This article walks you through 4 techniques to vertically center a child widget inside a Container in Flutter.
Using the alignment property
Set the alignment property to Alignment.center, Alignment.centerRight, or Alignment.centerLeft.
Example
Container(
width: double.infinity,
height: 500,
color: Colors.amber,
alignment: Alignment.center,
child: Container(
width: 200,
height: 200,
color: Colors.red,
),
),
Output:
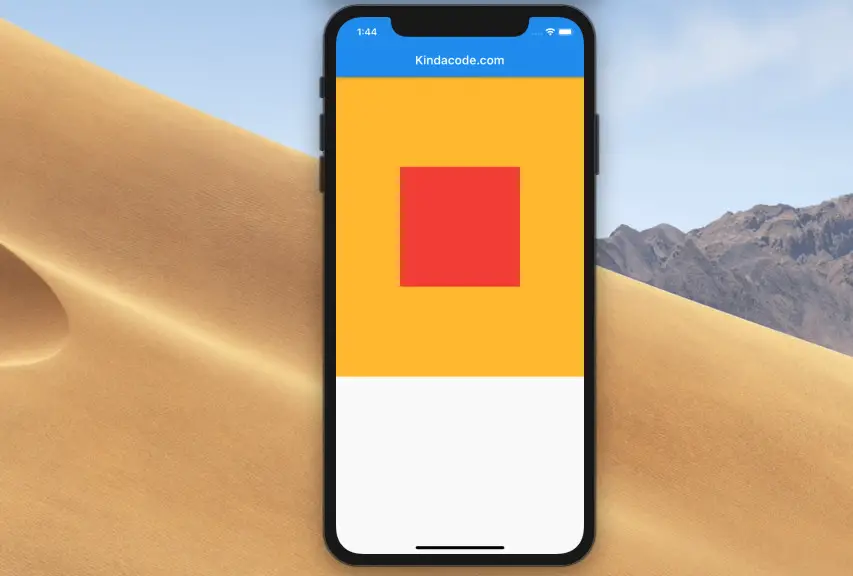
Using a Center widget
The Center widget is used to center its child within itself (both vertically and horizontally).
Example:
Container(
width: double.infinity,
height: 500,
color: Colors.amber,
child: Center(
child: Container(
width: 200,
height: 200,
color: Colors.purple,
),
),
),
Output:
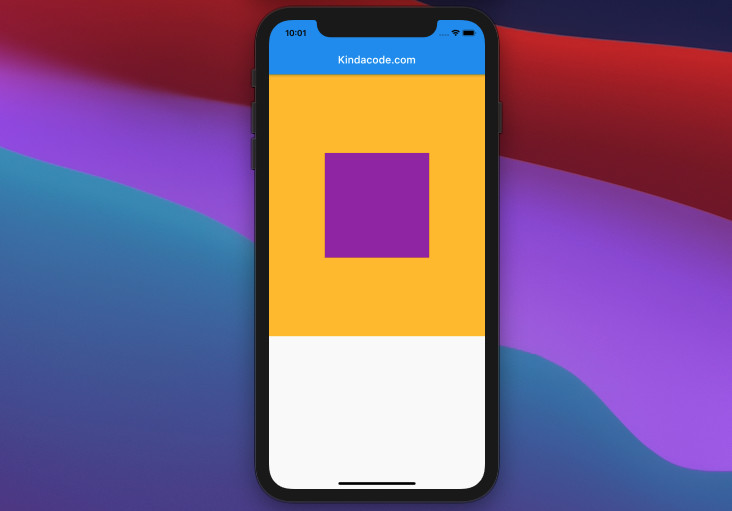
Adding a Column with mainAxisAlignment
In Flutter, to vertically center a child widget inside a Container, you can wrap the child widget with a Column and add mainAxisAlignment: MainAxisAlignment.center to it.
Example (with full code)
Create a new Flutter project and replace all the default code in ./lib/main.dart file with the following:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Flutter Example',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Example'),
),
body: Container(
height: 600,
// set the width of this Container to 100% screen width
width: double.infinity,
decoration: const BoxDecoration(color: Colors.yellow),
child: Column(
// Vertically center the widget inside the column
mainAxisAlignment: MainAxisAlignment.center,
children: [
Container(
height: 100,
width: 100,
decoration: const BoxDecoration(color: Colors.purple),
),
],
),
));
}
}
Output:
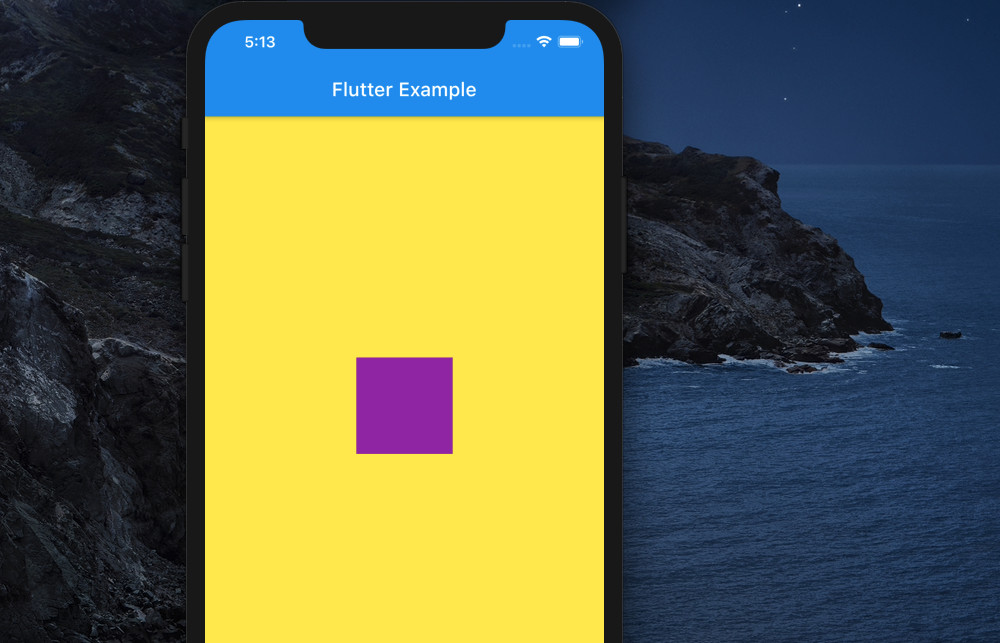
Using an Align widget
Wrap the child widget with an Align widget and set the alignment argument to Alignment.center, Alignment.centerLeft, or Alignment.centerRight.
The complete example:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Container(
color: Colors.green,
width: double.infinity,
height: 500,
child: Align(
alignment: Alignment.center,
child: Container(
width: MediaQuery.of(context).size.width * 0.9,
height: 100,
color: Colors.amber,
),
),
),
);
}
}
Screenshot:
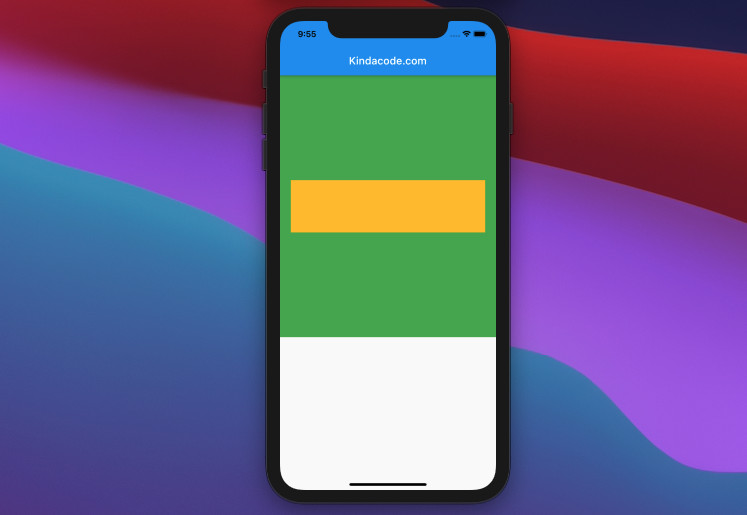
Wrapping Up
We’ve gone over some different ways for vertically centering a widget inside a Container. Knowing more than one way to solve a problem can help you gain more insight and flexibility in future situations.
Flutter’s awesome, and there’re many things to learn about it. Take a look at the following articles to explore more lovely stuff:
- Flutter and Firestore Database: CRUD example
- Working with Time Picker in Flutter
- Working with ReorderableListView in Flutter
- Flutter: Adding a Gradient Border to a Container (2 Examples)
- Flutter: Container border examples
- Working with Cupertino Date Picker in Flutter
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.