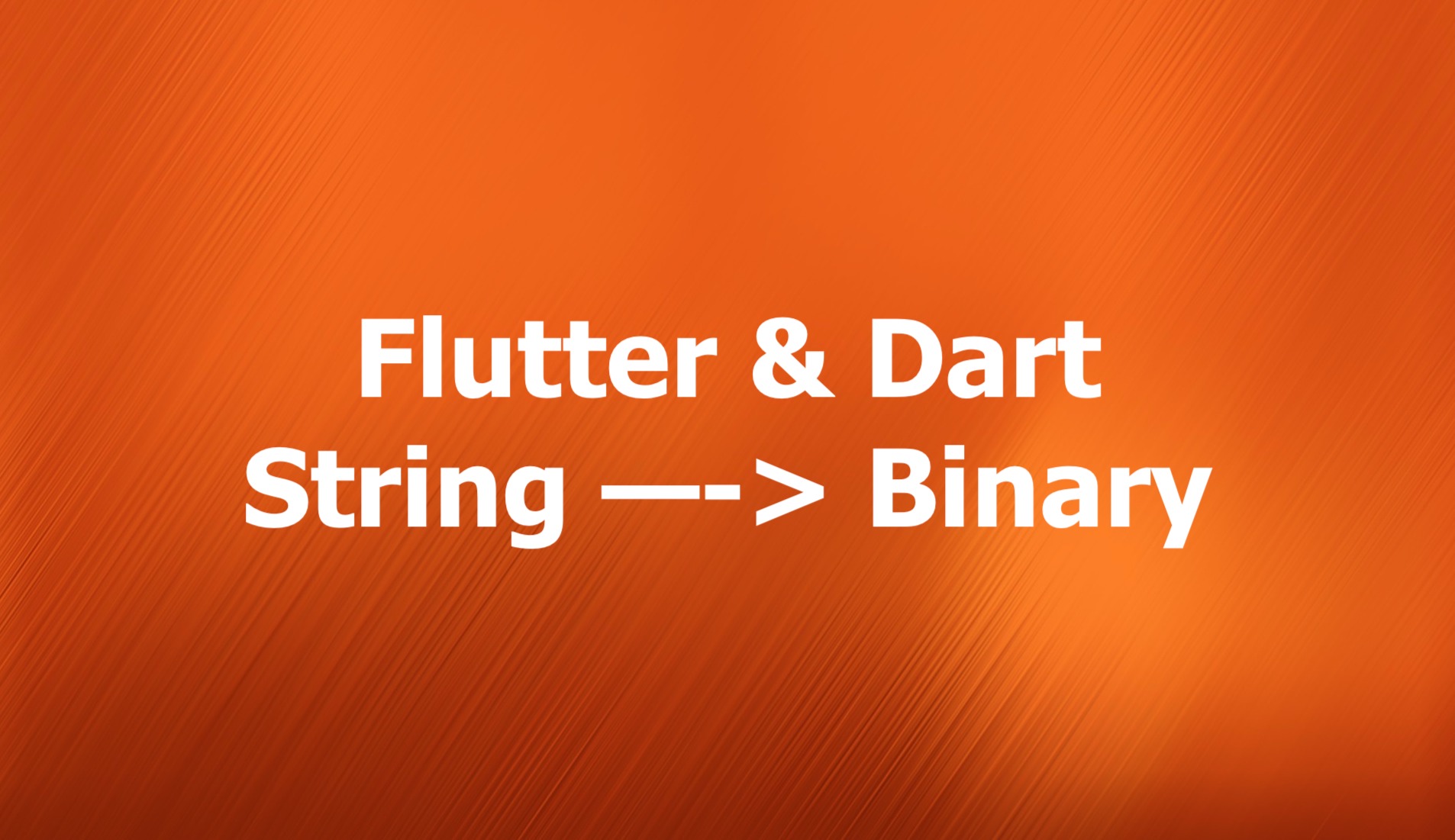
Binary is made up of 0s and 1s, arranged in a sequence, and can be of any length. This quick article will show you how to convert a given string into binary in Dart (and Flutter as well).
The steps:
- Declare a function named stringToBinary that takes a string parameter and returns a binary representation.
- Inside the function, create an empty list to store the binary representations of each character.
- Use a loop to iterate over each character in the input string.
- Get the ASCII value of the current character using the codeUnitAt() method.
- Convert the ASCII value to binary using the toRadixString() method with the radix value set to 2.
- Append the binary representation to the list.
- After the loop completes, join the binary representations in the list using a space separator.
- Return the joined binary string.
Code example:
// KindaCode.com
import 'package:flutter/foundation.dart';
String stringToBinary(String input) {
List<String> binaryList = [];
for (int i = 0; i < input.length; i++) {
int charCode = input.codeUnitAt(i);
String binary = charCode.toRadixString(2);
binaryList.add(binary);
}
return binaryList.join(' ');
}
void main() {
String input = "KindaCode.com";
String binaryString = stringToBinary(input);
debugPrint(binaryString);
}
Output:
1001011 1101001 1101110 1100100 1100001 1000011 1101111 1100100 1100101 101110 1100011 1101111 1101101
That’s it. Further reading:
- Flutter: 2 Ways to Create an Onboarding Flow (Intro Slider)
- Flutter & Dart: Sorting a List of Objects/Maps by Date
- How to Reverse a String in Dart (3 Approaches)
- Working with the Align widget in Flutter
- Flutter: Reading Bytes from a Network Image
- Flutter TextField: Styling labelText, hintText, and errorText
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.