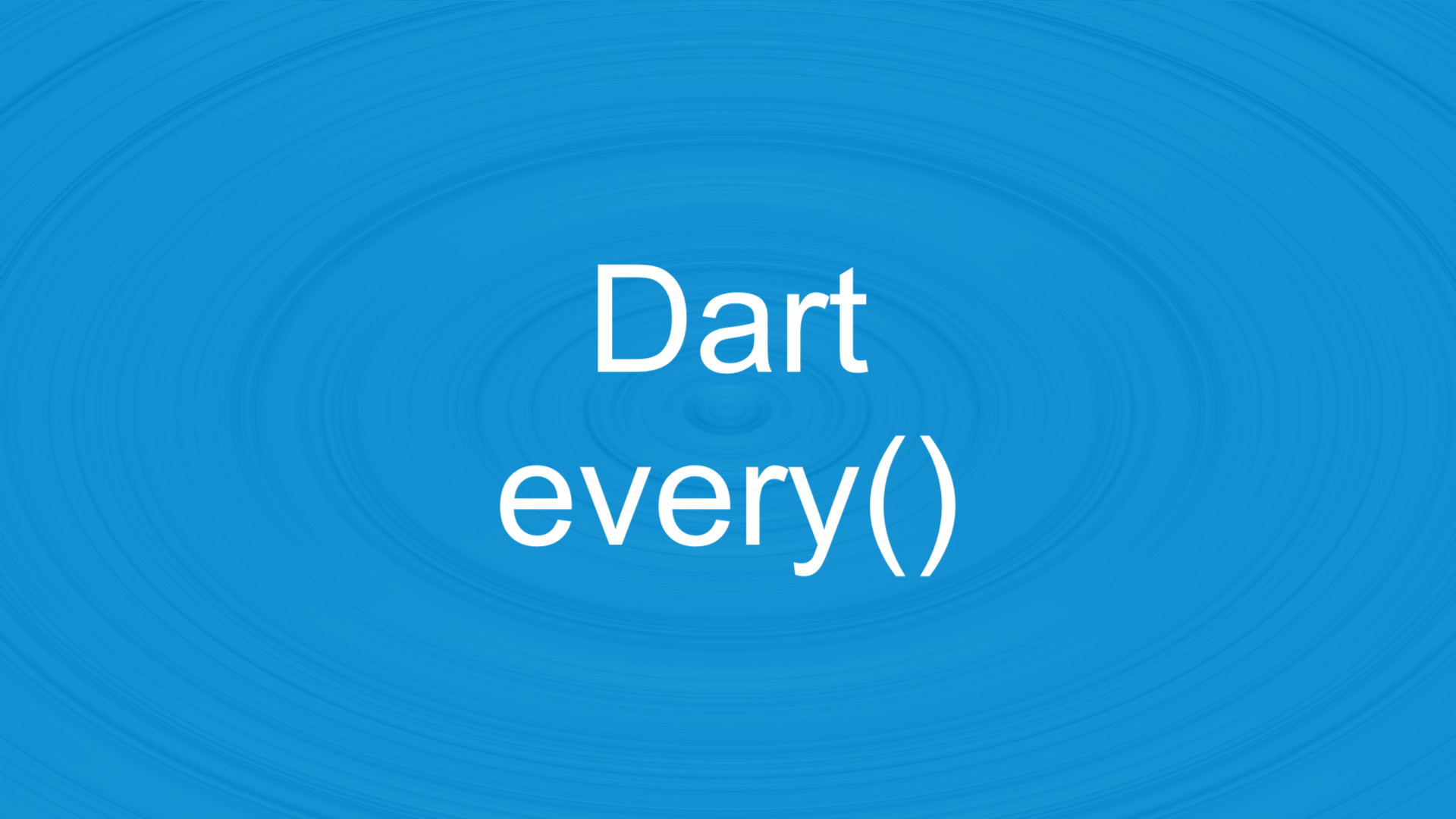
A few examples of using the every() method (of the Iterable class) in Dart (and Flutter as well). The purpose of this method is to check if every element of a given iterable satisfies one or multiple conditions (with a test function):
bool every(
bool test(
E element
)
)
Check whether every number in a list is exactly divisible by 3
The code:
void main() {
var myList = [0, 3, 6, 9, 18, 21, 81, 120];
bool result1 = myList.every((element){
if(element %3 ==0){
return true;
} else {
return false;
}
});
// expectation: true
print(result1);
}
Output:
true
Check whether every name in the list contains the “m” character
The code:
void main() {
var names = ['Obama', 'Trump', 'Biden', 'Pompeo'];
bool hasM = names.every((element) {
if(element.contains('m')) return true;
return false;
});
print(hasM);
}
Output:
false
You can find more details about the every() method in the official docs.
Further reading:
- How to Flatten a Nested List in Dart
- Using Cascade Notation in Dart and Flutter
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- Dart: Checking if a Map contains a given Key/Value
- Dart: Find List Elements that Satisfy Conditions
- How to Check if a Variable is Null in Flutter and Dart
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.