This concise article shows you how to create a strikethrough text (cross-out text) in Flutter.
Table of Contents
The TL;DR
You can get the job done easily by setting the decoration property of the TextStyle class to TextDecoration.lineThrough, like this:
Text(
'KindaCode.com',
style: TextStyle(decoration: TextDecoration.lineThrough),
)
The Example
A common use case for using strikethrough text is displaying both the usual price and the discount price of a product. You will very likely encounter this task when developing a shop or an e-commerce app.
Screenshot:
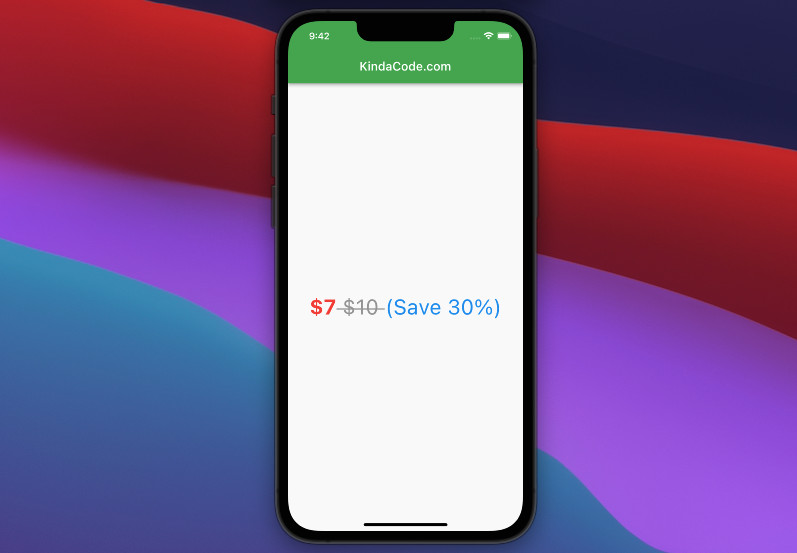
The code
You can put multiple Text widgets with different styles inside a Row widget to produce the app shown in the screenshot above:
Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: const [
Text(
'\$7',
style: TextStyle(
fontSize: 35, color: Colors.red, fontWeight: FontWeight.bold),
),
Text(
' \$10 ',
style: TextStyle(
fontSize: 35,
decoration: TextDecoration.lineThrough,
color: Colors.grey),
),
Text(
'(Save 30%)',
style: TextStyle(fontSize: 35, color: Colors.blue),
)
],
),
),
);
Conclusion
You’ve learned how to add some strikethrough text into your app. If you want to explore more about what you can do with a Text widget or other interesting things in Flutter, take a look at the following articles:
- Adding a Border to Text in Flutter
- Flutter Gradient Text Examples
- Flutter Vertical Text Direction: An Example
- Flutter: Dynamic Text Color Based on Background Brightness
- Using RichText and TextSpan in Flutter
- Flutter: Showing a Context Menu on Long Press
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.