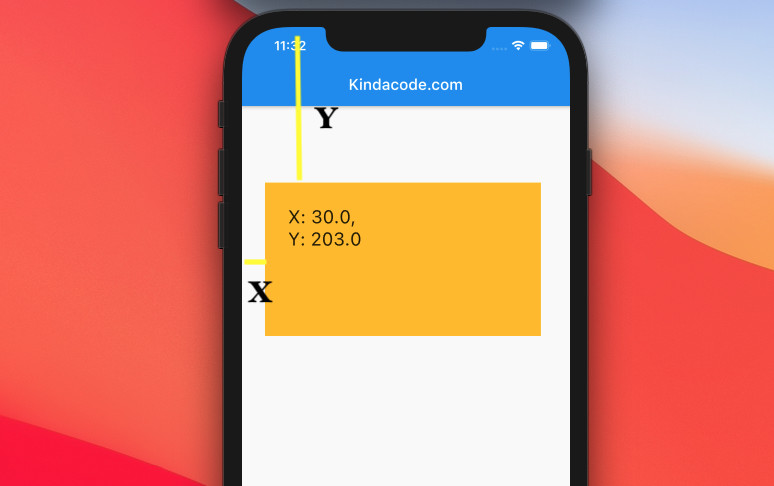
There may be times you want to calculate the X and Y positions of a widget in your Flutter application, for instance, programmatically scrolling to a particular destination when the user presses a tab or a button, etc.
- X: The left position (in pixels) relative to the left of the screen.
- Y offset: The top position (in pixels) relative to the top of the screen.
To get the exact position of a widget, we need to give it a key. To make it easier to understand, see the following example.
Table of Contents
Example
App Preview
This sample app contains an amber box and a floating button. Once the user presses the button, we’ll calculate and then display the X and Y coordinates of the box on the screen.
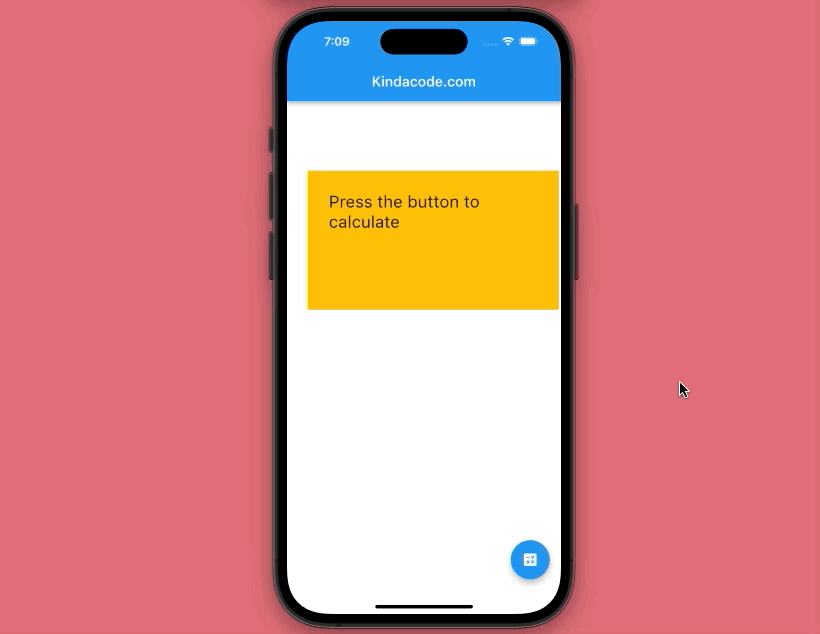
The Code
The complete code in main.dart (with explanations):
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// This key will be associated with the amber box
final GlobalKey _key = GlobalKey();
// Coordinates
double? _x, _y;
// This function is called when the user presses the floating button
void _getOffset(GlobalKey key) {
RenderBox? box = key.currentContext?.findRenderObject() as RenderBox?;
Offset? position = box?.localToGlobal(Offset.zero);
if (position != null) {
setState(() {
_x = position.dx;
_y = position.dy;
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Stack(children: [
Positioned(
top: 100,
left: 30,
// The amber box
child: Container(
key: _key,
width: 360,
height: 200,
padding: const EdgeInsets.all(30),
color: Colors.amber,
child: Text(
_x != null
? "X: $_x, \nY: $_y"
: 'Press the button to calculate',
style: const TextStyle(fontSize: 24),
),
),
),
]),
),
floatingActionButton: FloatingActionButton(
onPressed: () => _getOffset(_key),
child: const Icon(Icons.calculate)),
);
}
}
Conclusion
You’ve learned the technique to get the X and Y coordinates of a widget in Flutter. If you would like to explore more about the SDK, read also the following articles:
- Flutter: Scrolling to a desired Item in a ListView
- How to create a zebra striped ListView in Flutter
- How to make an image carousel in Flutter
- How to implement Star Rating in Flutter
- Using Stack and IndexedStack in Flutter
- Programmatically show/hide a widget using Visibility
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.