This short and straight-to-the-point article shows you how to set a base path for a React app created with create-react-app and using React Router.
Table of Contents
The Prefatory Matter
There might be situations where you want to run your React app with a base path (a URL prefix for all routes), such as your app is served from a subdirectory on your server, or you deploy multiple apps under a single domain.
These are some common real-world use cases:
https://www.kindacode.com/blogs/
https://www.kindacode.com/forums/
https://www.kindacode.com/dashboard/
https://www.kindacode.com/shop/
The Solution
To configure the base URL, set the basename prop of the <BrowserRouter> component (imported from react-router-dom) to a string, like so:
import { BrowserRouter, Routes, Route } from 'react-router-dom'
function App() {
return (
<BrowserRouter basename='/your-subdirectory'>
{/* ... */}
</BrowserRouter>
);
}
basename will be automatically added to every route of your app.
Before building your project for production, open the package.json file and add this line:
"homepage": "/your-subdirectory",
Here’s mine:
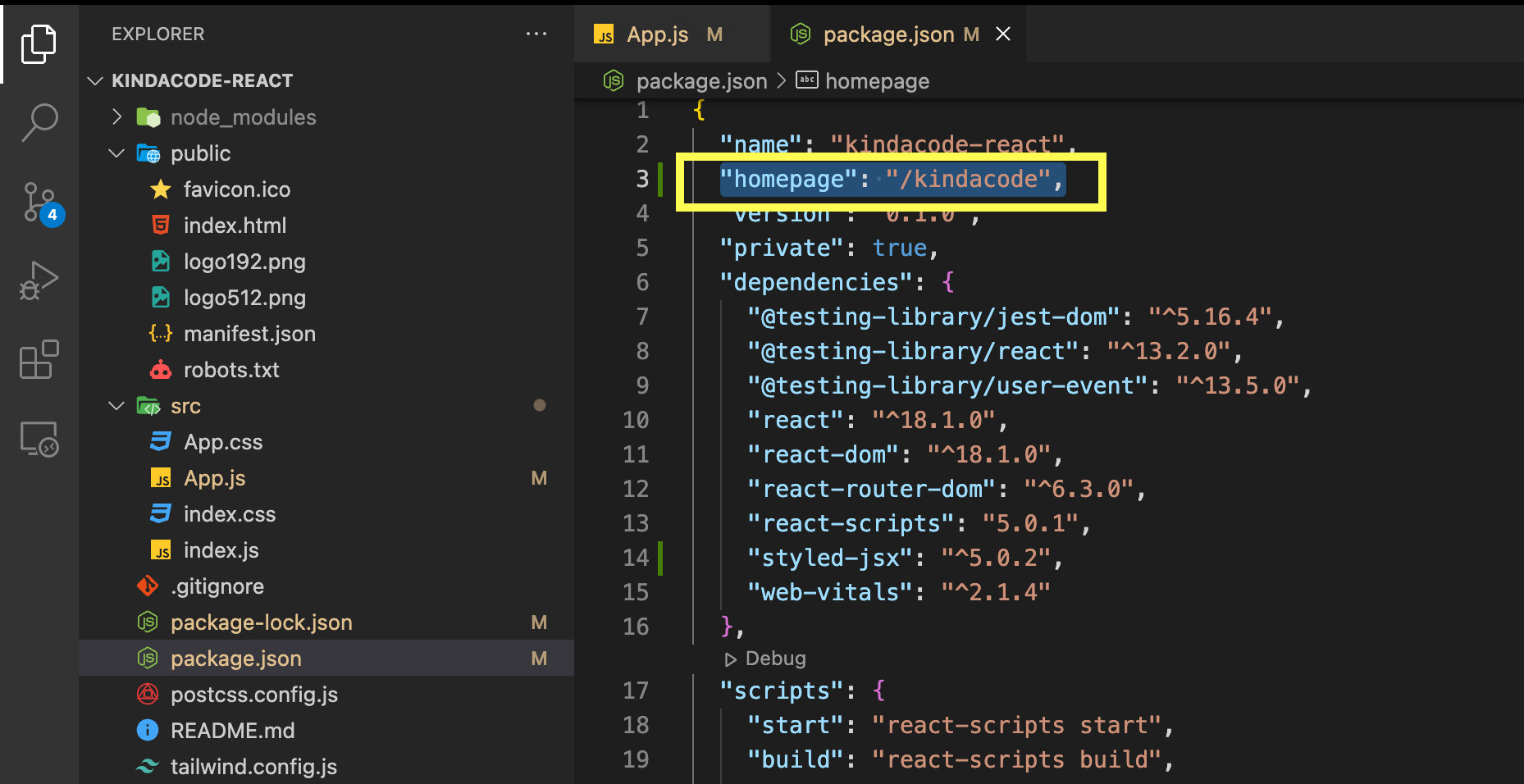
This will make sure that all the asset paths are relative to the index.html file when you deploy your build in the your-subdirectory folder (in my case, it is kindacode).
Example
This example uses React Router v6 (the latest one). The tiny app we’re going to make has two routes:
- /: Home
- /sample: SamplePage
The base path is /kindacode. Here’s how it works:
And here is the complete source code that produces our example:
// src/App.js
import { BrowserRouter, Routes, Route, Link } from 'react-router-dom'
function App() {
return (
<BrowserRouter basename='/kindacode'>
<Routes>
<Route path='/' element={<HomePage />} />
<Route path='/sample' element={<SamplePage />} />
</Routes>
</BrowserRouter>
);
}
// Home page
const HomePage = () => {
return <div style={{padding: 30}}>
<h1>Home Page</h1>
<p><Link to="/sample">Go to Sample page</Link></p>
</div>
}
// Sample Page
const SamplePage = () => {
return <div style={{padding: 30}}>
<h1>Sample Page</h1>
</div>
}
export default App;
Conclusion
You’ve learned how to change the base path for a React app for both development and production. If you’d like to explore more new and exciting about modern React, take a look at the following articles:
- React + TypeScript: Making a Reading Progress Indicator
- React + TypeScript: Making a Custom Context Menu
- Using Radio Buttons in React
- React: Check if user device is in dark mode/light mode
- React: Get the Position (X & Y) of an Element
- React + TypeScript: Making a Reading Progress Indicator
You can also check our React category page and React Native category page for the latest tutorials and examples.