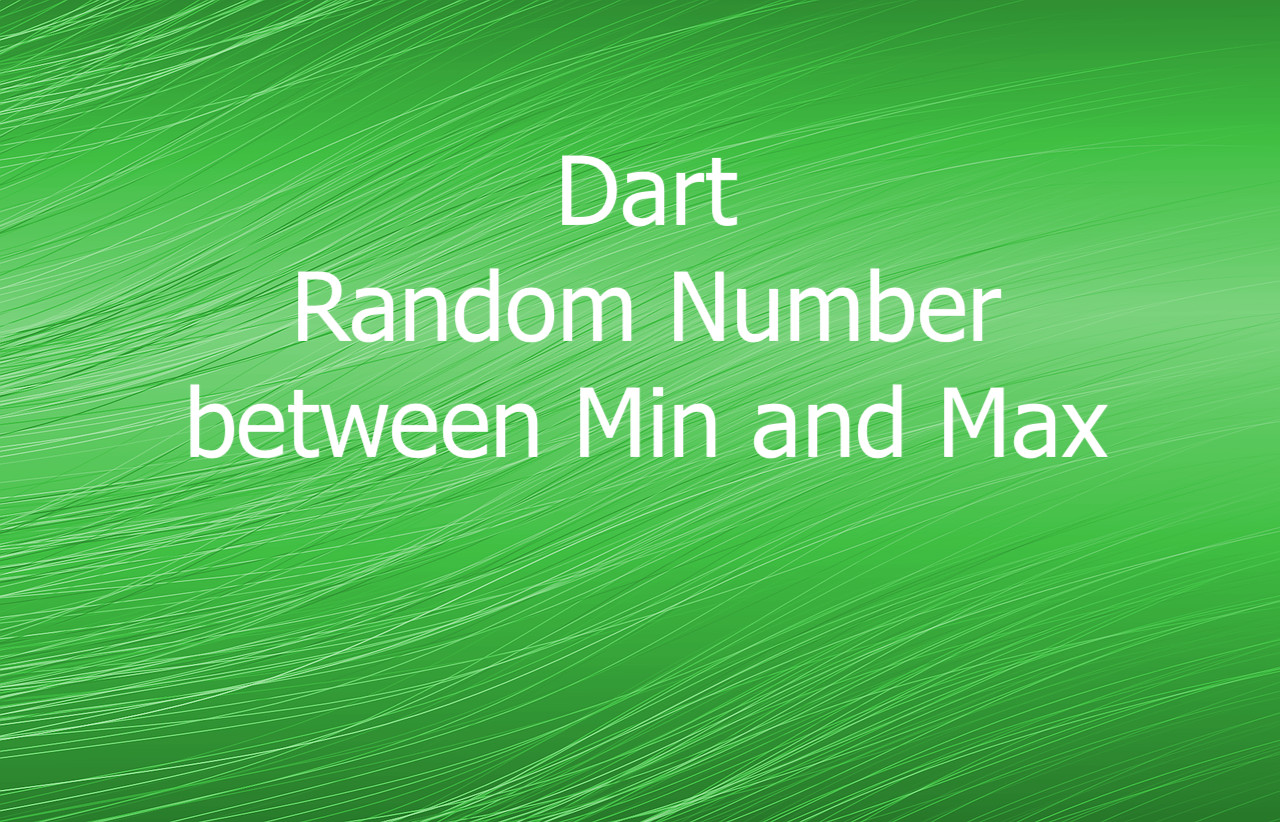
A couple of examples of generating a random integer within a given range in Dart (and Flutter as well).
Example 1: Using Random().nextInt() method
The code:
// KindaCode.com
import 'dart:math';
import 'package:flutter/foundation.dart';
// define a reusable function
randomGen(min, max) {
// the nextInt method generate a non-ngegative random integer from 0 (inclusive) to max (exclusive)
var x = Random().nextInt(max) + min;
// If you don't want to return an integer, just remove the floor() method
return x.floor();
}
void main() {
int a = randomGen(-99, 99);
debugPrint(a.toString());
}
Output:
-94
Due to the randomness, it’s very likely that you’ll get a different result each time you execute the code.
Example 2: Using Random.nextDouble() and floor() methods
The code:
// KindaCode.com
import 'dart:math';
import 'package:flutter/foundation.dart';
randomGen(min, max) {
// the nextDouble() method returns a random number between 0 (inclusive) and 1 (exclusive)
var x = Random().nextDouble() * (max - min) + min;
// If you don't want to return an integer, just remove the floor() method
return x.floor();
}
// Testing
void main() {
if (kDebugMode) {
// with posstive min and max
print(randomGen(10, 100));
// with negative min
print(randomGen(-100, 0));
}
}
Output (the output, of course, is random and will change each time you re-execute your code).
47
-69
The result you get will probably contain min but never contain max.
What’s Next?
Continue learning more about Flutter and Dart by taking a look at the following articles:
- Dart: Convert Map to Query String and vice versa
- Dart: Sorting Entries of a Map by Its Values
- Sorting Lists in Dart and Flutter
- 2 Ways to Create Multi-Line Strings in Dart
- How to remove items from a list in Dart
- Dart regex to validate US/CA phone numbers
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.