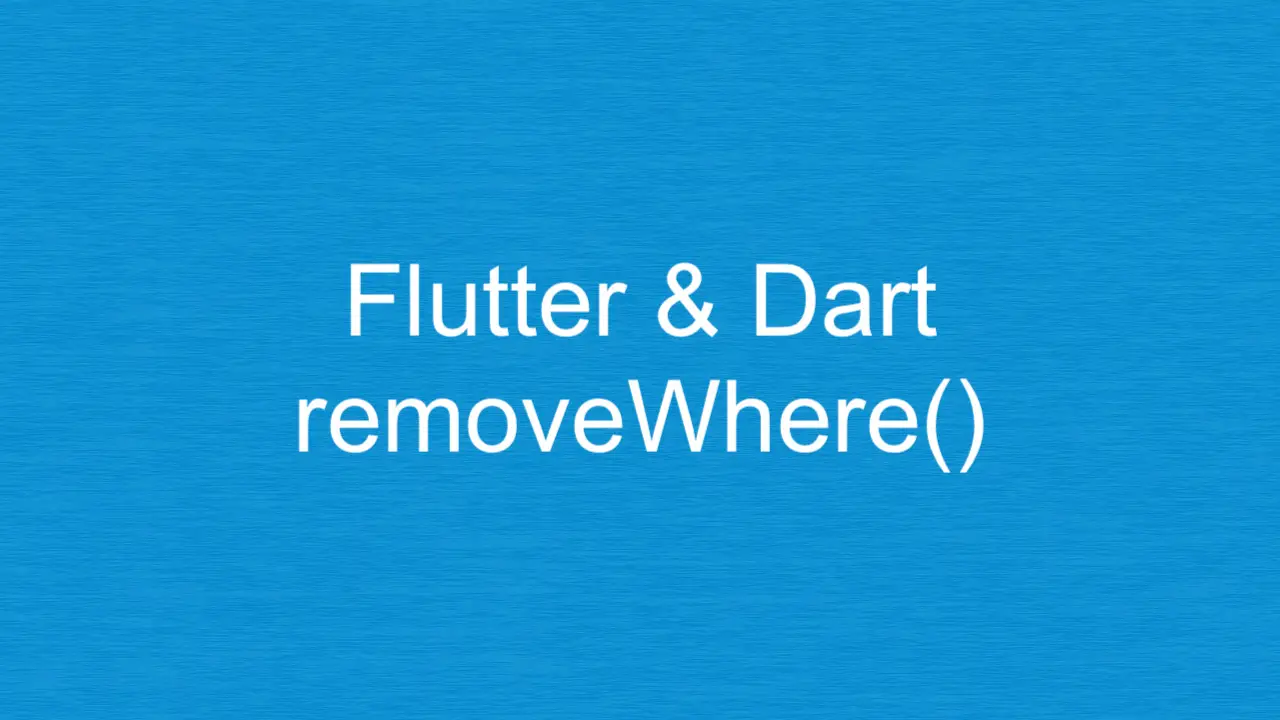
To remove elements from a list based on one or many conditions, you can use the built-in removeWhere() method.
Example
Let’s say we have a product list, and the task is to remove all products whose prices are higher than 100:
import 'package:flutter/foundation.dart';
// Define how a product looks like
class Product {
final double id;
final String name;
final double price;
Product(this.id, this.name, this.price);
}
void main() {
// Generate the product list
List<Product> products = [
Product(1, "Product A", 50),
Product(2, "Product B", 101),
Product(3, "Product C", 200),
Product(4, "Product D", 90),
Product(5, "Product E", 400),
Product(6, "Product F", 777)
];
// remove products whose prices are greater than 100
products.removeWhere((product) => product.price > 100);
// Print the result
debugPrint("Product(s) whose prices are less than or equal to 100:");
for (var product in products) {
debugPrint(product.name);
}
}
Output:
Product(s) whose prices are less than 100:
Product A
Product D
Further reading:
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- 2 Ways to Get a Random Item from a List in Dart (and Flutter)
- Dart: Converting a List to a Map and Vice Versa
- Flutter: Convert UTC Time to Local Time and Vice Versa
- Dart: Convert Timestamp to DateTime and vice versa
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.