This article walks you through 3 examples of setting borders for a Container in Flutter.
Example 1: Set a border for all sides
This example creates a square yellow box enclosed by a red border.
Preview:
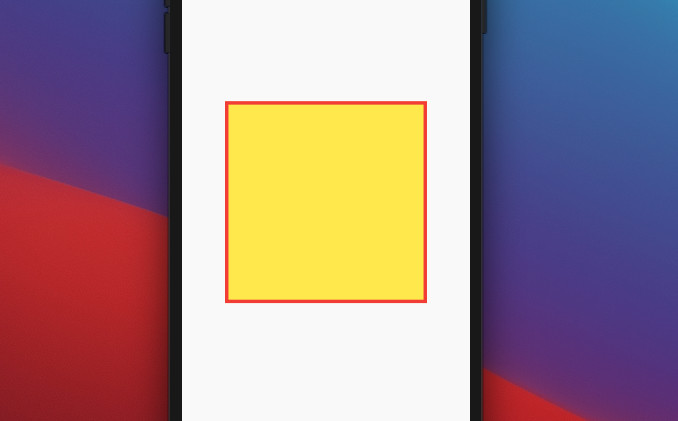
The code:
Center(
child: Container(
width: 300,
height: 300,
decoration: BoxDecoration(
color: Colors.yellow,
// Red border with the width is equal to 5
border: Border.all(
width: 5,
color: Colors.red
)
),
),
)
Example 2: Set a different border for each side
In this example, we specify a different border for each side of a box (top, right, bottom, and left) by using the BorderSide class.
Preview:
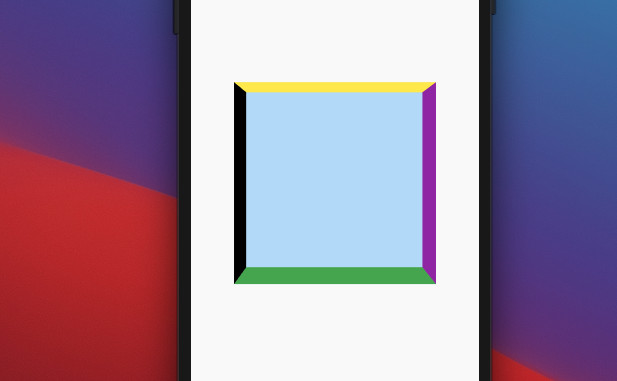
The code:
Center(
child: Container(
width: 300,
height: 300,
decoration: BoxDecoration(
color: Colors.blue[100],
// Set a border for each side of the box
border: const Border(
top: BorderSide(width: 15, color: Colors.yellow),
right: BorderSide(width: 20, color: Colors.purple),
bottom: BorderSide(width: 25, color: Colors.green),
left: BorderSide(width: 18, color: Colors.black)
)
),
))
Example 3: A border with rounded corners
Preview:
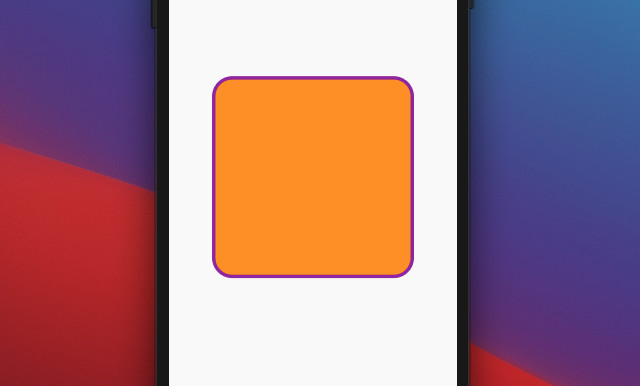
The code:
Center(
child: Container(
width: 300,
height: 300,
decoration: BoxDecoration(
color: Colors.orange,
border: Border.all(
width: 5,
color: Colors.purple,
),
// Make rounded corners
borderRadius: BorderRadius.circular(30)
),
))
Conclusion
In this article, you explored several examples of how to set borders for a Container in Flutter. If you’d like to learn more new and interesting things about that amazing technology, take a look at the following articles:
- Flutter Gradient Text Examples
- Flutter: Adding a Border to an Elevated Button
- Flutter: Adding a Gradient Border to a Container (2 Examples)
- Flutter: AnimatedContainer example
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
- Flutter: Showing a badge on the Top Right of a widget
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.