In this example, we’ll create a simple Flutter app that has a checkbox and a button. By default, the checkbox is unchecked, and the button is disabled. When the checkbox is checked, the button is clickable.
The complete example
The demo:
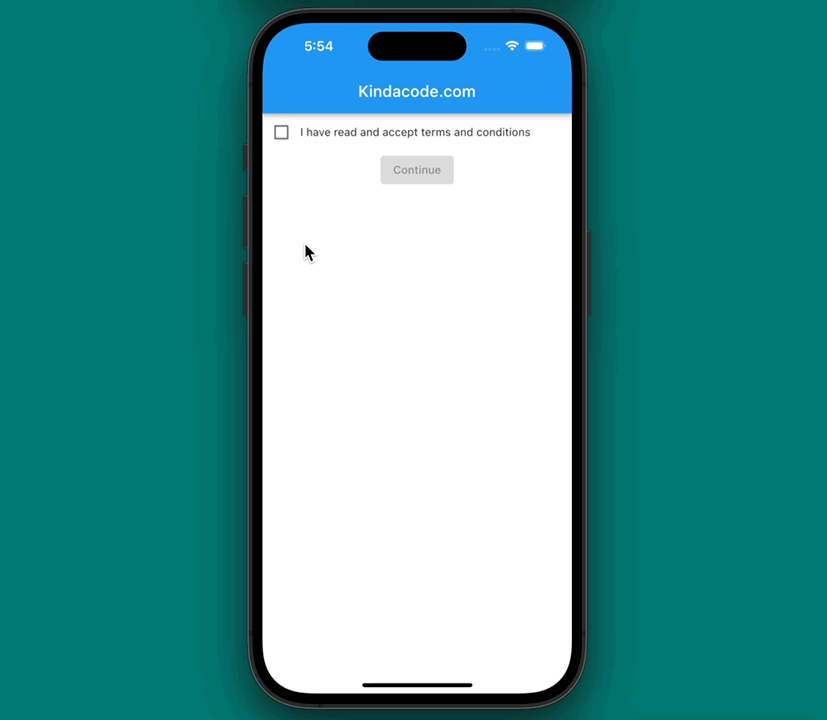
The full code in main.dart with explanations:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
// By defaut, the checkbox is unchecked and "agree" is "false"
bool agree = false;
// This function is triggered when the button is clicked
void _doSomething() {
// Do something
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Column(children: [
Row(
children: [
Material(
child: Checkbox(
value: agree,
onChanged: (value) {
setState(() {
agree = value ?? false;
});
},
),
),
const Text(
'I have read and accept terms and conditions',
overflow: TextOverflow.ellipsis,
)
],
),
ElevatedButton(
onPressed: agree ? _doSomething : null,
child: const Text('Continue'))
]),
);
}
}
Conclusion
Together we’ve built a simple app that demonstrates a common task in the real world: asking the user to agree to the terms of use before they can do something. If you’d like to explore more new and modern stuff of Flutter development, take a look at the following articles:
- Working with dynamic Checkboxes in Flutter
- Flutter: ExpansionPanelList and ExpansionPanelList.radio examples
- 2 Ways to Create Flipping Card Animation in Flutter
- Flutter: FilteringTextInputFormatter Examples
- Flutter: Caching Network Images for Big Performance gains
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.