This article shows you how to retrieve the name and extension of a file from a file path or a web URL in Dart (and Flutter as well).
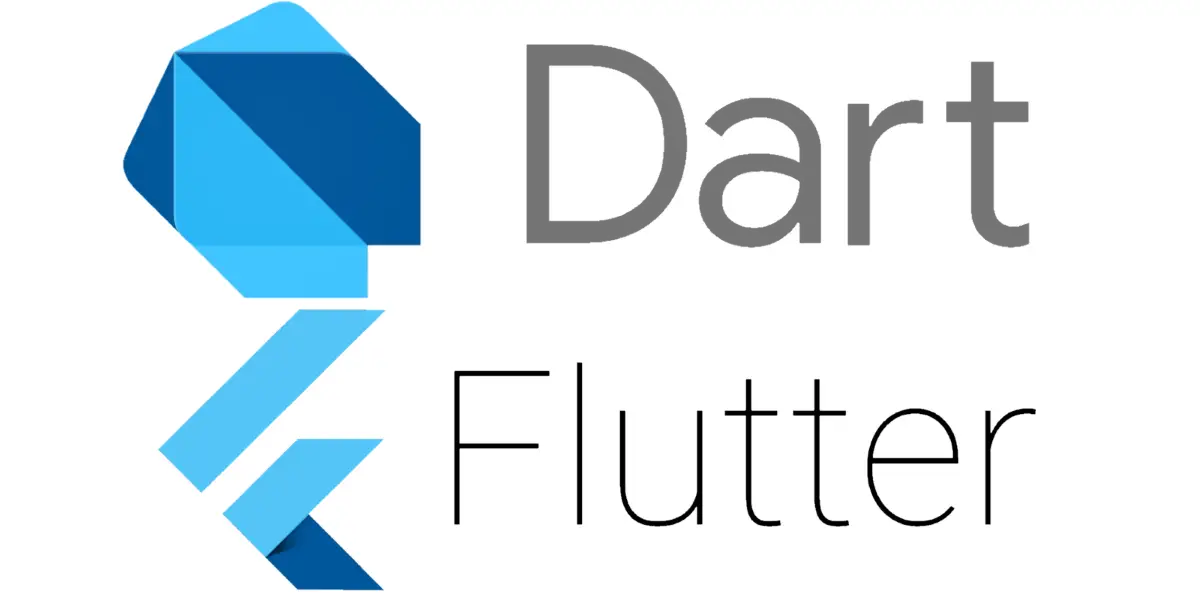
In order to get the job done, we need the dart:io library and the path package (this one is an official plugin published by the Dart team).
Install the latest version of the path package by executing the following command:
flutter pub add path
Then run:
flutter pub get
No special permission is required.
The path package provides 3 useful functions that can help us get the filename or extension with ease:
- basename(): Returns the file name with the extension
- extension(): Returns the file extension
- basenameWithoutExtension(): Returns the file name without extension
Example
The code:
// KindaCode.com
// main.dart
import 'dart:io';
import 'package:path/path.dart';
import 'package:flutter/foundation.dart';
void main() {
// File Path
const examplePath = '/my-secret/some-path/abcxyz/my-book.pdf';
final File file = File(examplePath);
final filename = basename(file.path);
final fileExtension = extension(file.path);
final nameWithoutExtension = basenameWithoutExtension(file.path);
debugPrint('Filename: $filename');
debugPrint('Filename without extension: $nameWithoutExtension');
debugPrint('Extension: $fileExtension');
}
Output:
Filename: my-book.pdf
Filename without extension: my-book
Extension: .pdf
You can get the same result with a file from the internet with its URL instead of a local path:
// KindaCode.com
// main.dart
import 'dart:io';
import 'package:path/path.dart';
import 'package:flutter/foundation.dart';
void main() {
// File URL from the internet
const exampleUrl = 'https://www.kindacode.com/some-directory/my-photo.jpg';
final File file = File(exampleUrl);
final filename = basename(file.path);
final nameWithoutExtension = basenameWithoutExtension(file.path);
final fileExtenion = extension(file.path);
debugPrint('Filename: $filename');
debugPrint('Filename without extension: $nameWithoutExtension');
debugPrint('Extension: $fileExtenion');
}
Output:
Filename: my-photo.jpg
Filename without extension: my-photo
Extension: .jpg
Conclusion
You’ve learned how to retrieve the name and extension of a file from a path or web URL. Continue learning more about Flutter and Dart by taking a look at the following articles:
- Flutter StreamBuilder examples
- Dart: Extract a substring from a given string (advanced)
- Working with Cupertino Date Picker in Flutter
- Flutter and Firestore Database: CRUD example
- Remove duplicate items from a list in Dart
- Working with dynamic Checkboxes in Flutter
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.