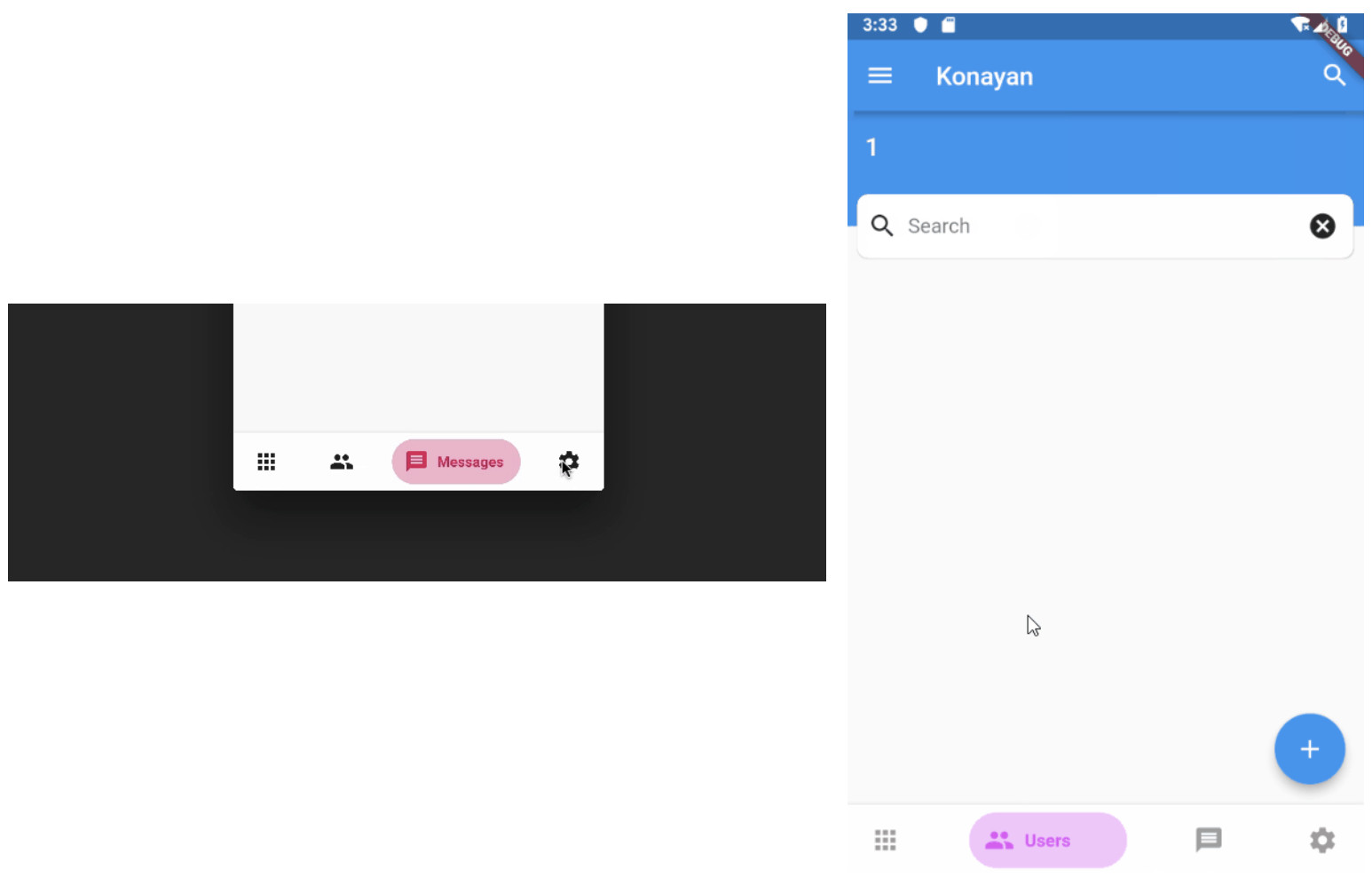
By default, BottomNavyBar uses your app’s theme. However, you can customize it as you want. To install the package, perform the following command:
flutter pub add bottom_navy_bar
Sample usage:
import 'package:bottom_navy_bar/bottom_navy_bar.dart';
// the currently selected page
int _selectedIndex = 0;
Scaffold(
/* Other code */
bottomNavigationBar: BottomNavyBar(
selectedIndex: _selectedIndex,
showElevation: true,
onItemSelected: (index) => setState(() {
_selectedIndex = index;
_pageController.animateToPage(index,
duration: Duration(milliseconds: 300), curve: Curves.ease);
}),
items: [
BottomNavyBarItem(
icon: Icon(Icons.home),
title: Text('Home'),
activeColor: Colors.red,
),
BottomNavyBarItem(
icon: Icon(Icons.favorite),
title: Text('Favorites'),
activeColor: Colors.purpleAccent
),
BottomNavyBarItem(
icon: Icon(Icons.settings),
title: Text('Settings'),
activeColor: Colors.blue
),
],
)
)
Animated Bottom Navigation Bar
Overview:
- Pub likes: 964+
- GitHub stars: 254+
- License: BSD-2-Clause
- Written in: Dart
- Links: Pub page | GitHub repo | Documentation
This package helps you implement an animated bottom nav bar with a notch (or without a notch). It is highly customizable and easy to use.
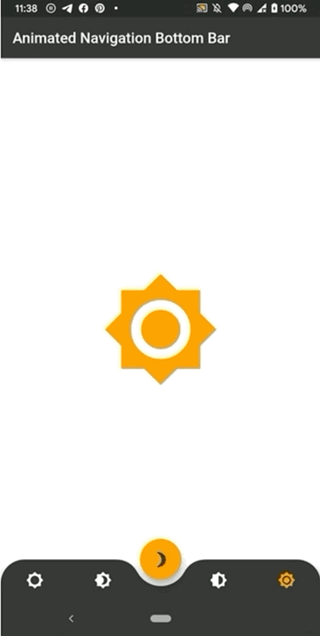
Install:
flutter pub add animated_bottom_navigation_bar
Quick example:
import 'package:animated_bottom_navigation_bar/animated_bottom_navigation_bar.dart';
int _bottomNavIndex = 0;
Scaffold(
floatingActionButton: FloatingActionButton(/* ... */),
floatingActionButtonLocation: FloatingActionButtonLocation.centerDocked,
bottomNavigationBar: AnimatedBottomNavigationBar(
icons: iconList,
activeIndex: _bottomNavIndex,
gapLocation: GapLocation.center,
notchSmoothness: NotchSmoothness.verySmoothEdge,
leftCornerRadius: 32,
rightCornerRadius: 32,
onTap: (index) => setState(() => _bottomNavIndex = index),
//other params
),
);
Salomon Bottom Bar
Some Insights:
- Pub likes: 780+
- GitHub stars: 202+
- License: MIT
- Written in: Dart, HTML, Swift
- Links: Pub page | GitHub repo | Documentation
This package helps you easily implement an animated, succinct, gorgeous bottom bar.
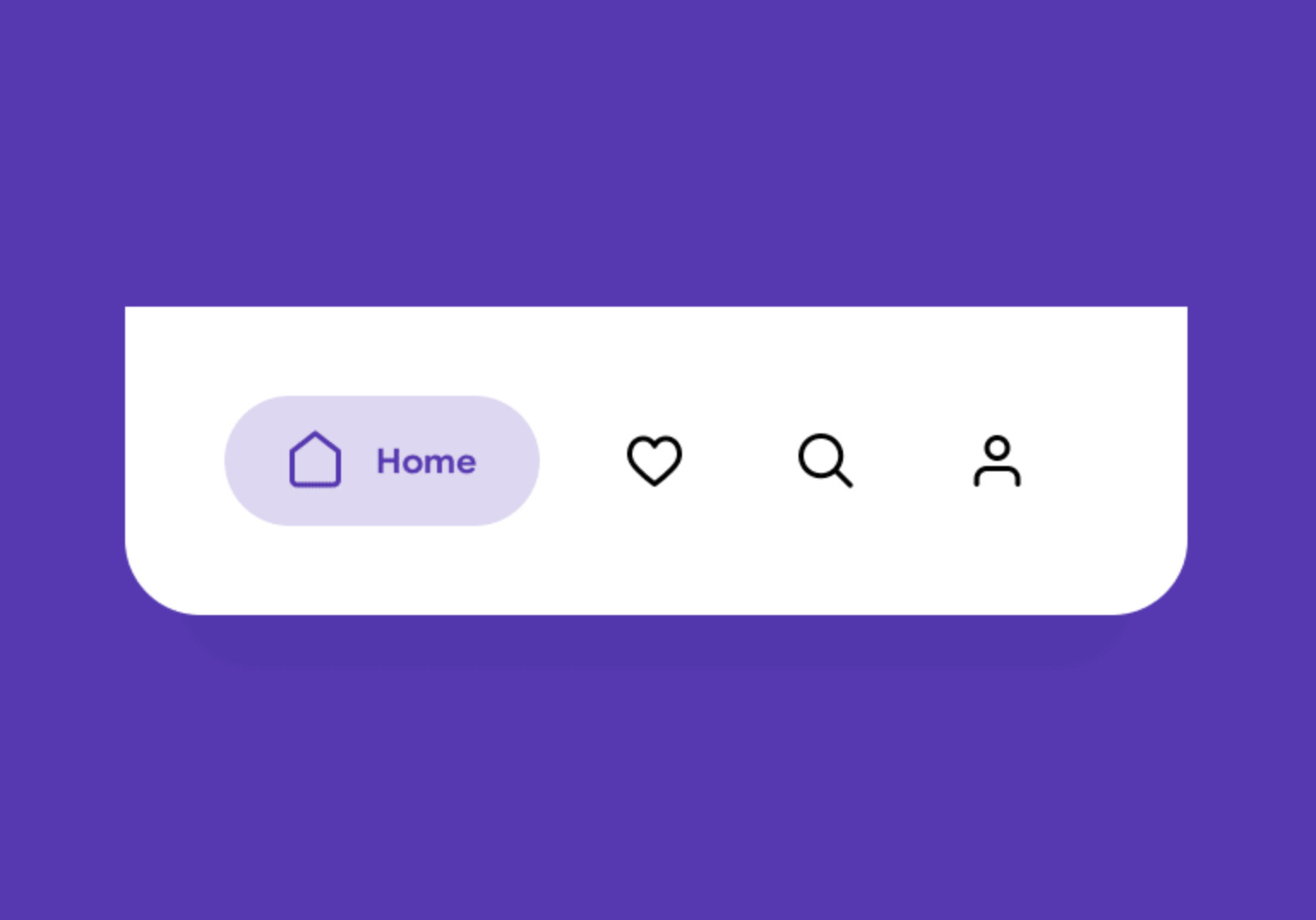
To install the plugin, run the command below:
flutter pub add salomon_bottom_bar
Then use it like so:
import 'package:salomon_bottom_bar/salomon_bottom_bar.dart';
int _currentIndex = 0;
Scaffold(
appBar: AppBar(
title: Text(MyApp.title),
),
bottomNavigationBar: SalomonBottomBar(
currentIndex: _currentIndex,
onTap: (i) => setState(() => _currentIndex = i),
items: [
/// Home
SalomonBottomBarItem(
icon: Icon(Icons.home),
title: Text("Home"),
selectedColor: Colors.purple,
),
/// Likes
SalomonBottomBarItem(
icon: Icon(Icons.favorite_border),
title: Text("Likes"),
selectedColor: Colors.pink,
),
/// Search
SalomonBottomBarItem(
icon: Icon(Icons.search),
title: Text("Search"),
selectedColor: Colors.orange,
),
/// Profile
SalomonBottomBarItem(
icon: Icon(Icons.person),
title: Text("Profile"),
selectedColor: Colors.teal,
),
],
),
)
Bottom Bar With Sheet
Summary:
- Pub likes: 474+
- GitHub stars: 377+
- License: Dart, C++, CMake, HTML, Swift
- Written in: Dart, C++, CMake, HTML
- Links: Pub page | GitHub repo | Documentation
This package helps you implement a kind of combination of a bottom nav bar with a bottom sheet. You can also add a big action button as needed. It’s hard to be depicted by words. Let’s see the GIF below for more understanding:
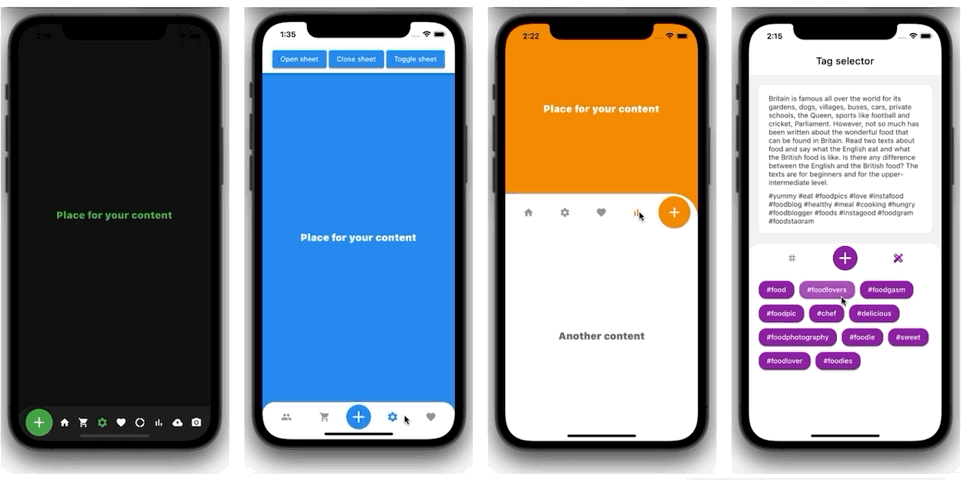
Install:
flutter pub add bottom_bar_with_sheet
Quick example:
import 'package:bottom_bar_with_sheet/bottom_bar_with_sheet.dart';
/*...*/
Scaffold(
bottomNavigationBar: BottomBarWithSheet(
controller: _bottomBarController,
bottomBarTheme: const BottomBarTheme(
decoration: BoxDecoration(color: Colors.white),
itemIconColor: Colors.grey,
),
items: const [
BottomBarWithSheetItem(icon: Icons.people),
BottomBarWithSheetItem(icon: Icons.favorite),
],
),
)
Bubble Bottom Bar
Overview:
- Pub likes: 221+
- GitHub stars: 285+
- License: MIT
- Written in: Dart, Java, Objective-C, Shell
- Links: Pub page | GitHub repo | Documentation
Another nice package for making bottom app bars in Flutter.
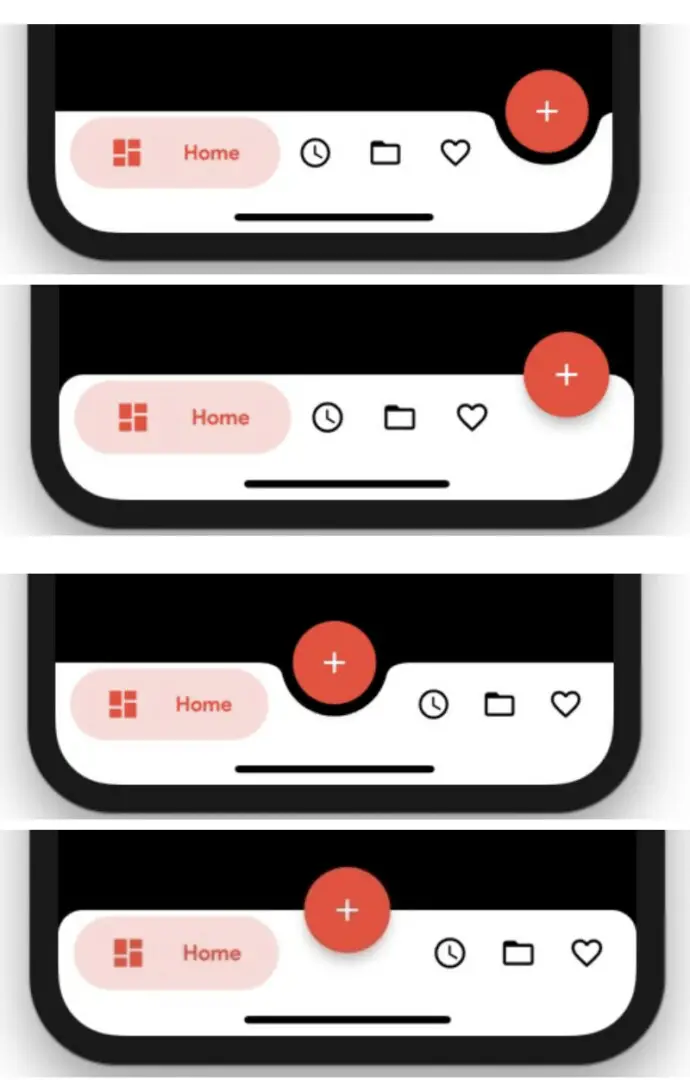
Installing:
flutter pub add bubble_bottom_bar
Quick example:
import 'package:bubble_bottom_bar/bubble_bottom_bar.dart';
/* ... */
Scaffold(
floatingActionButton: FloatingActionButton(
onPressed: (){},
child: Icon(Icons.add),
backgroundColor: Colors.red,
),
floatingActionButtonLocation: FloatingActionButtonLocation.endDocked,
bottomNavigationBar: BubbleBottomBar(
opacity: .2,
currentIndex: currentIndex,
onTap: changePage,
borderRadius: BorderRadius.vertical(top: Radius.circular(16)),
elevation: 8,
fabLocation: BubbleBottomBarFabLocation.end, //new
hasNotch: true, //new
hasInk: true, //new, gives a cute ink effect
inkColor: Colors.black12, //optional, uses theme color if not specified
items: <BubbleBottomBarItem>[
BubbleBottomBarItem(backgroundColor: Colors.red, icon: Icon(Icons.dashboard, color: Colors.black,), activeIcon: Icon(Icons.dashboard, color: Colors.red,), title: Text("Home")),
BubbleBottomBarItem(backgroundColor: Colors.deepPurple, icon: Icon(Icons.access_time, color: Colors.black,), activeIcon: Icon(Icons.access_time, color: Colors.deepPurple,), title: Text("Logs")),
BubbleBottomBarItem(backgroundColor: Colors.indigo, icon: Icon(Icons.folder_open, color: Colors.black,), activeIcon: Icon(Icons.folder_open, color: Colors.indigo,), title: Text("Folders")),
BubbleBottomBarItem(backgroundColor: Colors.green, icon: Icon(Icons.menu, color: Colors.black,), activeIcon: Icon(Icons.menu, color: Colors.green,), title: Text("Menu"))
],
),
)
Wrapping Up
We’ve covered the most popular open-source bottom bar packages for Flutter. All of them are null-safety and well-documented. Choose from them the one that suits your needs. If you find something obsolete or incorrect, please let us know by leaving a comment.
Flutter is awesome and there are so many interesting things to learn. Keep your motivation and continue strengthening your skills by taking a look at the following articles:
- Working with ListWheelScrollView in Flutter (2 Examples)
- Using GetX (Get) for State Management in Flutter
- Using GetX (Get) for Navigation and Routing in Flutter
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Flutter: SliverGrid example
- Flutter AnimatedList – Tutorial and Examples
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.