In Dart and Flutter, if you want to import only one class from a file that contains multiple classes, just use the show keyword. The example below will help you understand this better.
Example
Let’s say our Flutter project has 2 files (all are in the lib folder): main.dart and test.dart. The test.dart file has 2 classes named FirstClass and SecondClass, but we only want to use FirstClass. Therefore, it makes sense if we only import FirstClass and ignore SecondClass, as shown below:
// main.dart
import './test.dart' show FirstClass;
Here’s the code for the test.dart file:
import 'package:flutter/material.dart';
// FirstClass
class FirstClass extends StatelessWidget {
const FirstClass({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
color: Colors.amber,
child: const Center(
child: Text(
'First Class',
style: TextStyle(fontSize: 40),
)),
);
}
}
// SecondClass
class SecondClass extends StatelessWidget {
const SecondClass({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container();
}
}
The full source code in main.dart:
// main.dart
import 'package:flutter/material.dart';
// import FirstClass
import './test.dart' show FirstClass;
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the DEBUG banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('KindaCode.com')),
body: const FirstClass(),
);
}
}
Here’s the output:
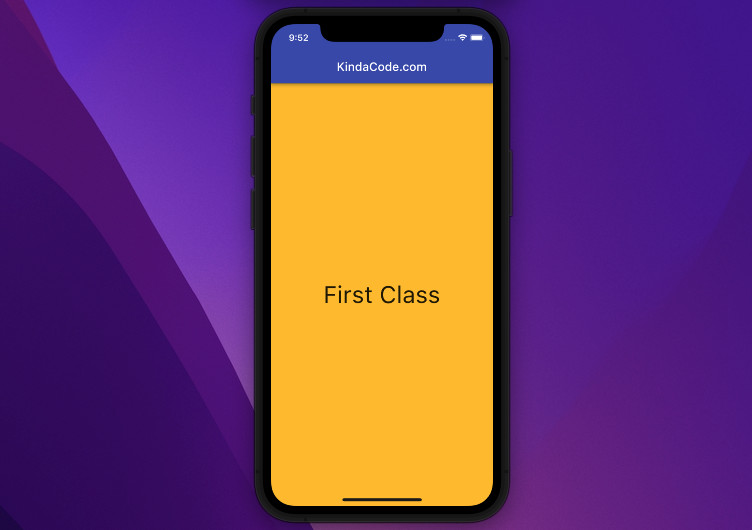
If you try to call the SecondClass, you will face an error that looks like this:
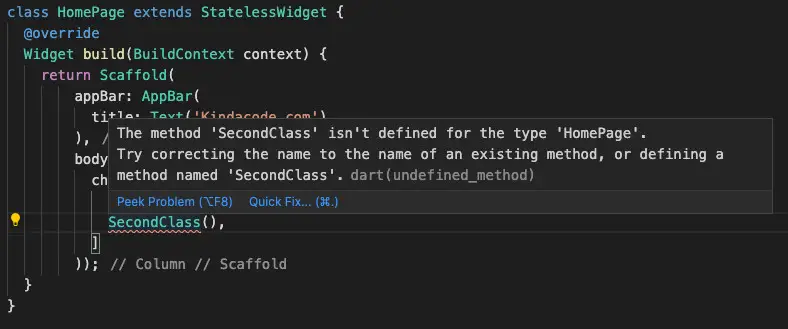
That’s obvious since we didn’t import it.
To expand your knowledge about Flutter, see:
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- Flutter: 2 Ways to Create an Onboarding Flow (Intro Slider)
- Flutter: Changing App Display Name for Android & iOS
- Dart: Convert Timestamp to DateTime and vice versa
- Using Stepper widget in Flutter: Tutorial & Example
- Flutter: How to place Text over an Image
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.