In this article, you will see an example of how to create a fullscreen gradient background for a Flutter application.
Table of Contents
Summary
In order to set a gradient background for the entire screen, just follow these steps:
- Wrap the Scaffold widget with a Container
- Set Scaffold’s backgroundColor to Colors.transparent
- Set a gradient background for the wrapper Container using BoxDecoration.
Example
Screenshots:
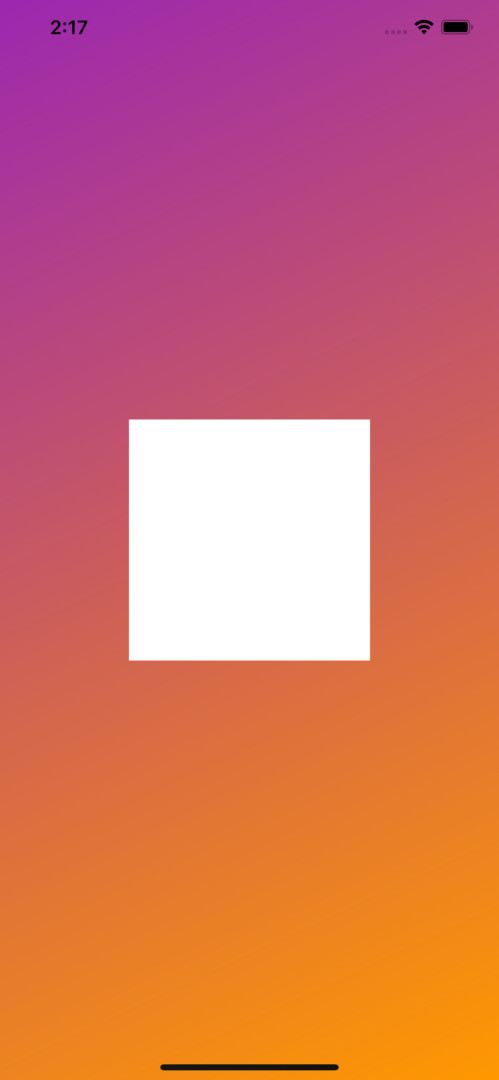
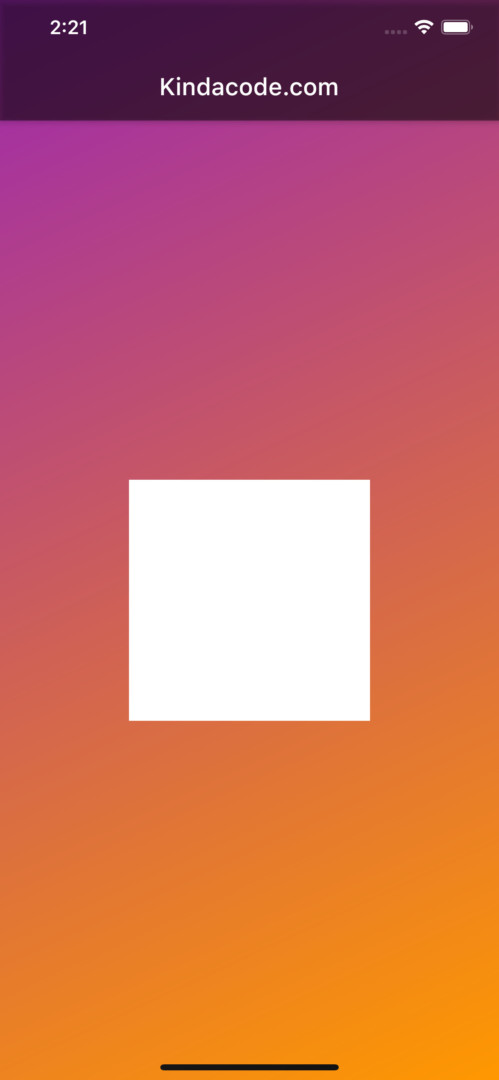
The code (no AppBar):
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
colors: [Colors.purple, Colors.orange])),
child: Scaffold(
// By defaut, Scaffold background is white
// Set its value to transparent
backgroundColor: Colors.transparent,
body: Center(
child: Container(
width: 200,
height: 200,
color: Colors.white,
),
)),
);
}
}
The code (with AppBar):
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
colors: [Colors.purple, Colors.orange])),
child: Scaffold(
// By defaut, Scaffold background is white
// Set its value to transparent
backgroundColor: Colors.transparent,
appBar: AppBar(
backgroundColor: Colors.black45,
title: const Text('Kindacode.com'),
),
body: Center(
child: Container(
width: 200,
height: 200,
color: Colors.white,
),
)),
);
}
}
Conclusion
In this article, you explored an example of how to set gradient background color for the whole screen in a Flutter app. If you’d like to learn more exciting stuff in Flutter and Dart, take a look at the following articles:
- Flutter Gradient Text Examples
- Flutter: Adding a Gradient Border to a Container (2 Examples)
- Flutter: Making a Tic Tac Toe Game from Scratch
- 2 Ways to Get a Random Item from a List in Dart (and Flutter)
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
- Flutter: SliverGrid example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.