In this article, we will go over a few examples of using the FittedBox widget in Flutter.
Example 1: Prevent Text from being invisible with FittedBox
The text’s font size is reduced to fit its parent.
Screenshots
In the left screenshot, we don’t use the FittedBox, and in the right screenshot, we do.
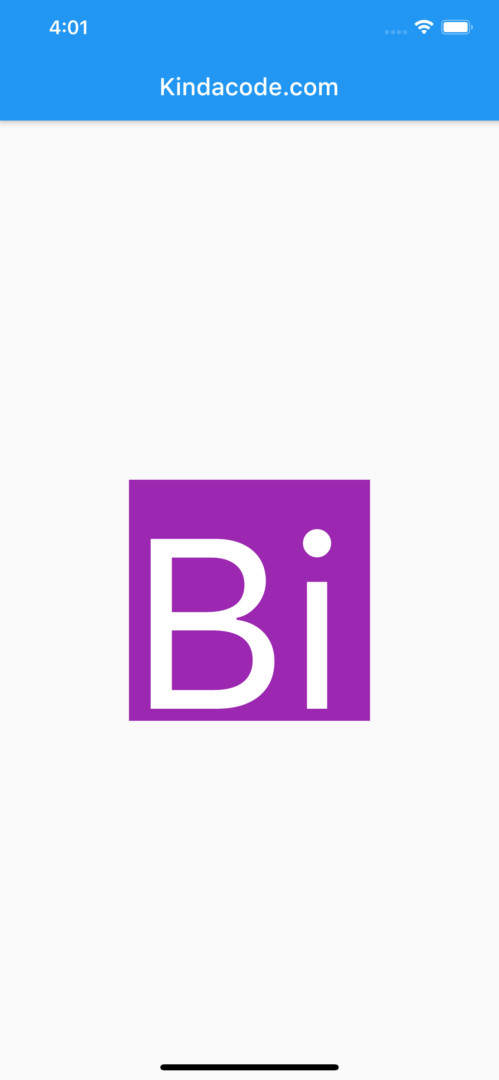
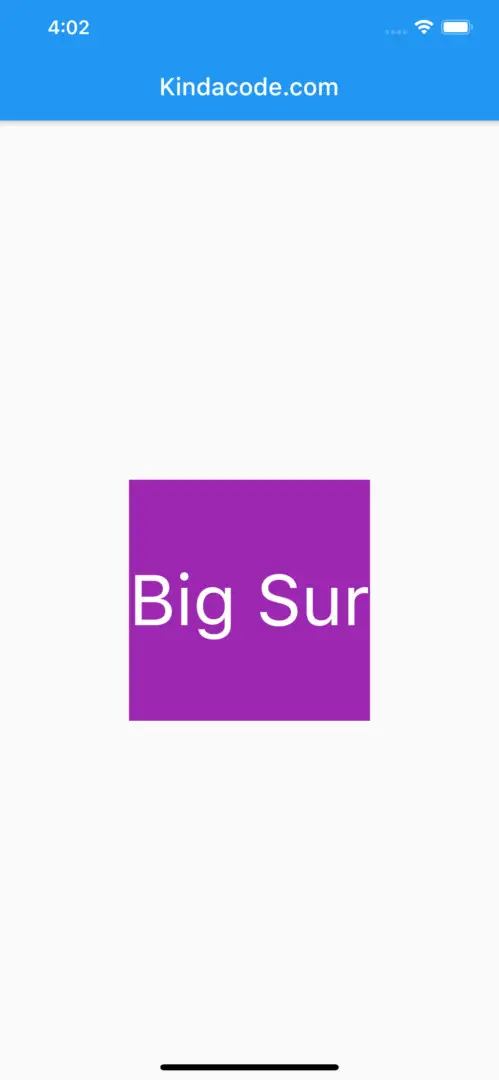
Code snippets:
// No FittedBox
Center(
child: Container(
width: 200,
height: 200,
color: Colors.purple,
child: const Text(
'Big Sur',
style: TextStyle(fontSize: 200, color: Colors.white),
),
),
)
// With FittedBox
Center(
child: Container(
width: 200,
height: 200,
color: Colors.purple,
child: FittedBox(
fit: BoxFit.contain,
child: const Text(
'Big Sur',
style: TextStyle(fontSize: 200, color: Colors.white),
),
),
),
)
Example 2: BoxFit.cover, BoxFit.contain, and BoxFit.fill
Screenshots:
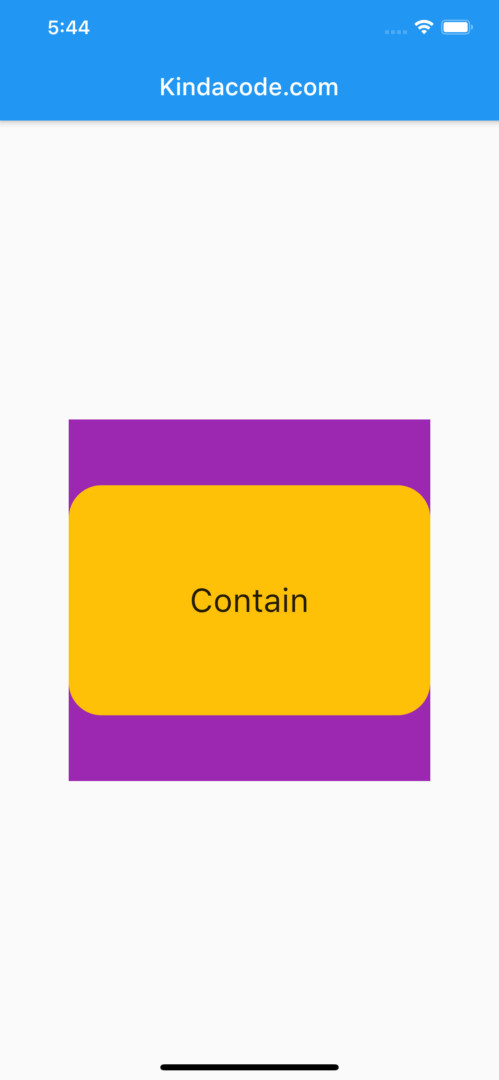
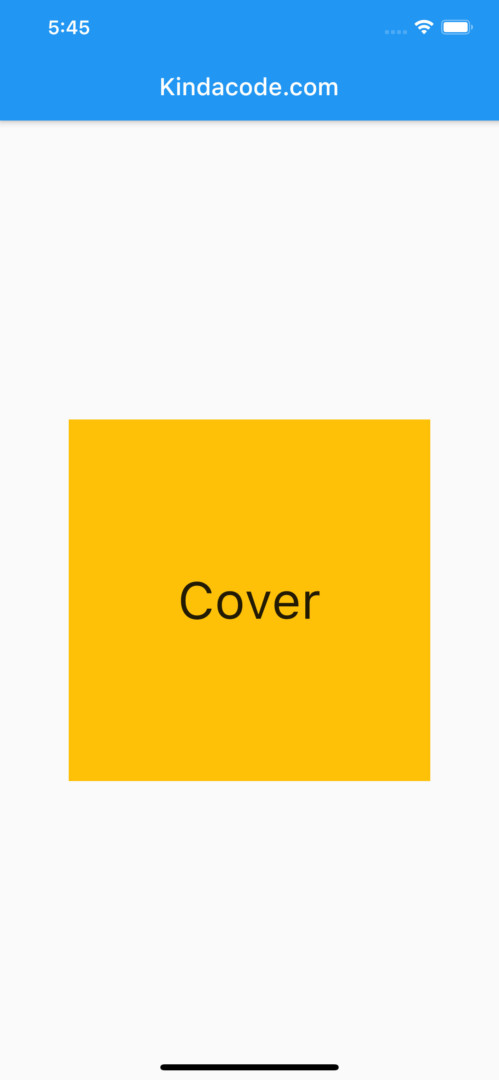
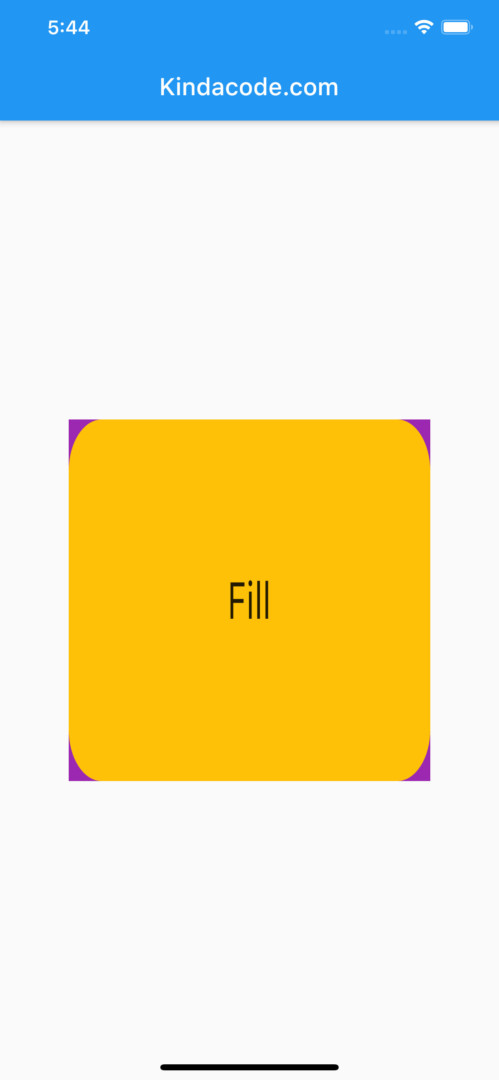
Contain: The child is as large as possible while still containing the source entirely within the target box.
Container(
width: 300,
height: 300,
color: Colors.purple,
child: FittedBox(
fit: BoxFit.contain,
child: Container(
width: 550,
height: 350,
decoration: BoxDecoration(
color: Colors.amber,
borderRadius: BorderRadius.circular(50)),
alignment: Alignment.center,
child: const Text(
'Contain',
style: TextStyle(fontSize: 50),
),
),
Cover: The child is as small as possible while still covering the entire target box.
Container(
width: 300,
height: 300,
color: Colors.purple,
child: FittedBox(
fit: BoxFit.cover,
child: Container(
width: 550,
height: 350,
decoration: BoxDecoration(
color: Colors.amber,
borderRadius: BorderRadius.circular(50)),
alignment: Alignment.center,
child: const Text(
'Cover',
style: TextStyle(fontSize: 50),
),
),
),
),
Fill: The child fills the target box by distorting the source’s aspect ratio.
Container(
width: 300,
height: 300,
color: Colors.purple,
child: FittedBox(
fit: BoxFit.fill,
child: Container(
width: 550,
height: 350,
decoration: BoxDecoration(
color: Colors.amber,
borderRadius: BorderRadius.circular(50)),
alignment: Alignment.center,
child: const Text(
'Fill',
style: TextStyle(fontSize: 50),
),
),
),
),
Conclusion
We’ve gone through a couple of examples about using the FittedBox widget to automatically scale and position its child to fit. If you’d like to explore more new and interesting things in modern mobile development with Flutter, take a look at the following articles:
- Using Chip widget in Flutter: Tutorial & Examples
- Styling Text in Flutter: Tutorial & Examples
- Using ErrorWidget in Flutter
- How to make an image carousel in Flutter
- Flutter: Showing a badge on the Top Right of a widget
- How to implement Star Rating in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.