This concise and straightforward article shows you how to programmatically determine the width and height of a network image in Flutter.
Table of Contents
What is the point?
The steps to get the size of a network image are as follows:
1. Read bytes from the image URL (as Uint8List):
final ByteData data =
await NetworkAssetBundle(Uri.parse(imageUrl)).load(imageUrl);
final Uint8List bytes = data.buffer.asUint8List();
2. Decode the received bytes to get the image dimensions:
final decodedImage = await decodeImageFromList(bytes);
print(decodedImage.width);
print(decodedImage.height);
If you are still confused by this, just move on to the next section and see an end-to-end beginner-friendly working example.
Complete example
Preview
In this example, we will determine the width and height of a network image and then display them on the screen as follows:
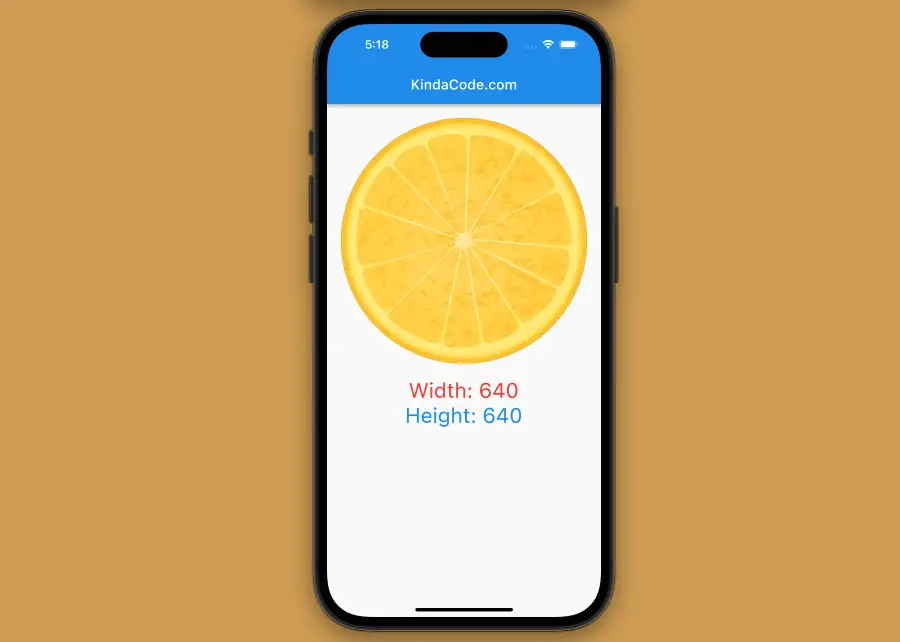
The code
The full source code with explanations:
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
// hide the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
int? _width;
int? _height;
// Reading bytes from a network image
Future<void> getImageSize(String imageUrl) async {
// get the bytes from the image
final ByteData data =
await NetworkAssetBundle(Uri.parse(imageUrl)).load(imageUrl);
final Uint8List bytes = data.buffer.asUint8List();
// decode the bytes to get the image size
final decodedImage = await decodeImageFromList(bytes);
// print the image size
print(decodedImage.width);
print(decodedImage.height);
// set the state
setState(() {
_width = decodedImage.width;
_height = decodedImage.height;
});
}
// Example image URL
final exampleImageUrl =
'https://www.kindacode.com/wp-content/uploads/2022/07/lemon.png';
@override
initState() {
super.initState();
// call the "getImageSize" function
getImageSize(exampleImageUrl);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text("KindaCode.com")),
body: Padding(
padding: const EdgeInsets.all(20),
child: Column(
children: [
Image.network(exampleImageUrl),
const SizedBox(height: 20),
// Display the size of the image
Text("Width: $_width",
style: const TextStyle(fontSize: 30, color: Colors.red)),
Text("Height: $_height",
style: const TextStyle(fontSize: 30, color: Colors.blue)),
],
),
));
}
}
What is next?
You’ve learned how to get the size of an arbitrary network image with a few lines of Dart code. There’s no need to install any third-party libraries for this task. If you’d like to explore more new and interesting stuff about modern Flutter development, take a look at the following articles:
- Flutter & Dart: Sorting a List of Objects/Maps by Date
- How to Create a Stopwatch in Flutter
- Flutter & Hive Database: CRUD Example
- Flutter & Dart: Sorting a List of Objects/Maps by Date
- Flutter: ValueListenableBuilder Example
- Flutter: How to Read and Write Text Files
- Flutter reorderableListView example
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.