This short article walks you through a few examples of creating circular images in Flutter. Without any further ado, let’s see some code.
Note: In the following examples, we will use some free images from Pixabay under the Pixabay License.
Using ClipRRect and Image widgets
The code:
Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Center(
child: ClipRRect(
borderRadius: BorderRadius.circular(800),
child: Image.network(
'https://www.kindacode.com/wp-content/uploads/2022/02/turtle.webp',
width: 400,
height: 400,
fit: BoxFit.cover,
),
),
),
);
Here’s the result on iOS and Android:
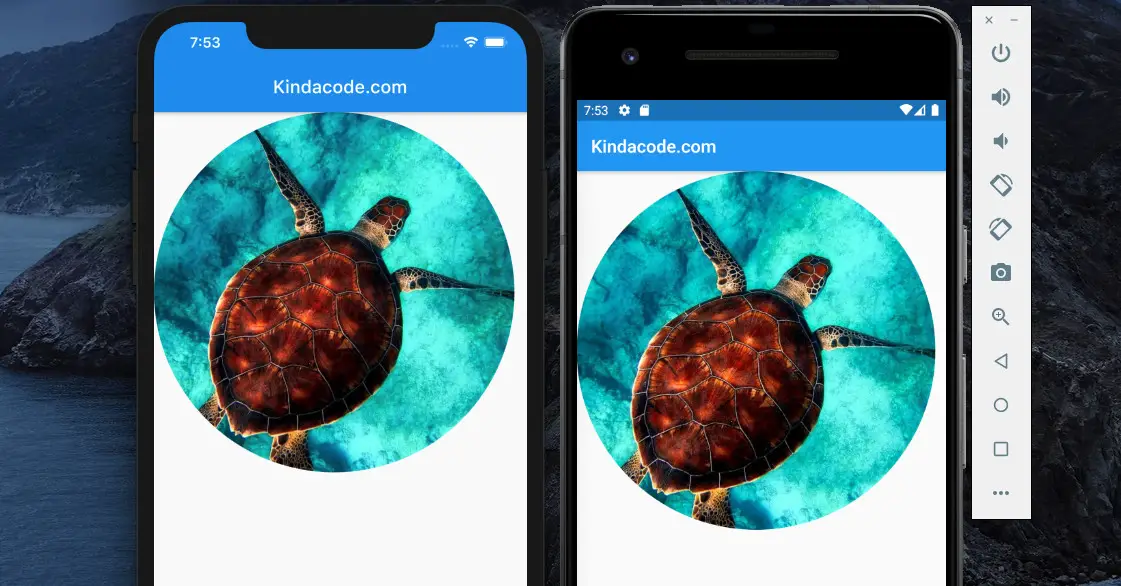
Using the CircleAvatar widget
The code:
Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: const Padding(
padding: EdgeInsets.all(10),
child: Center(
child: CircleAvatar(
radius: 300,
backgroundImage: NetworkImage(
'https://www.kindacode.com/wp-content/uploads/2022/02/horse.jpeg',
scale: 1),
)),
),
);
Screenshot:
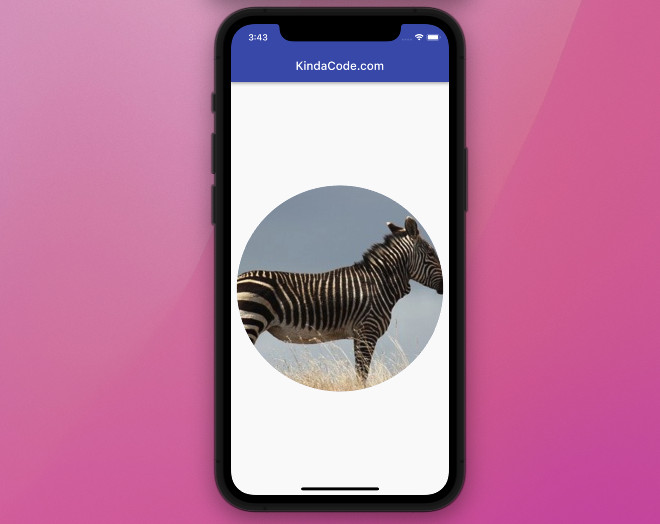
The two examples in this article are simple, but from here, you’re pretty good to go.
Further reading:
- Flutter image loading builder example
- Hero Widget in Flutter: Tutorial & Example
- Flutter: Caching Network Images for Big Performance gains
- Flutter: Reading Bytes from a Network Image
- Creating Masonry Layout in Flutter with Staggered Grid View
- Using Chip widget in Flutter: Tutorial & Examples
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.