This concise, practical article will walk you through three complete examples of creating gradient text in Flutter. We’ll use only the built-in features of Flutter and won’t rely on any third-party libraries.
Example 1: Linear Gradient Text (with Shader)
Screenshot:
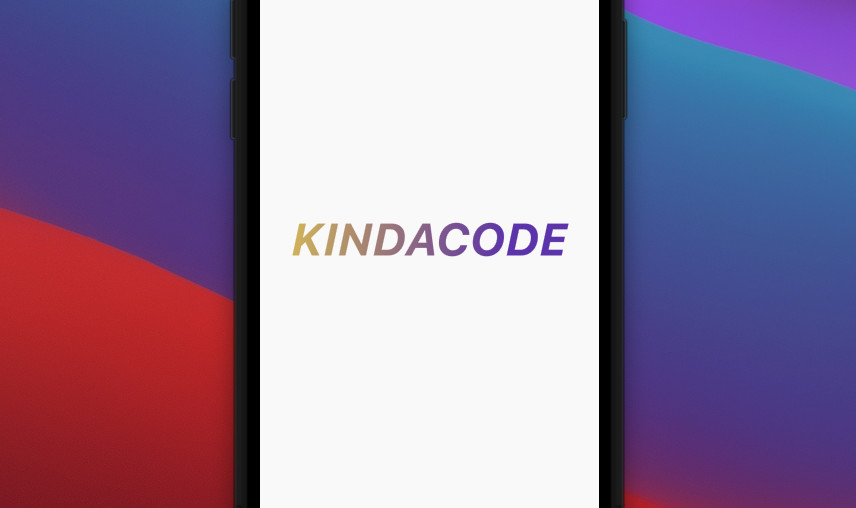
The full code (with explanations):
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage());
}
}
class HomePage extends StatelessWidget {
// Creates a Shader for this gradient to fill the given rect
final Shader _linearGradient = const LinearGradient(
colors: [Colors.yellow, Colors.deepPurple],
begin: Alignment.centerLeft,
end: Alignment.bottomRight,
).createShader(const Rect.fromLTWH(0.0, 0.0, 320.0, 80.0));
HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Text(
'KINDACODE',
style: TextStyle(
fontSize: 50,
fontStyle: FontStyle.italic,
fontWeight: FontWeight.bold,
foreground: Paint()..shader = _linearGradient),
),
),
);
}
}
Example 2: Linear Gradient Text (with ShaderMask)
Screenshot:
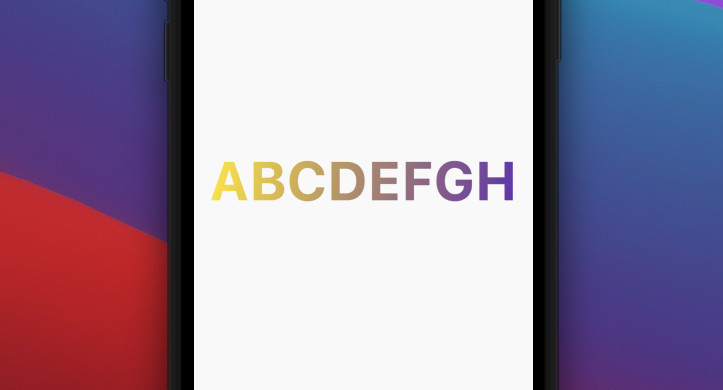
The code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage());
}
}
class HomePage extends StatelessWidget {
// Create a linear gradient
final Gradient _gradient = const LinearGradient(
colors: [Colors.yellow, Colors.deepPurple],
begin: Alignment.topLeft,
end: Alignment.bottomRight,
);
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
// Use ShaderMask to apply the gradient as the text color
child: ShaderMask(
blendMode: BlendMode.modulate,
shaderCallback: (size) => _gradient.createShader(
Rect.fromLTWH(0, 0, size.width, size.height),
),
child: const Text(
'ABCDEFGH',
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
fontSize: 60,
),
),
),
),
);
}
}
Example 3: Radial Gradient Text (with ShaderMask)
Screenshot:
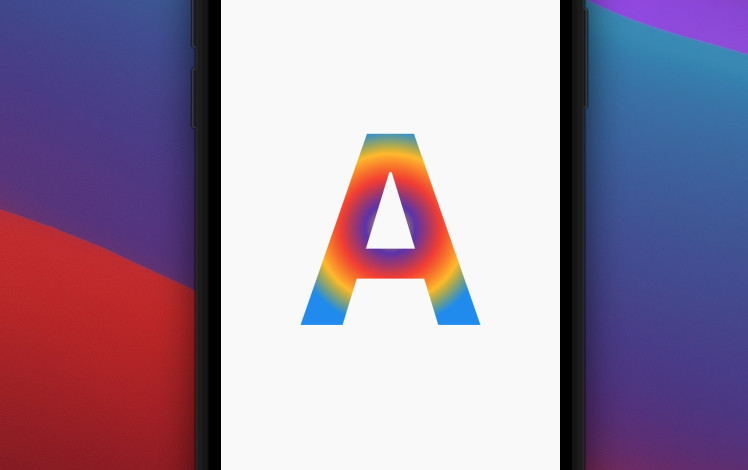
The code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage());
}
}
class HomePage extends StatelessWidget {
// Create a RadialGradient object
final Gradient _gradient = const RadialGradient(
colors: [
Colors.yellow,
Colors.deepPurple,
Colors.red,
Colors.amber,
Colors.blue
],
center: Alignment.center,
);
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
// Use ShaderMask to apply the gradient as a mask
child: ShaderMask(
blendMode: BlendMode.modulate,
shaderCallback: (size) => _gradient.createShader(
Rect.fromLTWH(0, 0, size.width, size.height),
),
child: const Text(
'A',
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
fontSize: 300,
),
),
),
),
);
}
}
Wrapping Up
We’ve gone through some examples of making beautiful gradient text in Flutter. Continue learning and exploring more by taking a look at the following:
- Flutter & SQLite: CRUD Example
- How to create selectable text in Flutter
- Flutter: ValueListenableBuilder Example
- Flutter Vertical Text Direction: An Example
- Flutter: Dynamic Text Color Based on Background Brightness
- A Complete Guide to Underlining Text in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.