There may be times when you want to programmatically show or hide a widget in your Flutter applications. One of the best ways to achieve so is to use the Visibility widget.
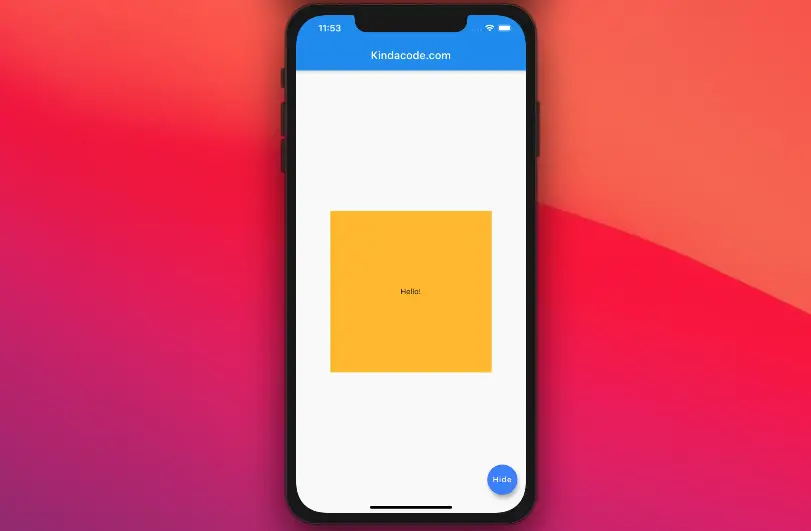
In this article, we are going to take a look at the Visibility widget and go over an example of implementing it.
Table of Contents
Example
This example creates a simple app that contains a floating button and an amber box. When users tap the button, the box will be visible or invisible based on a variable named _isShown.
App Preview
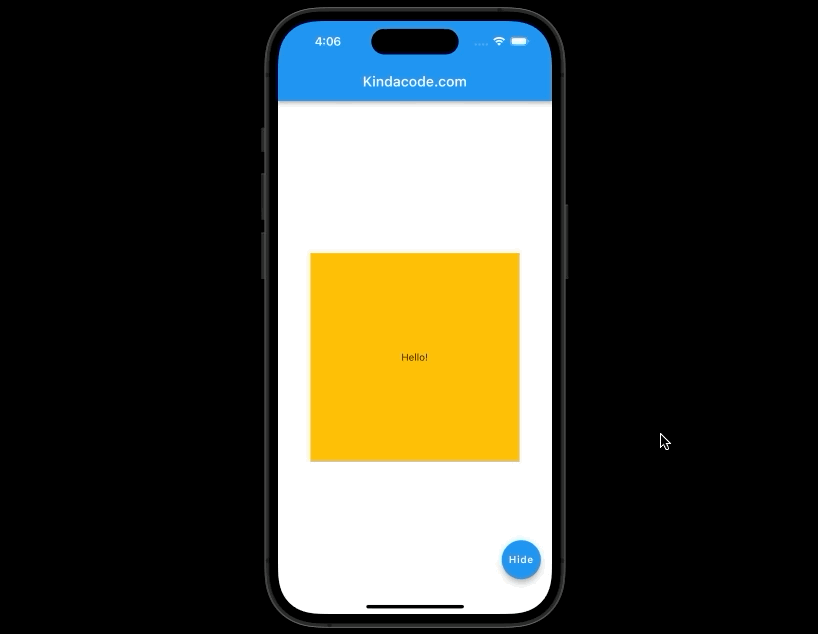
The full code
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(primarySwatch: Colors.blue),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
// The amber box is shown by default
bool _isShown = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Visibility(
visible: _isShown,
child: Container(
width: 300,
height: 300,
color: Colors.amber,
alignment: Alignment.center,
child: const Text('Hello!'),
),
),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_isShown = !_isShown;
});
},
child: Text(
_isShown ? 'Hide' : 'Show',
),
),
);
}
}
Options of Visibility
Below is the list of options supported by the Visibility widget:
Option | Required | Default | Type | Description |
---|---|---|---|---|
child | Yes | Widget | The child widget of the Visibility widget, that will be shown or hidden | |
visible | Optional | true | bool | Whether show or hide the child |
replacement | Optional | a zero-sized box | Widget | The widget that is displayed when the child is not visible |
maintainState | Optional | false | bool | Whether to maintain the child’s state if it becomes not visible |
maintainAnimation | Optional | false | bool | Whether to maintain the child’s animations if it becomes not visible |
maintainSize | Optional | false | bool | Whether to maintain space for where the widget would have been |
maintainSemantics | Optional | false | bool | Whether to maintain the semantics for the widget when it is hidden |
maintainInteractivity | Optional | false | bool | Whether to allow the widget to be interactive when hidden |
Conclusion
This article demonstrated how to programmatically hide or show a widget in an app using the Visibility widget. To explore more amazing and beautiful things in Flutter, take a look at the following articles:
- Using Chip widget in Flutter: Tutorial & Examples
- Using BlockSemantics in Flutter: Tutorial & Example
- Flutter: Making a Tic Tac Toe Game from Scratch
- Flutter: SliverGrid example
- Flutter StreamBuilder examples (null safety)
- Working with Timer and Timer.periodic in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.