CSV stands for Comma-Separated Values, is a file type widely used to store tabular data (numbers and text) in the plain text where each line will have the same number of fields. In this article, we will explore how to read content from a CSV file in Flutter and display that content on the screen.
Below is the CSV file used in the following example. Just download it before diving into the code:
https://www.kindacode.com/wp-content/uploads/2021/01/kindacode.csv
The content inside this file is simple (which only includes IDs, names, and ages of some fiction users) as below:
Id,Name,Age
1,John Doe,40
2,Kindacode,41
3,Voldermort,71
4,Joe Biden,80
5,Ryo Hanamura,35
Table of Contents
The Complete Example
Preview
This example has a floating button. When this button is pressed, the data from the CSV file will be loaded and displayed in a ListView:
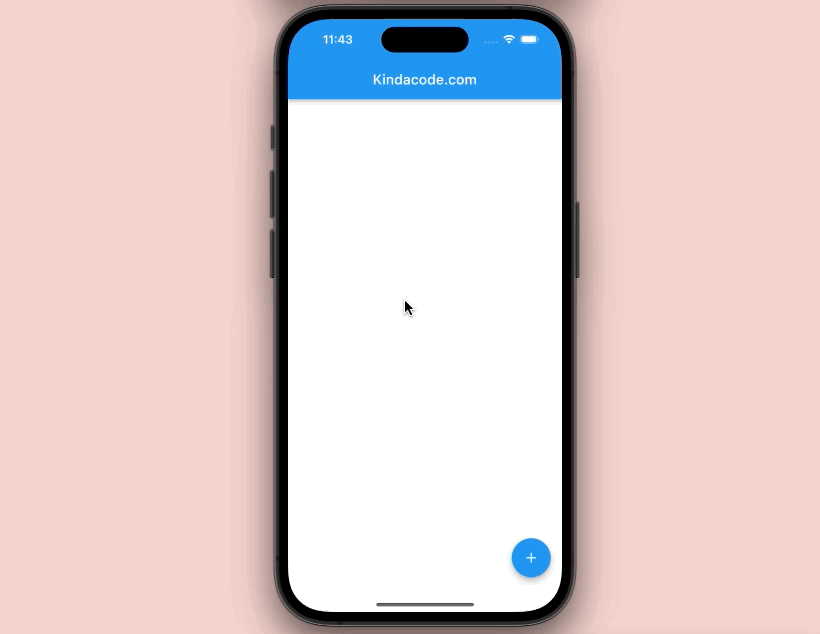
The steps
1. Copy the CSV file (the one you’ve seen at the beginning of this article) to the assets folder (create one if it doesn’t exist) in the root directory of your project. Don’t forget to declare this assets folder in the pubspec.yaml:
# The following section is specific to Flutter.
flutter:
assets:
- assets/
2. Install the csv package by executing the following command:
flutter pub add csv
Then run:
flutter pub get
3. Add the following to your main.dart:
import 'package:flutter/material.dart';
import 'package:flutter/services.dart' show rootBundle;
import 'package:csv/csv.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
List<List<dynamic>> _data = [];
// This function is triggered when the floating button is pressed
void _loadCSV() async {
final rawData = await rootBundle.loadString("assets/kindacode.csv");
List<List<dynamic>> listData = const CsvToListConverter().convert(rawData);
print(listData[0]);
setState(() {
_data = listData;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("Kindacode.com"),
),
// Display the contents from the CSV file
body: ListView.builder(
itemCount: _data.length,
itemBuilder: (_, index) {
return Card(
margin: const EdgeInsets.all(3),
color: index == 0 ? Colors.amber : Colors.white,
child: ListTile(
leading: Text(_data[index][0].toString()),
title: Text(_data[index][1]),
trailing: Text(_data[index][2].toString()),
),
);
},
),
floatingActionButton: FloatingActionButton(
onPressed: _loadCSV, child: const Icon(Icons.add)),
);
}
}
Now run your app, and you will see it works as shown above.
If you prefer to implement a Table instead of a ListView, check this guide: Working with Table in Flutter.
Conclusion
In this article, we went over a complete example of reading content from a CSV file and showing that content to the user. If you would like to learn more about Flutter, take a look at the following articles:
- Set an image Background for the entire screen
- 3 Ways to create Random Colors in Flutter
- Flutter Transform examples – Making fancy effects
- How to encode/decode JSON in Flutter
- How to read data from local JSON files in Flutter
- Flutter: How to Read and Write Text Files
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.