This article gives you a practical Flutter example of implementing FractionallySizedBox, a widget that sizes its child to a fraction of the total available space. Here’s its constructor:
FractionallySizedBox({
Key? key,
AlignmentGeometry alignment = Alignment.center,
double? widthFactor,
double? heightFactor,
Widget? child
})
Example
This sample app contains a yellow box with a height of 500 and a width of double.infinity. The child of the yellow box is a FractionallySizedBox whose child is a purple rectangular. The width of the purple one is 80% of the width of the yellow box, and its height is 30% of the yellow box.
Screenshot:
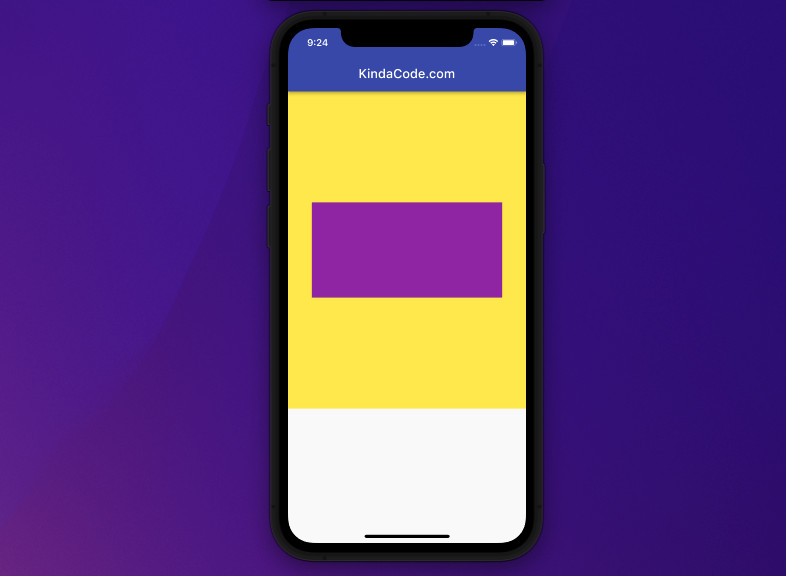
The code (for the yellow box and its descendants):
Container(
width: double.infinity,
height: 500,
color: Colors.yellow,
// implement FractionallySizedBox
child: FractionallySizedBox(
widthFactor: 0.8,
heightFactor: 0.3,
alignment: Alignment.center,
// the purple box
child: Container(
color: Colors.purple,
),
),
)
The full source code in main.dart (with boilerplate):
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(primarySwatch: Colors.indigo),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
backgroundColor: Colors.indigo,
),
// this Container constructs the yellow box
body: Container(
width: double.infinity,
height: 500,
color: Colors.yellow,
// implement FractionallySizedBox
child: FractionallySizedBox(
widthFactor: 0.8,
heightFactor: 0.3,
alignment: Alignment.center,
// the purple box
child: Container(
color: Colors.purple,
),
),
));
}
}
That’s it. Further reading:
- Flutter: SliverGrid example
- Create a Custom NumPad (Number Keyboard) in Flutter
- Flutter: GridPaper example
- Flutter: Creating Custom Back Buttons
- Best Libraries for Making HTTP Requests in Flutter
- Flutter: Creating an Auto-Resize TextField
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.