In Flutter, you can add a gradient background to a Container by using the gradient property of the BoxDecoration class. Below are a few examples of doing so in practice.
Example 1: LinearGradient Background
The code:
Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.purple[200]!, Colors.amber],
begin: Alignment.topLeft,
end: Alignment.bottomRight),
),
),
);
Screenshot:
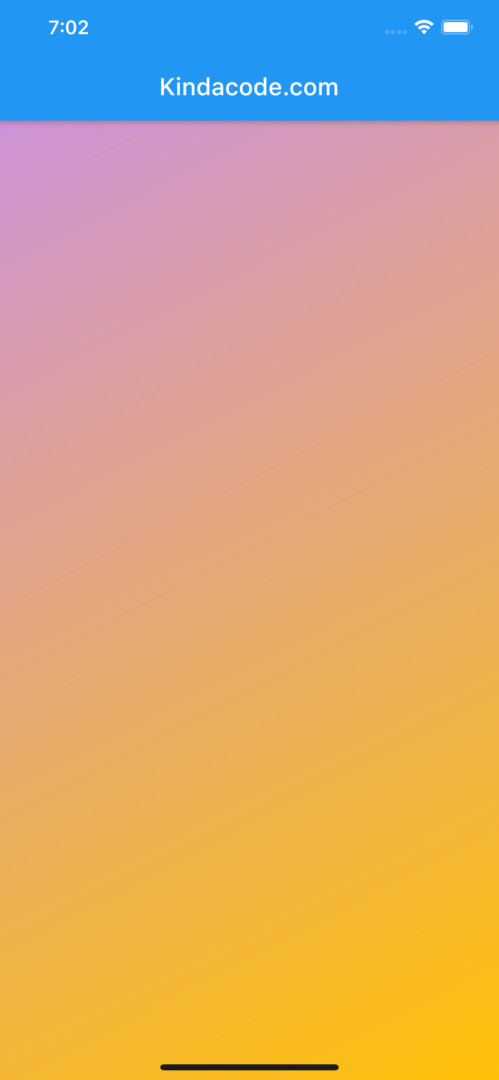
Example 2: RadialGradient Background
The code:
Scaffold(
body: Container(
decoration: const BoxDecoration(
gradient: RadialGradient(
center: Alignment.center, colors: [Colors.yellow, Colors.red])),
),
);
Screenshot:
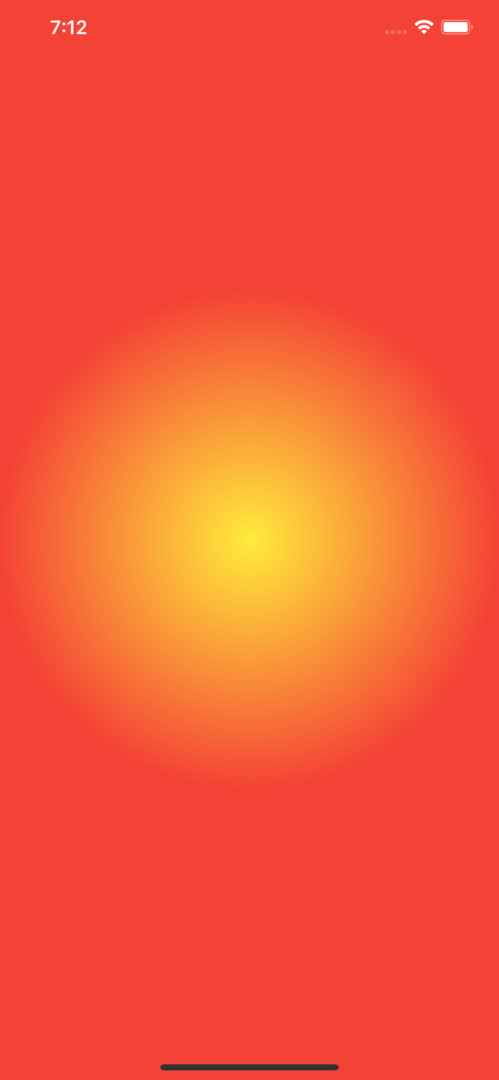
Example 3: SweepGradient
The code:
Scaffold(
body: Container(
decoration: const BoxDecoration(
gradient: SweepGradient(
center: Alignment.center, colors: [Colors.green, Colors.yellow])),
));
Screenshot:
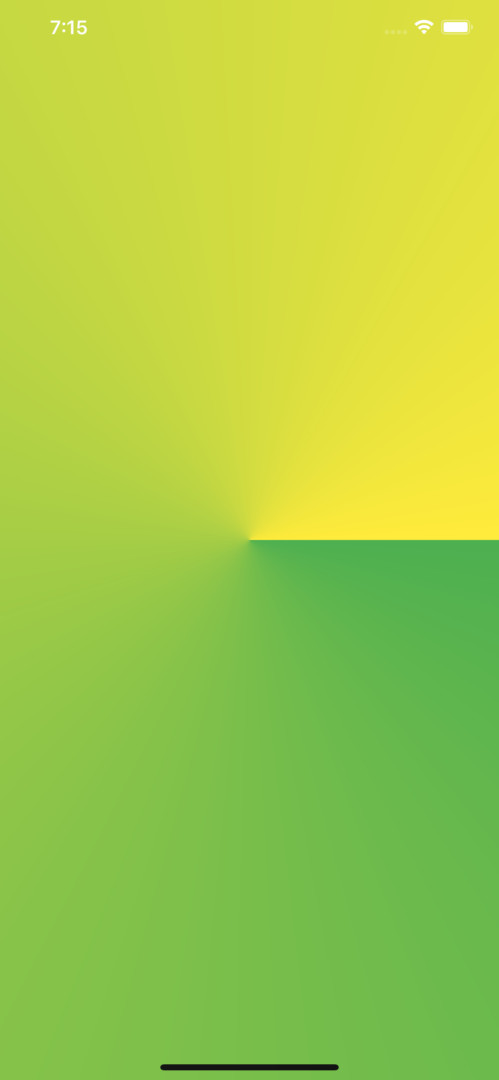
Wrap Up
We’ve gone through a couple of examples of creating gradient background Containers in Flutter with the BoxDecoration class and the gradient property. If you’d like to explore more new and exciting things in Flutter and Dart, take a look at the following articles:
- Flutter: Create a Password Strength Checker from Scratch
- Flutter: How to Draw a Heart with CustomPaint
- Implementing Tooltips in Flutter
- Flutter: Drawing an N-Pointed Star with CustomClipper
- Flutter Gradient Text Examples
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.