A few examples of using the InkWell widget in Flutter to create a rectangular area that responds to touch like a button.
Example 1: Simple Usage
Demo (the ripple effect you see in the GIF below won’t be as good as in real life):
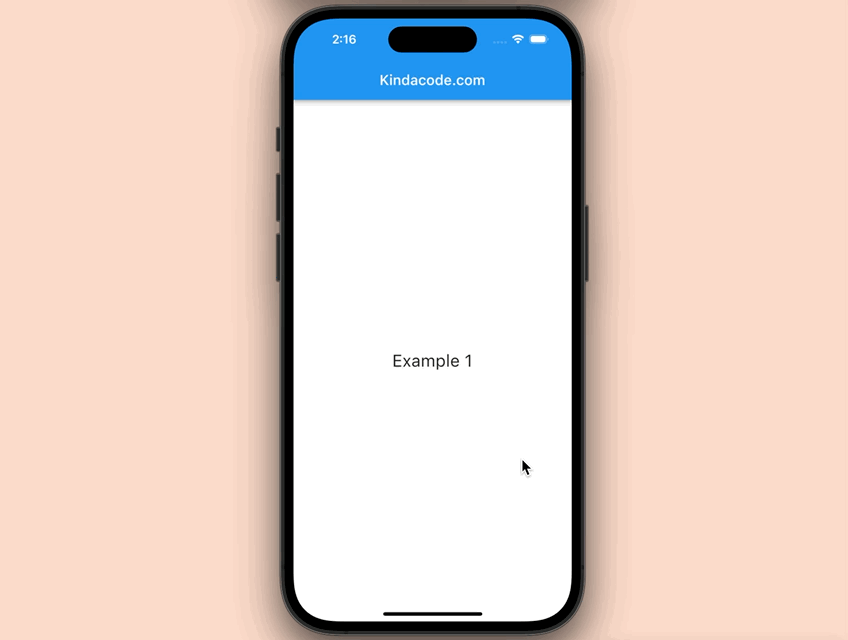
The code:
Scaffold(
appBar: AppBar(title: const Text('Kindacode.com')),
body: Center(
child: InkWell(
child: Container(
width: 200,
height: 80,
alignment: Alignment.center,
child: const Text(
'Example 1',
style: TextStyle(fontSize: 24),
),
),
onTap: () {
debugPrint('InkWell tapped');
// do something
}),
),
);
Example 2: Using InkWell with Material
By wrapping the InkWell widget inside a Material widget, we can set a background color. Here’s how it works (I’m really sorry that the GIF can’t convey the awesomeness of the animation):
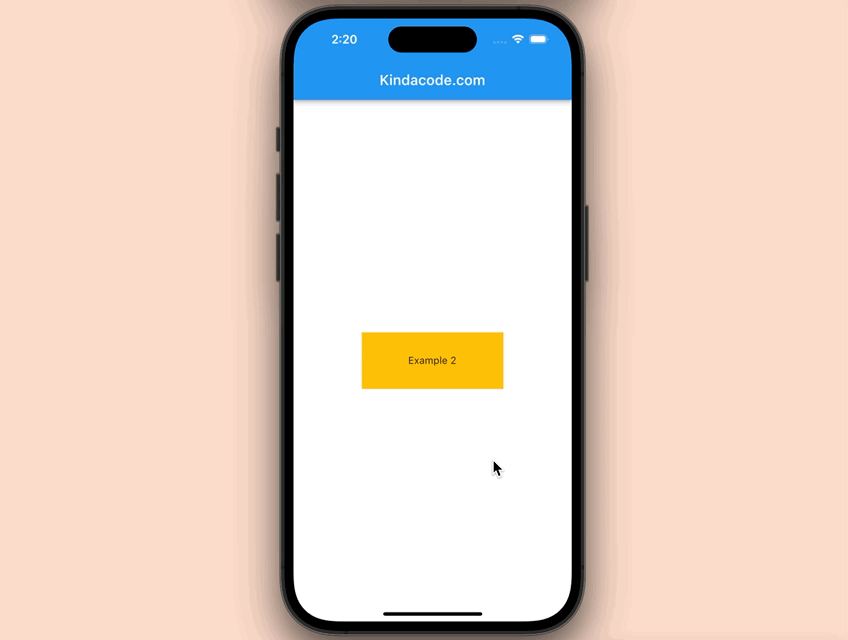
The code:
Scaffold(
appBar: AppBar(title: const Text('Kindacode.com')),
body: Center(
child: Material(
color: Colors.amber,
child: InkWell(
hoverColor: Colors.orange,
splashColor: Colors.red,
focusColor: Colors.yellow,
highlightColor: Colors.purple,
child: Container(
width: 200,
height: 80,
alignment: Alignment.center,
child: const Text('Example 2'),
),
onTap: () {
// Do somthing
},
),
),
),
);
See also: Ways to Create Typewriter Effects in Flutter
API
InkWell class constructor:
InkWell({
Key? key,
Widget? child,
GestureTapCallback? onTap,
GestureTapCallback? onDoubleTap,
GestureLongPressCallback? onLongPress,
GestureTapDownCallback? onTapDown,
GestureTapCancelCallback? onTapCancel,
ValueChanged<bool>? onHighlightChanged,
ValueChanged<bool>? onHover,
MouseCursor? mouseCursor,
Color? focusColor,
Color? hoverColor,
Color? highlightColor,
MaterialStateProperty<Color?>? overlayColor,
Color? splashColor,
InteractiveInkFeatureFactory? splashFactory,
double? radius,
BorderRadius? borderRadius,
ShapeBorder? customBorder,
bool? enableFeedback = true,
bool excludeFromSemantics = false,
FocusNode? focusNode,
bool canRequestFocus = true,
ValueChanged<bool>? onFocusChange,
bool autofocus = false
})
You can find more detailed information about each property in the official docs.
Final Words
We’ve walked through a few examples of implementing the InkWell widget. If you’d like to explore more new and exciting things in Flutter, take a look at the following articles:
- Flutter: Convert UTC Time to Local Time and Vice Versa
- Flutter: Making a Tic Tac Toe Game from Scratch
- Flutter & Hive Database: CRUD Example
- How to make Circular Buttons in Flutter
- Using BlockSemantics in Flutter: Tutorial & Example
- Flutter: Creating an Auto-Resize TextField
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.