The SizedOverflowBox widget in Flutter, as its name describes itself, is used to create a box with certain width and height but lets its child overflow. You can implement a SizedOverFlow box as follows:
SizedOverflowBox({
Key? key,
required Size size, // Size(double width, double height)
AlignmentGeometry alignment = Alignment.center,
Widget? child
})
Where:
- size: The size this widget should attempt to be
- alignment: Align the child widget
- child: The child widget
Example
This example displays 2 widgets. The parent widget is purple in color and the child widget is amber. Here’s the screenshot:
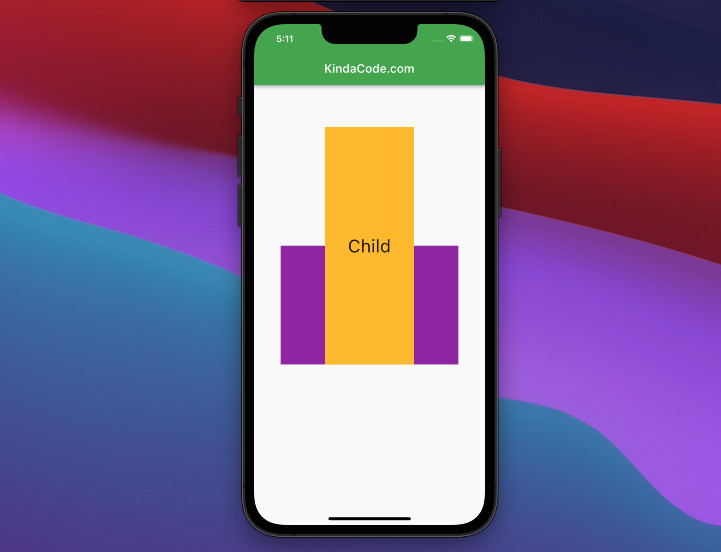
The code:
Center(
child: Container(
color: Colors.purple,
child: SizedOverflowBox(
size: const Size(300, 200),
alignment: Alignment.bottomCenter,
child: Container(
width: 150,
height: 400,
color: Colors.amber,
child: const Center(
child: Text(
'Child',
style: TextStyle(fontSize: 30),
),
),
),
),
),
),
Further reading:
- Flutter: Creating Strikethrough Text (Cross Out Text)
- Flutter: Firing multiple Futures at the same time with FutureGroup
- Flutter: Create a Password Strength Checker from Scratch
- Flutter StreamBuilder examples (null safety)
- Flutter and Firestore Database: CRUD example (null safety)
- 2 Ways to Create Flipping Card Animation in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.