In Flutter, you can make a widget fill up the remaining space of a Row or Column by wrapping it inside an Expanded or a Flexible widget.
Table of Contents
Example
Preview:
In this example, the amber box will occupy all of the free space of its parent row. Other boxes have fixed sizes.
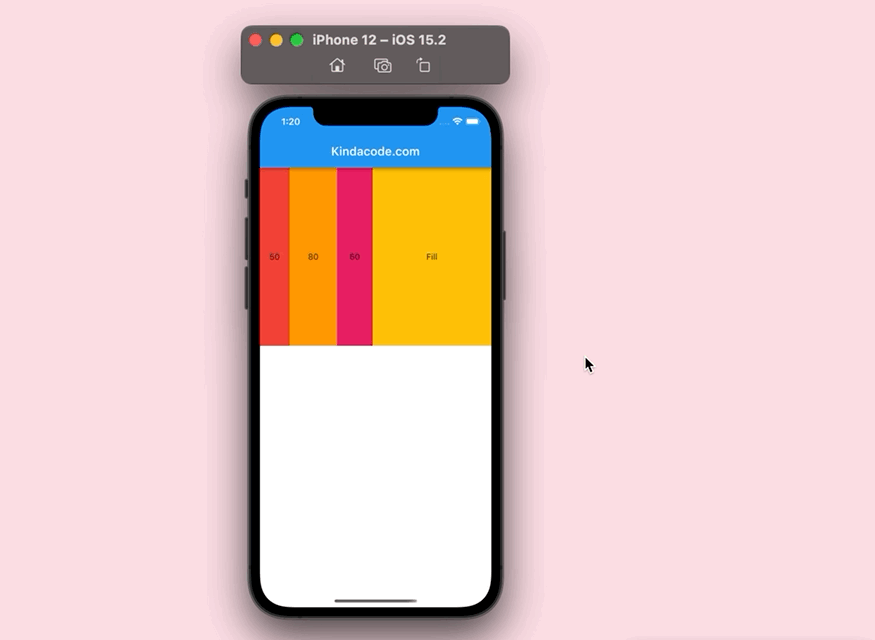
The Code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomePage());
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Row(
children: [
Container(
width: 50,
height: 300,
color: Colors.red,
child: const Center(
child: Text('50'),
),
),
Container(
width: 80,
height: 300,
color: Colors.orange,
child: const Center(
child: Text('80'),
),
),
Container(
width: 60,
height: 300,
color: Colors.pink,
child: const Center(
child: Text('60'),
),
),
// this will take all the remaning space
Flexible(
child: Container(
height: 300,
color: Colors.amber,
child: const Center(
child: Text('Fill'),
),
),
),
],
),
);
}
}
Another Example
Preview:
This example contains a column with some boxes inside it. The orange box will occupy the free space of its parent.
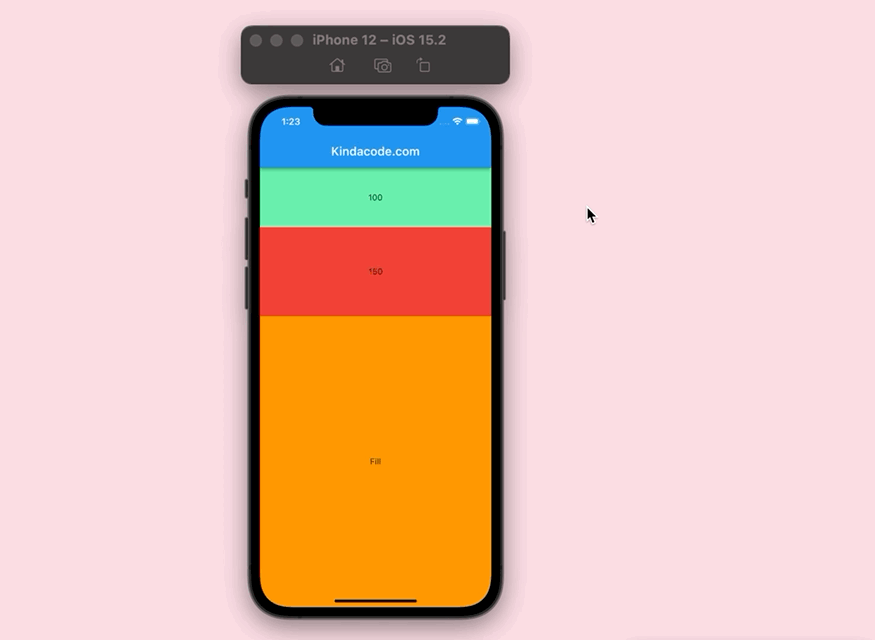
The Code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage());
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Column(
children: [
Container(
width: double.infinity,
height: 100,
color: Colors.greenAccent,
child: const Center(
child: Text('100'),
),
),
Container(
width: double.infinity,
height: 150,
color: Colors.red,
child: const Center(
child: Text('150'),
),
),
Flexible(
child: Container(
width: double.infinity,
color: Colors.orange,
child: const Center(
child: Text('Fill'),
),
),
),
],
),
);
}
}
What’s Next?
Continue learning more new and interesting things about Flutter by taking a look at the following articles:
- Flutter: Add a Search Field to an App Bar (2 Approaches)
- Using Provider for State Management in Flutter
- Flutter: ListView Pagination (Load More) example
- Flutter & SQLite: CRUD Example
- Flutter CupertinoSegmentedControl Example
- Flutter: ValueListenableBuilder Example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.