To get the device operating system version that your Flutter app is running on, just import dart:io and use Platform.operatingSystemVersion. No third-party package or installation is required.
A Quick Example
import 'dart:io';
void main() {
// Detect the OS
print(Platform.operatingSystem);
// The OS version
print(Platform.operatingSystemVersion);
}
A Complete Example
Complete code in main.dart:
import 'package:flutter/material.dart';
import 'dart:io';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Padding(
padding: const EdgeInsets.all(30),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
// Display the platform
Text(
'Platform: ${Platform.operatingSystem}',
style: const TextStyle(fontSize: 25),
),
// Display the version
Text(
'Version: ${Platform.operatingSystemVersion}',
style: const TextStyle(fontSize: 25),
)
],
),
),
);
}
}
When running the code with your iOS simulator and Android emulator, you will get something like this (the platforms should be iOS and Android, but the versions may be different):
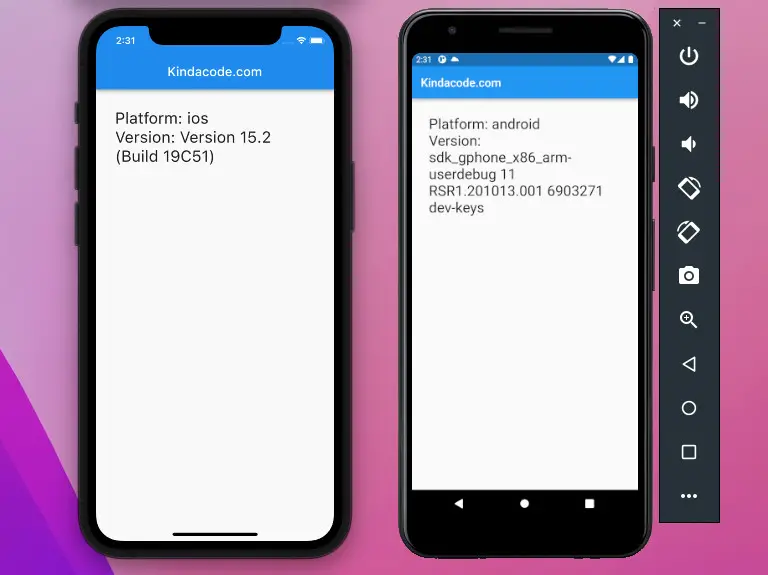
That’s it. Have a nice day. Further reading:
- Flutter: Convert UTC Time to Local Time and Vice Versa
- Flutter: Hide the AppBar in landscape mode
- Using Chip widget in Flutter: Tutorial & Examples
- Flutter & Hive Database: CRUD Example
- Flutter and Firestore Database: CRUD example
- How to Check if a Variable is Null in Flutter and Dart
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.