The 2 following examples show you how to create rounded-corner cards and circle cards in Flutter by using the Card widget.
Rounded Card
Screenshot:
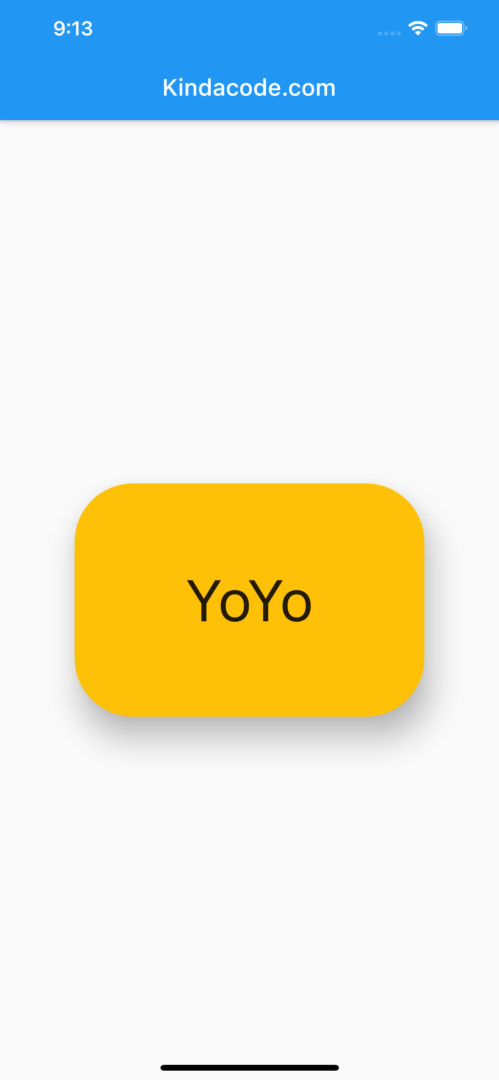
The code:
Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Card(
elevation: 20,
color: Colors.amber,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(50),
),
child: const SizedBox(
width: 300,
height: 200,
child:
Center(child: Text('YoYo', style: TextStyle(fontSize: 50))),
)),
));
Circle Card
A circle card actually is a specific rounded card.
Screenshot:
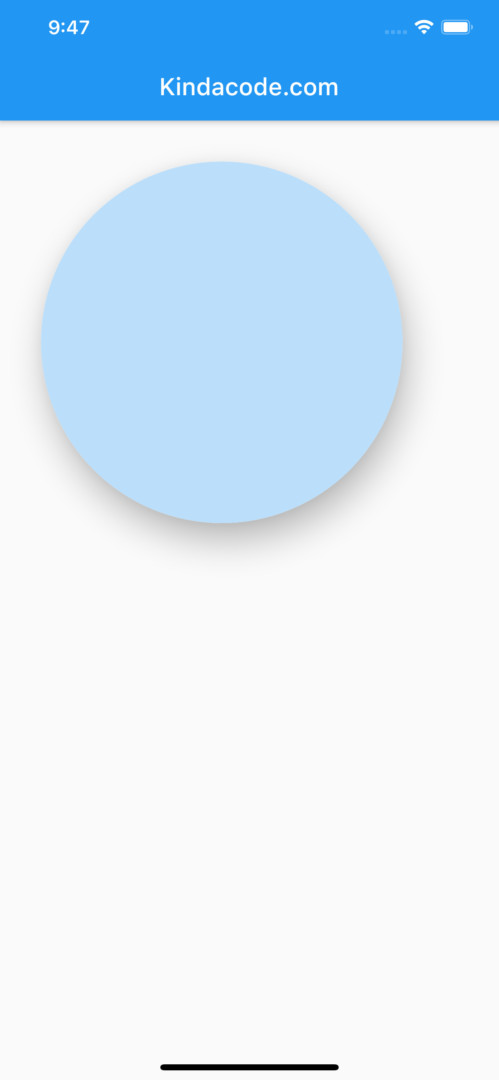
The code snippets for the circle Card
You can set the shape property to RoundedRectangleBorder() or CircleBorder() in order to create a circle Card.
If you use RoundedRectangleBorder, the key point here is to increase the border radius to a large number, at least half the width of the Card’s child. One other necessary thing is that the child needs to be a square.
Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Card(
elevation: 20,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(150),
),
child: Container(
width: 300,
height: 300,
decoration: BoxDecoration(
/*
The child of a round Card should be in round shape
if it has a background color
*/
shape: BoxShape.circle,
color: Colors.blue[100]))),
));
Using CircleBorder is much shorter and neater:
Card(
elevation: 20,
shape: const CircleBorder(),
color: Colors.blue[100],
child: const SizedBox(
width: 300,
height: 300,
)),
Full code in main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: "Kindacode.com",
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Padding(
padding: const EdgeInsets.all(30),
child: Card(
elevation: 20,
color: Colors.blue[100],
shape: const CircleBorder(),
child: const SizedBox(
width: 300,
height: 300,
)),
));
}
}
Hope these examples are helpful to you.
If you would like to learn more new and interesting things about Flutter, take a look at the following articles:
- Ways to Store Data Offline in Flutter
- Flutter: Global, Unique, Value, Object, and PageStorage Keys
- How to make an image carousel in Flutter
- Flutter SliverAppBar Example (with Explanations)
- Flutter: Check Internet Connection without any plugins
- Using Stack and IndexedStack in Flutter
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.