This article walks you through a few examples of using the GridTile widget in Flutter applications.
Overview
GridTile helps us quickly and easily create a tile with rich content (a combination of text, images, and icons). This widget is often used with the GridView widget, but it can be used as a standalone component.
Constructor:
GridTile({
Key? key,
Widget? header,
Widget? footer,
required Widget child
})
If you want to make a one-line or two-line header/footer, you can use the GridTileBar widget:
GridTileBar({
Key? key,
Color? backgroundColor,
Widget? leading,
Widget? title,
Widget? subtitle,
Widget? trailing
})
Now it’s time to write some code.
Examples
Social Network Photo Frame
This example creates a social network photo post frame (like what you can see on Instagram or many other places).
Screenshot:
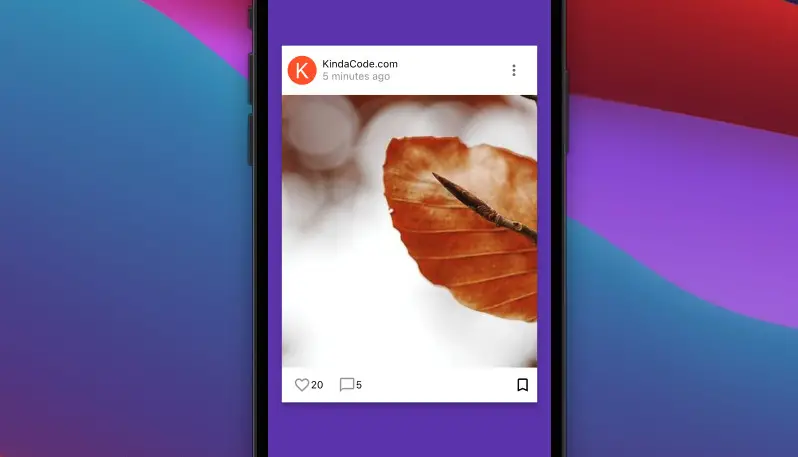
The code:
Scaffold(
backgroundColor: Colors.deepPurple,
body: Center(
child: SizedBox(
width: 360,
height: 500,
child: Card(
elevation: 6,
child: GridTile(
// header section
header: GridTileBar(
backgroundColor: Colors.white,
leading: const CircleAvatar(
backgroundColor: Colors.deepOrange,
child: Text(
'K',
style: TextStyle(color: Colors.white, fontSize: 30),
),
),
title: const Text(
'KindaCode.com',
style: TextStyle(color: Colors.black),
),
subtitle: const Text('5 minutes ago',
style: TextStyle(color: Colors.grey)),
trailing: IconButton(
onPressed: () {},
icon: const Icon(
Icons.more_vert_rounded,
color: Colors.black54,
)),
),
// footer section
footer: GridTileBar(
backgroundColor: Colors.white,
title: Row(
children: const [
Icon(
Icons.favorite_outline,
color: Colors.grey,
),
Text('20', style: TextStyle(color: Colors.black)),
SizedBox(
width: 20,
),
Icon(
Icons.chat_bubble_outline,
color: Colors.grey,
),
Text(
'5',
style: TextStyle(color: Colors.black),
),
],
),
trailing: const Icon(
Icons.bookmark_outline,
color: Colors.black,
),
),
// main child
child: Image.network(
'https://www.kindacode.com/wp-content/uploads/2021/12/sample-leaf.jpeg',
fit: BoxFit.cover,
)),
),
),
),
);
Creating a Grid of Products
This example creates a grid view that displays some fictional products. You will likely see this in commerce apps.
App preview:
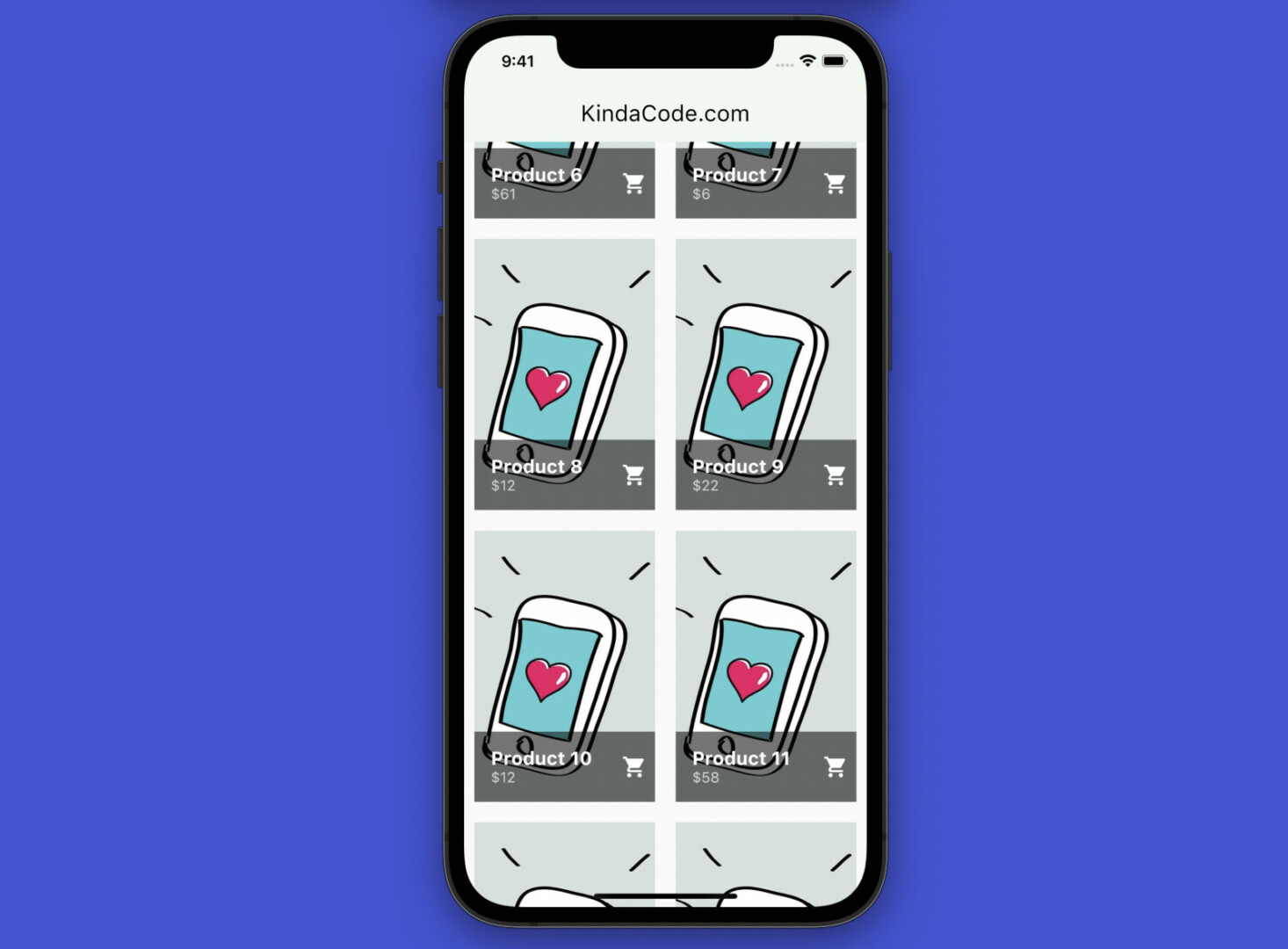
The complete code:
// main.dart
import 'package:flutter/material.dart';
import 'dart:math';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
// enable Material 3
useMaterial3: true,
primarySwatch: Colors.green,
),
home: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final List<Map> _products = List.generate(
100,
(index) => {
"id": index,
"name": "Product $index",
"price": Random().nextInt(100)
}).toList();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: GridView.builder(
padding: const EdgeInsets.all(10),
gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: 200,
childAspectRatio: 2 / 3,
crossAxisSpacing: 20,
mainAxisSpacing: 20),
itemCount: _products.length,
itemBuilder: (BuildContext ctx, index) {
return GridTile(
key: ValueKey(_products[index]['id']),
footer: GridTileBar(
backgroundColor: Colors.black54,
title: Text(
_products[index]['name'],
style: const TextStyle(
fontSize: 18, fontWeight: FontWeight.bold),
),
subtitle: Text("\$${_products[index]['price'].toString()}"),
trailing: const Icon(Icons.shopping_cart),
),
child: Image.network(
'https://www.kindacode.com/wp-content/uploads/2021/12/phone.jpeg',
fit: BoxFit.cover,
),
);
}),
);
}
}
Conclusion
You’ve learned the fundamentals of the GridTile widget and gone over a few examples of implementing it in practice. If you’d like to explore more new and awesome features of Flutter, take a look at the following articles:
- Flutter: SliverGrid example
- Creating Masonry Layout in Flutter with Staggered Grid View
- Flutter: Create a Password Strength Checker from Scratch
- Flutter: How to Draw a Heart with CustomPaint
- Using Static Methods in Dart and Flutter
- Adding a Border to Text in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.