In Flutter, you can use the ClipRRect widget to create rounded corners rectangle widgets, or even circular widgets. Let’s walk through the following examples to get a better understanding.
Example 1: Rounded corners Container
The code:
Center(
child: Padding(
padding: const EdgeInsets.all(15),
child: ClipRRect(
borderRadius: BorderRadius.circular(100),
child: Container(
width: 300, height: 300, color: Colors.blue[200]))),
),
Output:
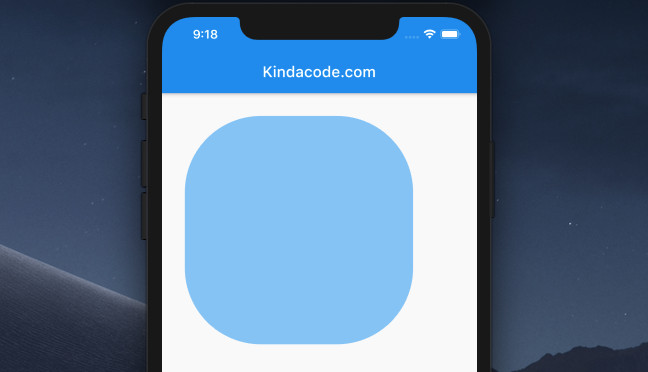
Example 2: Circle Container
The code:
ClipRRect(
// the radius must be at least half of the width of the child Container
borderRadius: BorderRadius.circular(600),
child:
Container(width: 300, height: 300, color: Colors.blue[200])
)
Output:
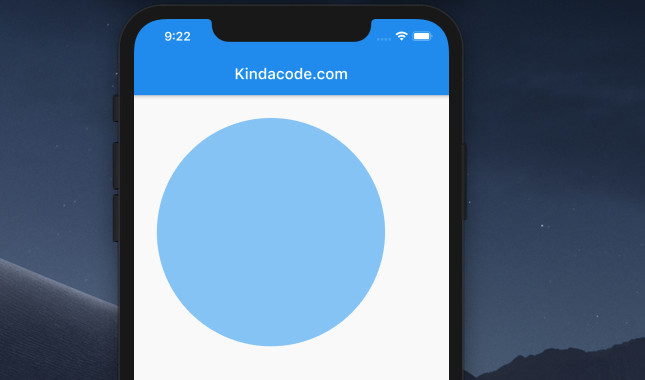
Example 3: Make a circle image
The code:
Center(
child: ClipRRect(
borderRadius: BorderRadius.circular(150),
child: Image.network(
'https://www.kindacode.com/wp-content/uploads/2020/11/my-dog.jpg',
width: 300,
height: 300,
fit: BoxFit.cover,
),
)
)
Output:
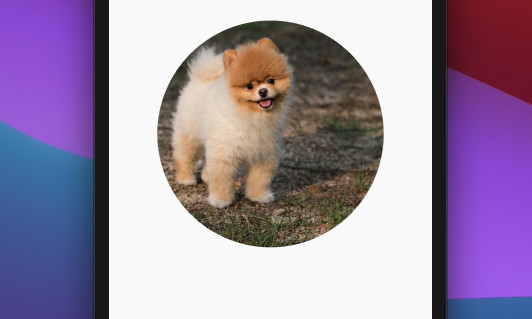
Note: You can also use the CircleAvatar widget to make a circular image in Flutter.
Example 4: Make a circular ElevatedButton
The code:
Center(
child: ClipRRect(
borderRadius: BorderRadius.circular(100),
child: SizedBox(
width: 200,
height: 200,
child: ElevatedButton(
onPressed: () {},
child: const Text('Button', style: TextStyle(fontSize: 24),),
),
),
))
Output:
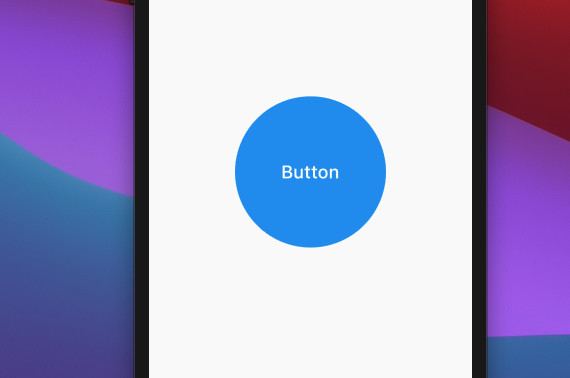
Wrapping Up
We’ve gone through some practical examples of using the ClipRRect widget to create rounded sharp things. If you’d like to explore more new and interesting stuff in the modern Flutter world, take a look at the following articles:
- Using Chip widget in Flutter: Tutorial & Examples
- Flutter: Drawing Polygons using ClipPath (4 Examples)
- Flutter: How to Draw a Heart with CustomPaint
- Flutter: Drawing an N-Pointed Star with CustomClipper
- Flutter: Making a Tic Tac Toe Game from Scratch
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.