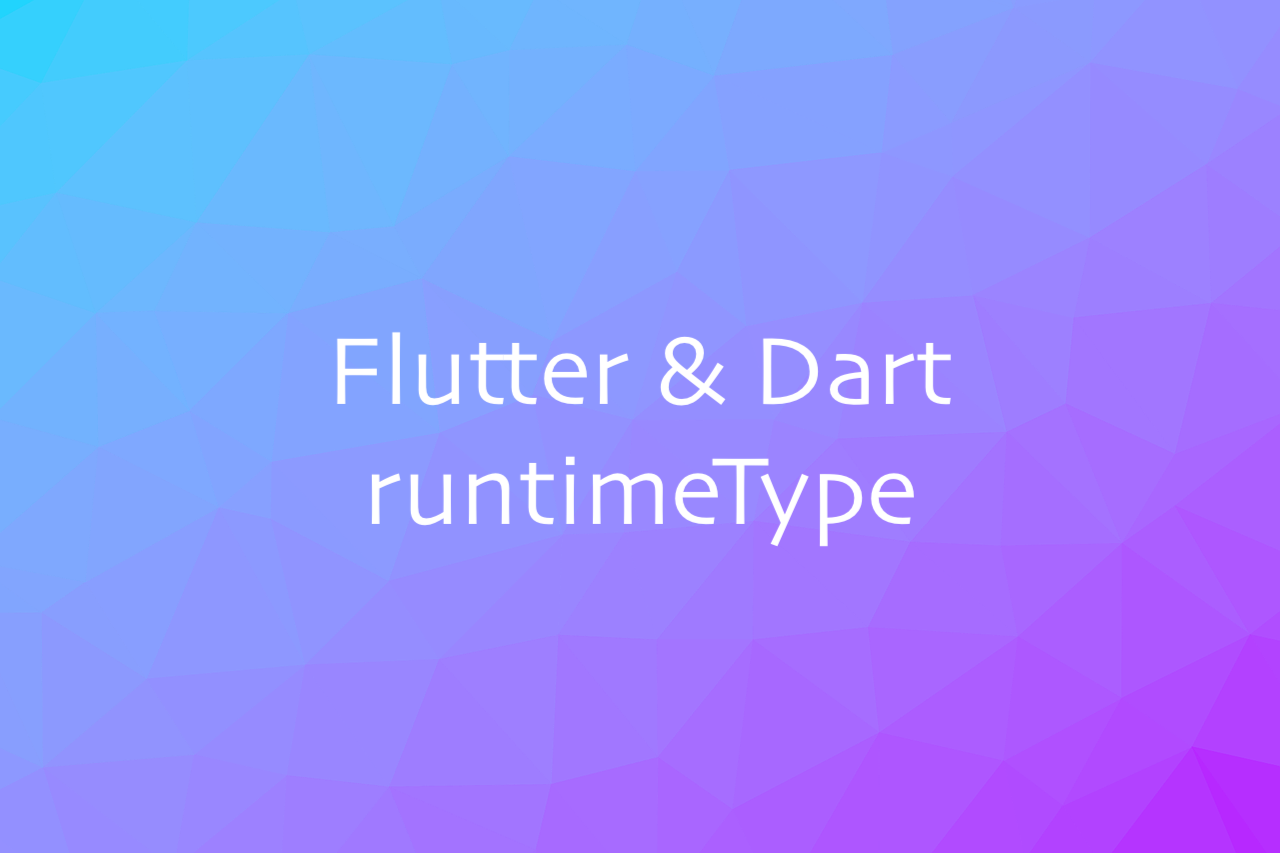
To check the type of a variable in Flutter and Dart, you can use the runtimeType property.
Example
The code:
void main(){
var a = 'Apple';
var b = 100;
var c = [1, 2, 3, 4, 5];
var d = {
"name": "John Doe",
"age" : 40
};
var e = 1.14;
print(a.runtimeType);
print(b.runtimeType);
print(c.runtimeType);
print(d.runtimeType);
print(e.runtimeType);
}
Output:
String
int
List<int>
_InternalLinkedHashMap<String, Object>
double
If you want to avoid warnings (just warnings, not errors) that come with the print() function, you can use do a check with kDebugmode like this:
import 'package:flutter/foundation.dart';
void main() {
var a = 'Apple';
var b = 100;
var c = [1, 2, 3, 4, 5];
var d = {"name": "John Doe", "age": 40};
var e = 1.14;
if (kDebugMode) {
print(a.runtimeType);
print(b.runtimeType);
print(c.runtimeType);
print(d.runtimeType);
print(e.runtimeType);
}
}
Further reading:
- Understanding Typedefs (Type Aliases) in Dart and Flutter
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- Flutter: Convert UTC Time to Local Time and Vice Versa
- Create a Custom NumPad (Number Keyboard) in Flutter
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
- Flutter: 2 Ways to Make a Dark/Light Mode Toggle
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.