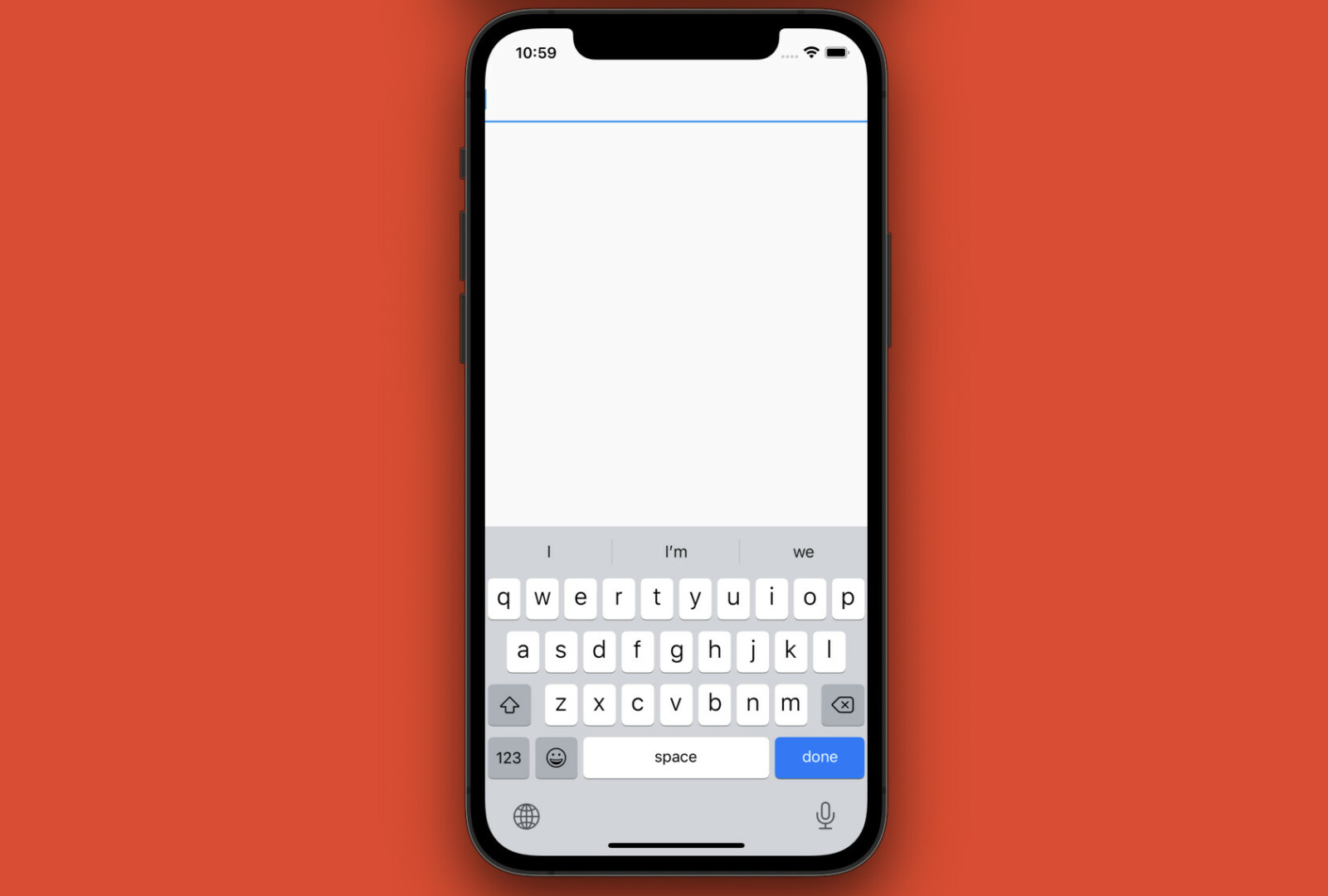
This is an easy-to-understand step-by-step guide on how to use stateful widgets in Flutter (beginner level).
Table of Contents
Prerequisites
Before getting started, you should have:
- Basic Flutter knowledge
- Flutter SDK, Visual Studio Code
- Android Studio or Xcode
The tiny app we’re going to make has nothing but a text field. When the user enters text into the text field, the entered text will be displayed on the screen immediately:
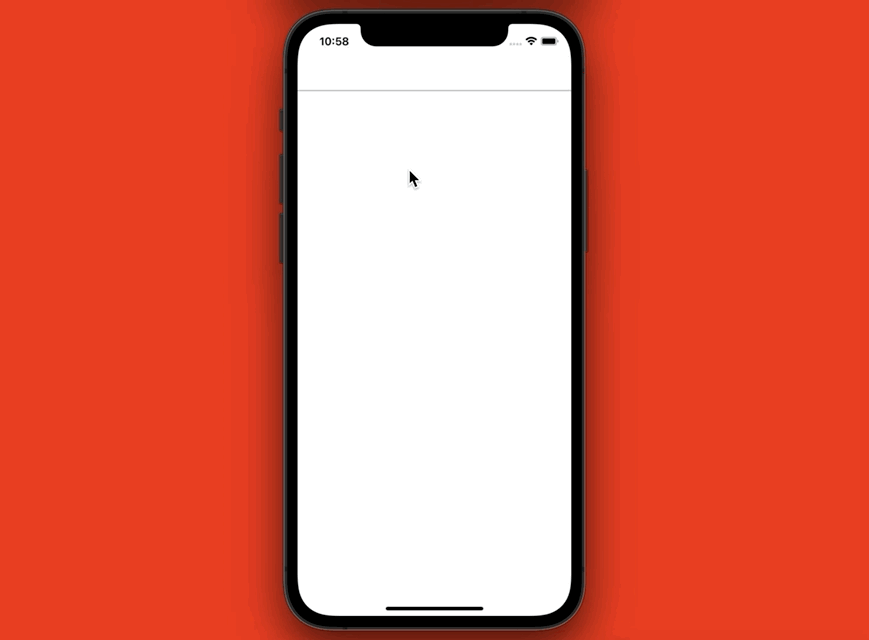
The Steps
1. Create a new Flutter project:
flutter create my_project
2. Delete everything in lib/main.dart then add the following:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.pink,
),
home: const HomeScreen(),
);
}
}
3. Type “st” and select “Flutter stateful widg…” from the suggestions:
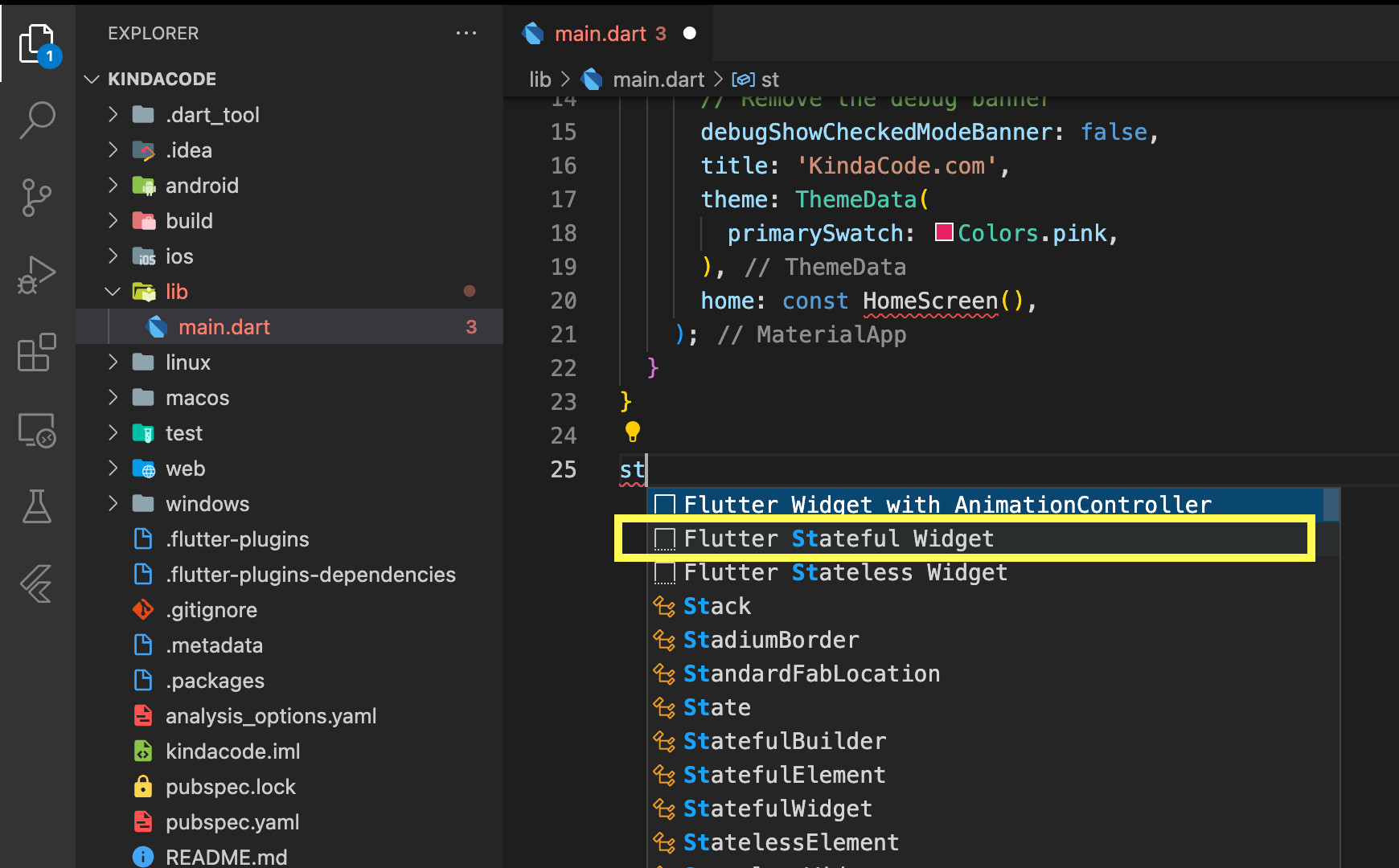
4. Type “HomeScreen” to name all the classes like so:
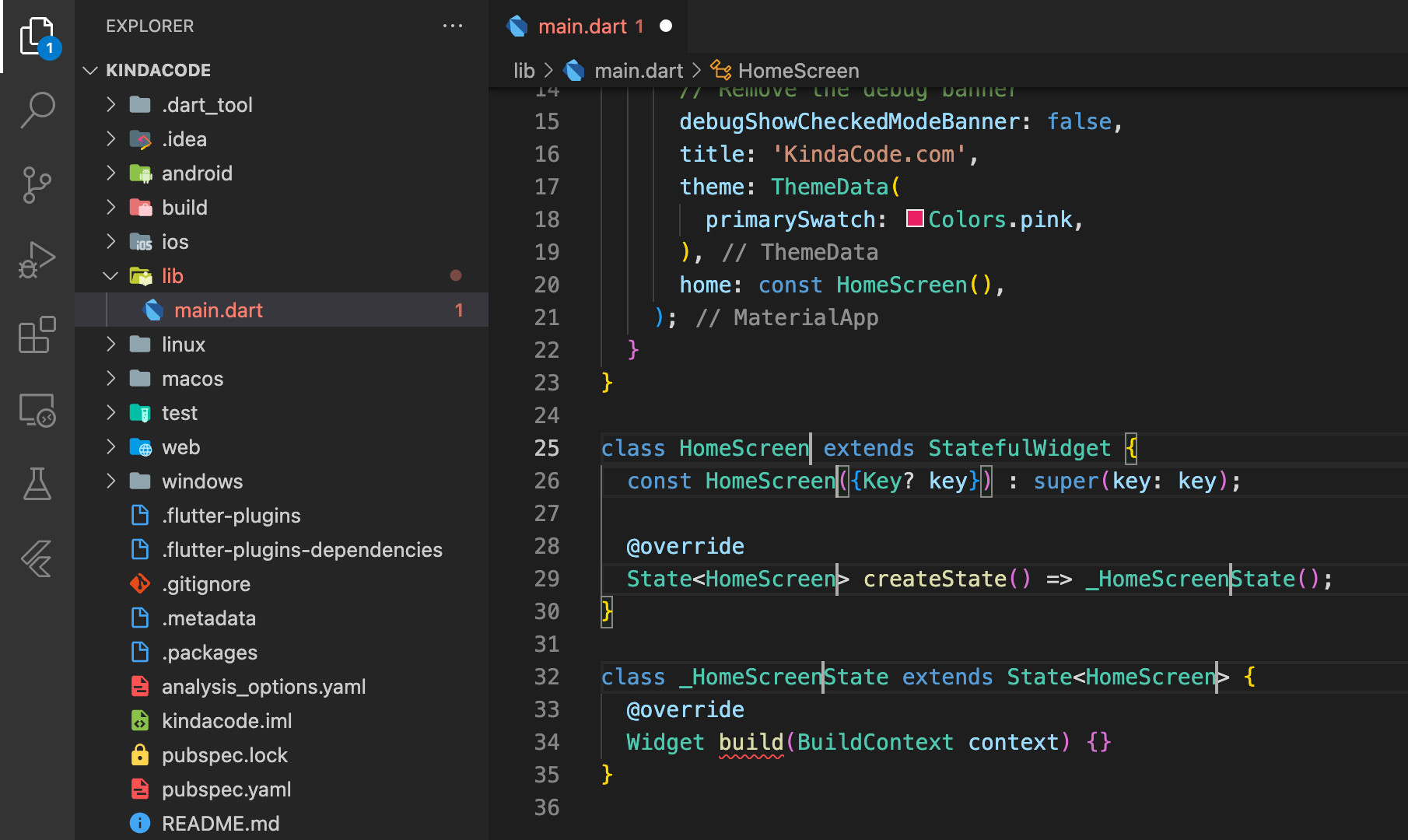
5. Edit the _HomeScreenState class like this:
class _HomeScreenState extends State<HomeScreen> {
// the text entered by the user
String enteredText = '';
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
home: Scaffold(
body: SafeArea(
child: Column(children: [
// The TextField that lets the user type something in
TextField(
onChanged: (value) {
setState(() {
enteredText = value;
});
},
),
// Adding some vertical space
const SizedBox(height: 30),
// Display the text entered by the user
Text(
enteredText,
style: const TextStyle(fontSize: 30),
),
]),
),
),
);
}
}
The complete code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.pink,
),
home: const HomeScreen(),
);
}
}
class HomeScreen extends StatefulWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
// the text entered by the user
String enteredText = '';
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
home: Scaffold(
body: SafeArea(
child: Column(children: [
// The TextField that lets the user type something in
TextField(
onChanged: (value) {
setState(() {
enteredText = value;
});
},
),
// Adding some vertical space
const SizedBox(height: 30),
// Display the text entered by the user
Text(
enteredText,
style: const TextStyle(fontSize: 30),
),
]),
),
),
);
}
}
Conclusion
Using stateful widgets is the easiest way to manage states in a single-screen or non-complex Flutter app. Once you feel comfortable with it, you can explore more advanced state management approaches by taking a look at the following:
- Most Popular Packages for State Management in Flutter
- Using Provider for State Management in Flutter
- Using GetX (Get) for State Management in Flutter
- Flutter: ValueListenableBuilder Example
- Flutter StatefulBuilder example
If you’d like to learn more about other things in Flutter, take a look at these articles:
- How to Subtract Two Dates in Flutter & Dart
- Flutter: Creating Transparent/Translucent App Bars
- Flutter: Firebase Remote Config example
- Flutter and Firestore Database: CRUD example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.