Knowing the screen width and height can be useful in several scenarios while developing mobile apps. Some of the common use cases include:
- Responsive Design: By knowing the screen size, developers can create a responsive design that adjusts to the size of the screen, ensuring that the content is displayed optimally on different devices.
- Optimizing UI elements: By knowing the screen size, developers can adjust the size of buttons, text, and other UI elements to ensure that they are easy to use and accessible on all devices.
- Image optimization: Based on the screen size, developers can choose to load different versions of images that are optimized for a specific screen size, reducing the overall size of the app and improving performance.
In Flutter, you can get the device screen width by using:
MediaQuery.of(context).size.width;
To get the screen height, use:
MediaQuery.of(context).size.height;
Example
Screenshot:
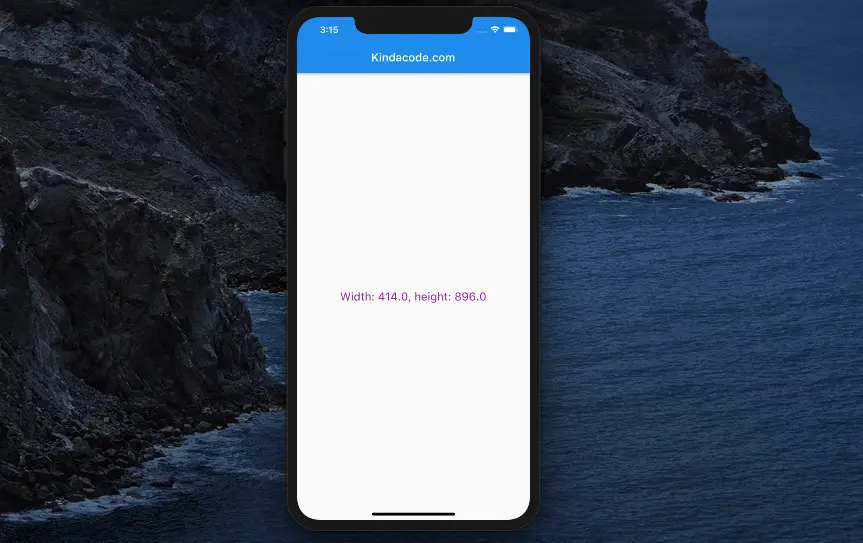
Full code in main.dart:
// KindaCode.com
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
final width = MediaQuery.of(context).size.width;
final height = MediaQuery.of(context).size.height;
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Text(
"Width: $width, height: $height",
style: const TextStyle(fontSize: 20, color: Colors.purple),
),
),
);
}
}
Hope this helps. Futher reading:
- Flutter: Columns with Percentage Widths
- Using IntrinsicWidth in Flutter: Example & Explanation
- How to set width, height, and padding of TextField in Flutter
- Flutter: Get the Heights of AppBar and CupertinoNavigationBar
- Flutter: Changing App Display Name for Android & iOS
- Android: How to Get SHA Certificate Fingerprints
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.