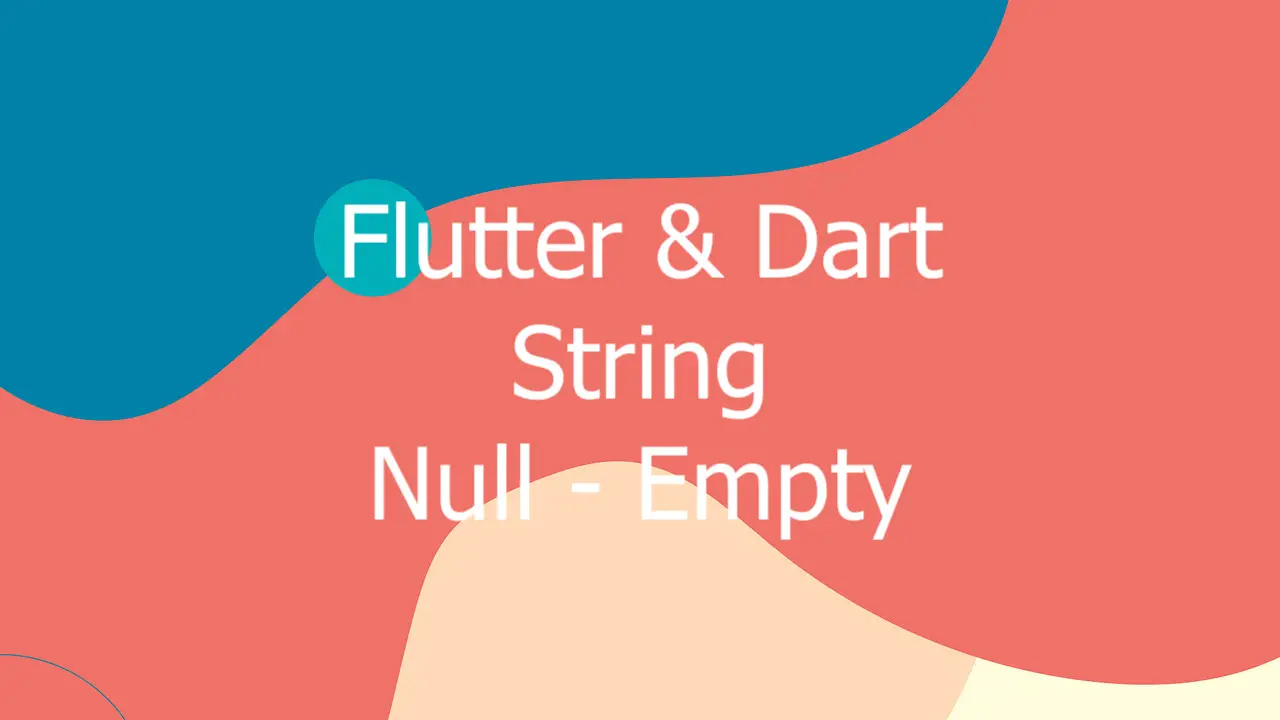
When working with Flutter and Dart, there might be cases where you have to check whether a given string is null or empty. We can define a reusable function to do the job as follows:
bool validateInput(String? input) {
if (input == null) {
return false;
}
if (input.isEmpty) {
return false;
}
return true;
}
We can shorten the function like this:
bool validateInput(String? input) {
return input?.isNotEmpty ?? false;
}
The function will return false if the input is null or empty. It will return true if this string contains at least one character. Let’s give it a shot:
// main.dart
bool validateInput(String? input) {
return input?.isNotEmpty ?? false;
}
void main() {
print(validateInput(''));
print(validateInput('abc'));
print(validateInput(null));
}
Output:
false
true
false
That’s it. Further reading:
- 3 Ways to Cancel a Future in Flutter and Dart
- Dart: Converting a List to a Set and vice versa
- Sorting Lists in Dart and Flutter (5 Examples)
- Flutter and Firestore Database: CRUD example
- Flutter & Hive Database: CRUD Example
- Using GetX (Get) for State Management in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.